Key Takeaways
- JavaScript provides four ways to convert a number to a string: String Interpolation, String Concatenation, toString() method, and String() constructor function. The choice of method depends on the specific needs and use case.
- String Interpolation and String Concatenation are best used when incorporating numbers within a string. However, these methods can make the code less readable, especially with String Concatenation which might lead to unexpected results when used with more than one number.
- The main difference between using String() and toString() is that String() works with undefined and null values, whereas toString() doesn’t. Therefore, if there’s a chance that a value could be undefined or null, it’s safer to use String().
- String Interpolation: When inserting a number value within a string. For example, displaying text on a webpage like “You have used 7 credits out of 24”. You can also use Concatenation but beware.
- String or toString(): When changing the type of a number value to a String. For example, using numbers as inputs to functions or APIs that expect a string.
String
andtoString()
are mostly the same but treatundefined
andnull
variables differently.
Convert a Number to a String Using Interpolation
Interpolation is probably the most readable way of using numbers in strings. Instead of manually converting the number to a string, you can insert it into a string using this method. To use interpolation, wrap a string with backticks (`
) instead of quotation marks ("
or '
). Then, in the string, you can insert any variable using`${}`
as a placeholder. This is called a template literal and has a variety of other great benefits.
For example:
const number = 99;
console.log(`${number} percent of people love JavaScript`); // "99% of people love JavaScript"
Since the string that’s being logged into the console is wrapped with backticks, you can insert a variable into the string using ${}
.
You can see the example in action in the following CodePen demo.
See the Pen String Interpolation in JavaScript by SitePoint (@SitePoint) on CodePen.
Convert a Number to a String Using String Concatenation
The second approach is string concatenation. You can convert a number to a string using the+
operator.
For example:
console.log(10 + "USD"); //"10USD"
console.log(10 + ""); //"10"
See the Pen Convert Number to String with Concatenation by SitePoint (@SitePoint) on CodePen.
Although this approach is efficient (as it requires the least amount of code), it can make the code less readable.A string concatenation caveat
When using this approach with more than one number, an unexpected result might happen. For example:const a = 2000;
const b = 468;
console.log(a + b + " motorway"); // "2468 motorway"
Since a + b
is evaluated first before reaching the string, the operation is a numerical addition rather than a string concatenation. Once a string variable or literal is reached, the operation becomes a string concatenation. So, the result is 2468 motorway
.
However, try changing the code to the following:
const a = 2000;
const b = 468;
console.log("it is " + a + b + " motorway"); // "it is 2000468 motorway"
Because "it is" + a
is evaluated first, the +
operator is used for string concatenation for the rest of the expression. So, instead of an addition operation between a
and b
like the previous example, it becomes a string concatenation operation between the two.
This can be solved using parentheses:
const a = 2000;
const b = 468;
console.log("it is " + (a + b) + " motorway"); // "it is 2468 motorway"
The addition between a
and b
is performed first, which leads to the addition operation between the two variables. Then, string concatenation is used for the rest of the expression since the first operand is "it is"
.
Convert a Number to a String Using toString
The third approach is using thetoString()
method. This method is available for all JavaScript data types, including numbers. It converts the value of the number it’s used on and returns it.
For example:
const number = 10;
console.log(number); // 10
console.log(typeof number); // "number"
const numberStr = number.toString();
console.log(numberStr); // "10"
console.log(typeof numberStr); // "string"
This example shows the same result as that of the first approach. You can also see it in action in the following CodePen demo.
See the Pen JS Convert Number to String using toString() by SitePoint (@SitePoint) on CodePen.
Convert a Number to a String Using String
The fourth approach is using theString()
constructor function. This function accepts the variable to convert as a first parameter. It converts the parameter to a string and returns it.
For example:
const number = 10;
console.log(number); // 10
console.log(typeof number); // "number"
const numberStr = String(number);
console.log(numberStr); // "10"
console.log(typeof numberStr); // "string"
When logging the value of number
and its type in the console, the result is 10
and number
respectively. After converting it, the result is 10
as a string and string
respectively.
You can see the example in action in the following CodePen demo.
See the Pen JS Convert Number to String using String() by SitePoint (@SitePoint) on CodePen.
Conclusion
This tutorial shows you four approaches that you can use to convert a number to a string in JavaScript. Although these methods can produce the same results when used with numbers, there are some cases where one approach would be better than the others. The main difference between usingString()
and toString()
is that String()
works with undefined
and null
values, whereas toString()
doesn’t. So, if you have a value that should contain a number but you want to be safe when converting it to a string, you can use String()
.
As for string interpolation and string concatenation, they’re best used when using numbers within a string. Otherwise, using these methods can make the code less readable.
If you found this article useful, you may also enjoy the following:
- Quick Tip: How to Convert a Number to a String in JavaScript
- Learn to Code with JavaScript
- How to Learn JavaScript Fast: Six Simple Mind Tricks
- 25+ JavaScript Shorthand Coding Techniques
- ES6 in Action: New Number Methods
- ES6 in Action: New String Methods — String.prototype.*
- Truthy and Falsy Values: When All is Not Equal in JavaScript
Frequently Asked Questions (FAQs) about Converting Numbers to Strings in JavaScript
What is the significance of converting numbers to strings in JavaScript?
Converting numbers to strings in JavaScript is a common operation in programming. It is particularly useful when you need to display numerical data to the user, perform concatenation operations with strings, or store numbers in a format that is easily readable and manipulable. For instance, if you want to display a number in a text field on a web page, you would need to convert it to a string first, as text fields only accept string data.
How can I convert a number to a string using the toString() method?
The toString() method is a built-in JavaScript function that can be used to convert a number to a string. Here’s a simple example:let num = 123;
let str = num.toString();
console.log(str); // Outputs: "123"
In this code, the number 123 is converted to a string using the toString() method, and the result is then logged to the console.
Can I convert a number to a string without using the toString() method?
Yes, you can convert a number to a string in JavaScript without using the toString() method. One alternative way is to use the String() function. Here’s an example:let num = 123;
let str = String(num);
console.log(str); // Outputs: "123"
In this code, the number 123 is converted to a string using the String() function, and the result is then logged to the console.
How can I convert a floating-point number to a string in JavaScript?
Converting a floating-point number to a string in JavaScript is similar to converting an integer. You can use either the toString() method or the String() function. Here’s an example using the toString() method:let num = 123.45;
let str = num.toString();
console.log(str); // Outputs: "123.45"
In this code, the floating-point number 123.45 is converted to a string using the toString() method, and the result is then logged to the console.
Can I specify a radix when converting a number to a string in JavaScript?
Yes, you can specify a radix (base) when converting a number to a string in JavaScript using the toString() method. The radix can be any integer between 2 and 36. Here’s an example:let num = 123;
let str = num.toString(16);
console.log(str); // Outputs: "7b"
In this code, the number 123 is converted to a hexadecimal (base 16) string using the toString() method with a radix of 16, and the result is then logged to the console.
How can I convert a number to a string in scientific notation in JavaScript?
To convert a number to a string in scientific notation in JavaScript, you can use the toExponential() method. Here’s an example:let num = 123;
let str = num.toExponential();
console.log(str); // Outputs: "1.23e+2"
In this code, the number 123 is converted to a string in scientific notation using the toExponential() method, and the result is then logged to the console.
How can I convert a number to a string with a specific number of decimal places in JavaScript?
To convert a number to a string with a specific number of decimal places in JavaScript, you can use the toFixed() method. Here’s an example:let num = 123.456;
let str = num.toFixed(2);
console.log(str); // Outputs: "123.46"
In this code, the number 123.456 is converted to a string with two decimal places using the toFixed() method, and the result is then logged to the console.
Can I convert a string back to a number in JavaScript?
Yes, you can convert a string back to a number in JavaScript using the Number() function, the parseInt() function, or the parseFloat() function, depending on the specific requirements. Here’s an example using the Number() function:let str = "123";
let num = Number(str);
console.log(num); // Outputs: 123
In this code, the string “123” is converted back to a number using the Number() function, and the result is then logged to the console.
What happens if I try to convert a non-numeric string to a number in JavaScript?
If you try to convert a non-numeric string to a number in JavaScript, the result will be NaN (Not a Number). Here’s an example:let str = "abc";
let num = Number(str);
console.log(num); // Outputs: NaN
In this code, the non-numeric string “abc” is attempted to be converted to a number using the Number() function, and the result is NaN.
Can I check if a string can be converted to a number in JavaScript?
Yes, you can check if a string can be converted to a number in JavaScript using the isNaN() function. Here’s an example:let str = "123";
let isNum = !isNaN(str);
console.log(isNum); // Outputs: true
In this code, the string “123” is checked to see if it can be converted to a number using the isNaN() function, and the result is then logged to the console.
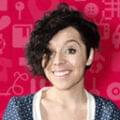
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.