This article will show you how you can build a navigation bar (“navbar”) in React, while covering everything from design considerations to implementation and accessibility best practices.
One of the essential parts of any web application is the navigation bar, as it allows users to navigate through different pages and sections of the website.
So it’s important that you build a navigation bar that has the required links, along with the right accessibility measures to ensure that your users are able to find their way within your application.
Key Takeaways
- A navbar is a crucial element of any website, as it provides users with a means to navigate through different pages and sections.
- React allows for the creation of reusable and modular components, making it an excellent choice for building complex UIs like navbars.
- Accessibility should be a top priority when creating a navbar, ensuring that all users, including those with disabilities, can effectively navigate your website.
What is a Navbar?
A navbar is a user interface element that typically appears at the top or side of a web page.
It serves as a navigation aid, providing links or buttons that allow users to access different sections or pages within the website.
It’s essential for creating a seamless and intuitive user experience, as it helps users understand the structure and hierarchy of the website, and enables them to move effortlessly between different parts of the application.
Here are a few examples of well-designed navbars:
Airbnb. Airbnb’s navbar features a clean and minimalist design, with clear links to various sections of the website, such as “Places to stay”, “Experiences”, and “Online Experiences”.
Dropbox. The Dropbox navbar is simple yet effective, with a prominent “Products” dropdown menu that allows users to explore different offerings.
Building a Navbar in React
Now that we understand the importance of navbars, let’s dive into the process of building one using React.
For this example, we’ll create a navbar for an ecommerce website called “ShopNow”.
Step 1: Designing the navbar
Before we start coding, it’s essential to have a clear design in mind for our navbar.
For our ShopNow website, we’ll aim for a simple yet modern design with the following elements:
- a logo on the left side
- links to different sections of the website (such as “Products”, “About Us” and “Contact”)
- a shopping cart icon with a badge displaying the number of items in the cart
- a user icon for account-related actions (such as “Sign In” and “My Account”)
Here’s a mockup of how our navbar might look.
Step 2: Setting up the React project
Before we start building our navbar, we’ll need to set up a new React project. You can create a new React project using Create React App by running the following command in your terminal:
npx create-react-app shopnow
Once the project is set up, navigate to the project directory and start the development server:
cd shopnow
npm start
Step 3: Creating the navbar component
With SPA frameworks like React, it’s important that you design and think in reusable, independent components. So it’s crucial to build components that you can reuse throughout your application.
One application of a reusable component is a navigation bar. You can create a reusable navbar component that you can reuse within your application.
Let’s create a new file called Navbar.js
in the src
directory and add the following code:
import React from 'react';
import './Navbar.css';
const Navbar = () => {
return (
<nav className="navbar">
{/* Navbar content will go here */}
</nav>
);
};
export default Navbar;
We’ve created a functional component called Navbar
that returns a <nav>
element with a class name of navbar
. We’ll also create a new file called Navbar.css
in the same directory to style our navbar.
Step 4: Adding the navbar structure
Now let’s add the structure of our navbar inside the Navbar
component:
import React from 'react';
import './Navbar.css';
const Navbar = () => {
return (
<nav className="navbar">
<div className="navbar-left">
<a href="/" className="logo">
ShopNow
</a>
</div>
<div className="navbar-center">
<ul className="nav-links">
<li>
<a href="/products">Products</a>
</li>
<li>
<a href="/about">About Us</a>
</li>
<li>
<a href="/contact">Contact</a>
</li>
</ul>
</div>
<div className="navbar-right">
<a href="/cart" className="cart-icon">
<i className="fas fa-shopping-cart"></i>
<span className="cart-count">0</span>
</a>
<a href="/account" className="user-icon">
<i className="fas fa-user"></i>
</a>
</div>
</nav>
);
};
export default Navbar;
In this code, we’ve divided the navbar into three sections:
navbar-left
. This contains the logo, which is a link to the home page.navbar-center
. This contains an unordered list (<ul>
) of navigation links.navbar-right
. This contains a shopping cart icon with a badge displaying the number of items in the cart and a user icon for account-related actions.
We’ve also used Font Awesome icons for the cart and user icons, which you’ll need to include in your project by following the instructions on their site.
Step 5: Styling the navbar
Now that we have the structure in place, let’s add some styles to make our navbar look more appealing. Open the Navbar.css
file and add the following styles:
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
background-color: #333;
color: #fff;
padding: 1rem;
}
.navbar-left .logo {
font-size: 1.5rem;
font-weight: bold;
color: #fff;
text-decoration: none;
}
.navbar-center .nav-links {
list-style-type: none;
display: flex;
margin: 0;
padding: 0;
}
.navbar-center .nav-links li {
margin-right: 1rem;
}
.navbar-center .nav-links a {
color: #fff;
text-decoration: none;
}
.navbar-right {
display: flex;
align-items: center;
}
.navbar-right .cart-icon,
.navbar-right .user-icon {
color: #fff;
text-decoration: none;
margin-left: 1rem;
position: relative;
}
.navbar-right .cart-count {
background-color: #f44336;
color: #fff;
border-radius: 50%;
padding: 0.2rem 0.5rem;
font-size: 0.8rem;
position: absolute;
top: -0.5rem;
right: -0.5rem;
}
Step 6: Importing and rendering the navbar
Finally, we need to import the Navbar
component and render it in our App.js
file:
import React from 'react';
import Navbar from './Navbar';
function App() {
return (
<div>
<Navbar /v>
{/* Rest of your app content goes here */}
</div>
);
}
export default App;
Now, when you run your React app, you should see the navbar at the top of the page, as shown in this Pen.
See the Pen Untitled by SitePoint (@SitePoint) on CodePen.
Click the 0.5x button in the Pen above to see the desktop layout of the navbar.
Step 7: Best Practices: Enhancing Accessibility
Building an accessible navbar is crucial for ensuring that all users can effectively navigate your website.
Here are some best practices to follow.
Use semantic HTML. We’ve already used semantic HTML elements in our navbar code. The main navigation section is wrapped in a
<nav>
element, the list of links is contained within an unordered list (<ul>
) with each link as a list item (<li>
), and the links themselves are represented by<a>
elements.Add ARIA roles and attributes. We can add the
role="navigation"
attribute to the<nav>
element to indicate that it is a navigation section:<nav className="navbar" role="navigation"> {/* ... */} </nav>
Ensure keyboard accessibility. >React provides built-in support for keyboard accessibility. By default, all interactive elements (such as links and buttons) can be focused using the tab key and activated using the Enter or Space keys. However, we should test this functionality to ensure it works as expected in our navbar.
Provide clear focus styles. To provide clear focus styles, we can add CSS rules for the
:focus
state of our links and icons. Here’s an example of how we can style the focused links in our navbar:.navbar-center .nav-links a:hover { color: red; }
You can adjust the
outline
andoutline-offset
properties to achieve the desired focus style.Use descriptive link text. In our navbar code, we’ve already used descriptive link text for the navigation links (“Products”, “About Us”, “Contact”). However, we should ensure that the link text for the cart and user icons is also descriptive. One way to achieve this is by adding visually hidden text using the
aria-label
attribute:<a href="/cart" className="cart-icon" aria-label="Shopping Cart"> <i className="fas fa-shopping-cart"></i> <span className="cart-count">0</span> </a> <a href="/account" className="user-icon" aria-label="User Account"> <i className="fas fa-user"></i> </a>
Make the layout responsive. To make our navbar responsive, we can use CSS media queries to adjust the layout and styles based on the screen size. For example, we could hide the navigation links on smaller screens and replace them with a hamburger menu:
@media (max-width: 768px) { .navbar-center .nav-links { display: none; } .navbar-right { display: flex; align-items: center; } .navbar-right .hamburger-menu { display: block; color: #fff; font-size: 1.5rem; cursor: pointer; } }
In the JavaScript code, we’d need to handle the toggling of the navigation links when the hamburger menu is clicked.
By implementing these accessibility best practices, we can ensure that our navbar is usable and inclusive for all users, including those with disabilities. Remember to test your application with various screen readers, keyboard navigation, and other assistive technologies to ensure it meets accessibility standards.
Wrapping Up
And that’s it! You now have yourself a navigation bar that you can reuse across your application that ensures your users are able to find their way within your app.
To check out the full code, explore the CodePen demo.
Frequently Asked Questions (FAQs)
How can I make the navbar responsive?
To make your navbar responsive, you can use CSS media queries to adjust the layout and styles based on the screen size. Additionally, you may want to consider using a responsive navigation pattern, such as a hamburger menu or a dropdown menu, for smaller screen sizes.
Can I use external libraries or components to create a navbar in React?
Yes, there are several third-party libraries and component libraries available that provide pre-built and customizable navbar components for React. Some popular options include React Bootstrap, Material-UI, and Ant Design. However, it’s important to evaluate the compatibility, performance, and accessibility of these components before using them in your project.
How can I handle navigation in a React application with a navbar?
In React, you can handle navigation using the React Router library, which provides a way to define routes and navigate between different components or pages in your application. You can map the links in your navbar to specific routes, making it easy for users to navigate through your app.
How can I add animations or transitions to the navbar?
You can add animations or transitions to your navbar using CSS or JavaScript. For example, you can use CSS transitions or animations to create smooth hover effects or sliding animations for dropdown menus. Alternatively, you can use a JavaScript animation library like React Transition Group or Framer Motion to create more complex animations and transitions.
Can I use the same navbar component across multiple pages or routes in my React application?
Yes, one of the benefits of using React is the ability to create reusable components like the navbar. You can import and render the same navbar component across multiple pages or routes in your application, ensuring a consistent user experience throughout your website.
How can I add a search functionality to my navbar?
To add search functionality to your navbar, you can create a search input field and handle the user’s input using React state and event handlers. You can then use this input to filter or search through your data and display the relevant results on a separate page or component.
How can I style the active or current link in the navbar?
To style the active or current link in your navbar, you can use the NavLink component provided by React Router. This component allows you to apply a specific style or class to the active link based on the current URL or route. Alternatively, you can use a custom hook or component to track the current URL and apply styles accordingly.
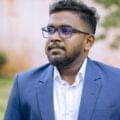
I'm a web developer with experience in Python, Java, JavaScript, MySQL, and React. I started to share my knowledge through blogs with the development community in early 2021.