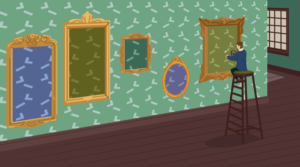
Learn about decorators in JavaScript: what they are, how they work, what they're useful for, their pros and cons, and how to use them.
Learn about decorators in JavaScript: what they are, how they work, what they're useful for, their pros and cons, and how to use them.
Decorators let us craft a function that tweaks the behavior of a class and its methods. They're now ready for the prime time in TypeScript 5.
We introduce nvm, a handy command-line tool that allows you to install multiple versions of Node.js and switch between them with ease.
Learn the different ways you can use the spread operator in JavaScript, and the main difference between the spread and rest operators.
In this quick JavaScript regex matching guide, you'll learn how to test if a string matches a regular expression using the test() method.
Learn how to transform the character case of a string — to uppercase, lowercase, and title case — using native JavaScript methods.
Learn how to split a string into substrings in JavaScript with both the substring() and split() methods, and how to choose which one to use.
Learn how to convert numbers to ordinals in JavaScript. Getting the ordinal of a number allows you to display it in a human-readable format.
Learn three simple ways to convert a string into a number in JavaScript, with simple-to-follow examples and tips on which method to use.
Learn four simple ways to convert a number into a string in JavaScript, with simple-to-follow examples and tips on which method to use.
Data fetched from remote servers is often in JSON format. Learn how to use JavaScript to parse the server's response.
Learn date-fns, a functional date library, and a lightweight alternative to Moment.js. With 130+ functions for all occasions, it's like Lodash for dates.
Learn how to parse query string parameters and get their values in JavaScript. Use the results for tracking referrals, autocomplete, and more
Sort an array of objects in JavaScript dynamically. Learn how to use Array.prototype.sort() and a custom compare function, and avoid the need for a library.
Jeff Mott guides you through a step-by-step approach to JavaScript object creation — from object literals to factory functions and ES6 classes.
Moritz Kröger shows to use ES6 default parameters and property shorthands to help speed up development and write cleaner, clearer and more organized code.
Camilo Reyes looks at the observer pattern — a handy pattern to use for keeping parts of a page in sync in response to events and the data they provide.
Take a step along the path to library-free development & join Giulio Mainardi for look at six native DOM manipulation methods that were inspired by jQuery.
Giulio Mainardi looks at event propagation in JavaScript. He examines event bubbling & capture and shows how they fit into the basic JavaScript event flow.
Albert Senghor shows how to make a sticky navigation menu, similar to the one you find on Medium, that drops back into view as your scroll up the page.
Do you use a WordPress blog, and wish to automate your social media posting of new blog entries? Learn how to use JetPack to do so!
Naveen Karippai takes a close look at how JavaScript references work, how they differ from primitive values, and shows how to avoid some common gotchas.
George Martsoukos shows how to build a simple Flexbox and jQuery plugin which sorts elements based on the values of their custom data attributes.
Ayush Gupta shows how to quickly create mock REST APIs to develop and test your client-side apps, with advanced features like filtering and pagination.
Julian Motz takes a look at jQuery's document.ready() method and shows how it can be replaced with vanilla JS, and is often not needed at all!
Yaphi Berhanu demonstrates two different methods for adding and removing a CSS class to DOM elements with vanilla JavaScript. No jQuery required!
Julian Motz examines how variables are declared in JavaScript, and introduces the three different types of declaration and their uses.
Dan Prince looks at factory functions in JavaScript, examining the different types, their use cases & how they allow us to separate data from computations.
Mark Brown demonstrates how to make a simple game loop in JavaScript, paving the way for you to start making your own browser based games and animations.
In this quick tip Bruno Mota demonstrates creates a reusable video component with React, using react-hot-boilerplate to jump start his dev environment.