Simple jQuery code snippet to get the time since an event happened. Useful for tracking how long the user is taking to interact with the page elements.
var last, diff;
$('div').click(function(event) {
if ( last ) {
diff = event.timeStamp - last
$('div').append('time since last event: ' + diff + '');
} else {
$('div').append('Click again.');
}
last = event.timeStamp;
});
or simply
new Date().getTime()
Don’t forget to put your jQuery code inside a document ready function. See 4 different jQuery document ready examples for more info.
Also see: Change CSS with jQuery
Source
Frequently Asked Questions (FAQs) about jQuery Timestamp and getTime()
What is the difference between jQuery timestamp and JavaScript getTime()?
jQuery timestamp and JavaScript getTime() both deal with time, but they are used in different contexts. jQuery timestamp is a property of the event object, which indicates the number of milliseconds that have passed since the UNIX epoch (January 1, 1970) at the time the event occurred. This is useful for tracking the exact time of user interactions with your web page.
On the other hand, JavaScript getTime() is a method of the Date object. It returns the number of milliseconds since the UNIX epoch at the time the Date object was created. This is useful for operations like comparing dates or calculating the time elapsed between two dates.
How can I use jQuery timestamp in my code?
jQuery timestamp is a property of the event object, so you can access it in any event handler function. Here’s an example of how to use it:$(document).click(function(event) {
console.log(event.timeStamp);
});
In this code, whenever the user clicks anywhere on the document, the timestamp of the click event is logged to the console.
Can I use jQuery timestamp to measure the time elapsed between two events?
Yes, you can use jQuery timestamp to measure the time elapsed between two events. Here’s an example:var startTime;
$(document).on('mousedown', function(event) {
startTime = event.timeStamp;
}).on('mouseup', function(event) {
var elapsedTime = event.timeStamp - startTime;
console.log(elapsedTime);
});
In this code, when the user presses the mouse button, the timestamp of the mousedown event is stored in startTime
. When the user releases the mouse button, the timestamp of the mouseup event is subtracted from startTime
to calculate the time elapsed between the two events.
How can I get the current time with jQuery?
jQuery does not provide a built-in method to get the current time. However, you can use JavaScript’s Date object to get the current time, like this:var currentTime = new Date().getTime();
console.log(currentTime);
This code creates a new Date object representing the current date and time, then calls the getTime() method to get the number of milliseconds since the UNIX epoch. This value is then logged to the console.
Can I use jQuery timestamp and JavaScript getTime() interchangeably?
While both jQuery timestamp and JavaScript getTime() return the number of milliseconds since the UNIX epoch, they are not interchangeable because they serve different purposes. jQuery timestamp is used to track the time of user interactions with your web page, while JavaScript getTime() is used for operations involving dates and times, such as comparing dates or calculating the time elapsed between two dates. Therefore, you should choose the one that best suits your needs.
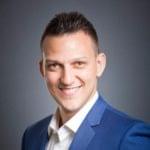
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.