In this tutorial, you’ll learn how to convert numbers to ordinals in JavaScript. Getting the ordinal of a number allows you to display it in a human-readable format.
What Are Ordinals?
Ordinals define numbers as being part of an order or sequence. The words “first”, “second”, and “third” are all examples of ordinals. When using numbers to display chart results, days of the month, or a ranking, you’ll often need to use ordinals.
Numbers can be used to display many different types of data and results. When numbers are presented to users, they often need be presented in a format that’s more readable — such as adding ordinal suffix (“June 12th” rather than “June 12”, for example).
Ordinal Suffix Rules in English
Let’s take a look at how ordinals are used in the English language. English ordinals follow a predictable, if not beautifully simple, set of rules:
“st” is appended to 1 and numbers that are one greater than a multiple of ten, except for 11 and numbers that are 11 greater than a multiple of 100. For example, 1st, 21st, 31st, etc. … but 11th, 111th, etc.
“nd” is appended to 2 and numbers that are two greater than a multiple of ten, except for 12 and numbers that are 12 greater than a multiple of 100. For example, 2nd, 22nd, 32nd, etc. … but 12th, 112th, etc.
“rd” is appended to 3 and numbers that are three greater than a multiple of ten, except for 13 and numbers that are 13 greater than a multiple of 100. For example, 3rd, 23rd, 33rd, etc. … but 13th, 113th, etc.
“th” is appended to everything else. For example, 24th.
How to Get the Ordinal of a Number
To get the ordinal of a number, you can use the following function:
function getOrdinal(n) {
let ord = 'th';
if (n % 10 == 1 && n % 100 != 11)
{
ord = 'st';
}
else if (n % 10 == 2 && n % 100 != 12)
{
ord = 'nd';
}
else if (n % 10 == 3 && n % 100 != 13)
{
ord = 'rd';
}
return ord;
}
The function getOrdinal
accepts an argument that is a number and returns the ordinal of that number. Since most ordinals end in “th”, the default value of ord
is set to th
. Then, you test the number on different conditions and change the ordinal if necessary.
You’ll notice that in each of the conditions the remainder (%) operator is used. This operator returns the leftover value of dividing the left operand by the right operand. For example, 112 % 100
returns 12
.
To test if the number should have the ordinal st
, you check if n
is one greater than a multiple of ten (n % 10 == 1
, which includes 1 itself), but isn’t 11 greater than a multiple of 100 (n % 100 != 11
, which includes 11 itself).
To test if the number should have the ordinal nd
, you check if n
is 2 greater than a multiple of ten (n % 10 == 2
which includes 2 itself), but isn’t 12 greater than a multiple of 100 (n % 100 != 12
, which includes 12 itself).
To test if the number should have the ordinal rd
, you check if n
is 3 greater than a multiple of ten (n % 10 == 3
, which includes 3 itself), but isn’t 13 greater than a multiple of 100 (n % 100 != 13
, which includes 13 itself).
If all of the conditions are false, then the value of ord
remains th
.
You can test it in live action with the following CodePen demo.
See the Pen Get the Ordinal of a Number by SitePoint (@SitePoint) on CodePen.
Conclusion
In this tutorial, you’ve learned how to retrieve the ordinal of a number. Ordinals can be used in variety of cases, such as displaying dates or ranking in human-readable formats.
If you found this article useful, you may also enjoy the following:
- Quick Tip: How to Convert a Number to a String in JavaScript
- Quick Tip: How to Convert a String to a Number in JavaScript
- Learn to Code with JavaScript
- How to Learn JavaScript Fast: Six Simple Mind Tricks
- 25+ JavaScript Shorthand Coding Techniques
- ES6 in Action: New Number Methods
- ES6 in Action: New String Methods — String.prototype.*
- Truthy and Falsy Values: When All is Not Equal in JavaScript
Frequently Asked Questions (FAQs) about Converting Numbers to Ordinals in JavaScript
How can I convert numbers to ordinal numbers in JavaScript?
Converting numbers to ordinal numbers in JavaScript can be achieved by creating a function that adds the appropriate suffix (st, nd, rd, th) to the number. This function can be created using a switch statement or an if-else statement. The function will take a number as an input and return the number with the correct ordinal suffix.
What are ordinal numbers?
Ordinal numbers are numbers that indicate the position or order of things in a set. They are usually followed by a suffix such as “st”, “nd”, “rd”, or “th”. For example, in the sequence 1st, 2nd, 3rd, 4th, the numbers 1, 2, 3, 4 are ordinal numbers.
Can I use the Intl.PluralRules object to convert numbers to ordinal numbers?
The Intl.PluralRules object is a built-in JavaScript object that provides language-sensitive plural rules. However, it does not directly support converting numbers to ordinal numbers. You would need to create a custom function to achieve this.
How can I format dates with ordinal number suffixes in JavaScript?
Formatting dates with ordinal number suffixes in JavaScript involves converting the day part of the date to an ordinal number. This can be done by creating a function that takes the day part of the date as an input and returns it with the correct ordinal suffix.
Why do I get an error when trying to convert a number to an ordinal number?
If you’re getting an error when trying to convert a number to an ordinal number, it could be due to several reasons. One common reason is that the input to the function is not a number. Make sure that the input is a number before trying to convert it to an ordinal number.
Can I convert numbers to ordinal numbers in other programming languages?
Yes, converting numbers to ordinal numbers is a common task in many programming languages. The method to achieve this varies from one language to another. In some languages, there might be built-in functions or libraries that can do this.
How can I handle large numbers when converting to ordinal numbers?
When dealing with large numbers, you only need to consider the last two digits to determine the correct ordinal suffix. For example, for the number 1234, you would consider the number 34 to determine the suffix.
Can I convert negative numbers to ordinal numbers?
Ordinal numbers are typically used to indicate a position or order in a set, which is usually positive. However, it is technically possible to convert negative numbers to ordinal numbers by adding the appropriate suffix to the absolute value of the number.
How can I convert numbers to ordinal numbers in different languages?
Converting numbers to ordinal numbers in different languages involves understanding the rules for ordinal numbers in those languages. In some languages, there might be built-in functions or libraries that can do this.
Can I convert decimal numbers to ordinal numbers?
Ordinal numbers are typically used with whole numbers. However, it is technically possible to convert decimal numbers to ordinal numbers by rounding the number to the nearest whole number and then adding the appropriate suffix.
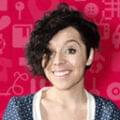
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.