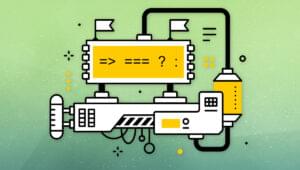
We explain what functional programming is, with five examples of how you can make your JavaScript more functional in style.
We explain what functional programming is, with five examples of how you can make your JavaScript more functional in style.
Learn how to recognize, write and use Python lambda functions — a handy option for one-liner functions, IIFEs, and functional programming.
In order to write good code, you need to have a strong understanding of the basics. This guide provides ten helpful techniques.
Higher-order functions can take other functions as arguments or return a function as a result. Learn how to use them and why they're useful.
Learn what higher-order functions are, how they work, why you might need them, and what they look like in PHP, JavaScript and Python.
As a programmer, you want to write elegant, maintainable, scalable, predictable code. The principles of functional programming can help.
Read Grab Our Free Printable Functional JavaScript Cheat Sheet and learn JavaScript with SitePoint. Our web development and design tutorials, courses, and books will teach you HTML, CSS, JavaScript, PHP, Python, and more.
Marcello Duarte explains how we can use functional programming to build a JSON parser from scratch in PHP! Join us in exploring advanced PHP!
Abstraction is the key to tenable code bases. Where OOP eyes abstraction with suspicion, functional programming pushes it as far as possible.
Marcello Duarte of Inviqa shares some functional programming insight with us by teaching us how to build Parser combinations with Phunkie! Hardcore!
Functional programming solutions for FizzBuzz using Vavr (formerly Javaslang) and common FP features like streams, pattern matching, and combinator.
Referential transparency is a tool to help programmers reason about their programs and make them safer, and easier to test and to maintain.
Java is known as a mostly strict language. It's useful, however, to implement a 'Lazy' type for lazy initialization, deferred computations, etc.
M. David Green uses filtering to limit a data set & chaining to combine the results with map/reduce. The result—clean code that performs complex operations.
With the aid of seven comprehensive demos, Mark Brown introduces you to Choo — a fun new framework for building single page apps in a functional manner.
Java's Optional, unlike may-or-may-not-contain-a-value types in other languages, is no well-behaving monad. And this matters to everyday developers like us!
The Combinator Pattern, well known in FP, combines primitives into complex structures. Gregor Trefs explores an implementation in Java 8.
M. David Green demonstrates how to implement function composition in JavaScript, a technique which lends itself to writing cleaner and more succinct code.
Florian Rappl introduces Ramda.js, a powerful toolbox designed with functional programming in mind, and talks about the concepts on which its based.
Ashraff explains how to create streams and then transform them using three widely used higher-order methods named map, filter and reduce.
Younes explains the concept of transducers and demonstrates their use in PHP with Michael Dowling's library
Using the Mori library, Dan Prince demonstrates how to create and work with immutable data structures, making your code simpler and easier to reason about.
Christopher Pitt dives deeper into PHP macros, using them to add some functional programming to PHP!
M. David Green demonstrates how using the functional programming techniques of mapping & reducing can lead to cleaner code which is easy to read & maintain.
Sebastian Porto takes a look at functional reactive programming with Elm, an up-and-coming programming language that compiles to JavaScript.
Curious about functional reactive programming? Florian Rappl explains the concepts, working through several examples using the RxJS library.
Mark Brown shows how to to write simpler programs with fewer bugs by using techniques from functional programming in JavaScript
M. David Green demonstrates the concept of currying — a useful technique, to partially evaluate functions and make your functional JavaScript more readable.
M. David Green demonstrates the powerful, but dizzying concept of recursion by refactoring normal for and while loops to use functions that call themselves.
In the second of a two-part series on Functional Programming, learn about Pure and Composable functions, the benefits and tactics therein.