Asset Access Restriction Methods – Block Unwanted Visitors
Key Takeaways
- Implementing access control to your assets is crucial for security. Whitelisting is a recommended approach, where a gatekeeper only allows trusted entities to access your assets. This gatekeeper can be placed at the HTTP server or the application code, providing tools to check the HTTP_HOST.
- The HTTP server can handle access control efficiently without needing to fire up the application code for every request. Depending on the server, different methods such as mod_rewrite or Allow/Deny in Apache, or HttpRefererModule in Nginx can be used. However, the scalability of this approach can be a challenge if there are many domains to manage or frequent changes.
- Access control at the application code level offers more flexibility. This method can also handle iframe situations, where the referrer doesn’t provide information about the iframe status. However, both HTTP server and application code methods are not foolproof and a token-based GateKeeper such as OAuth is recommended for enhanced security.
When building an awesome web app or website, we sometimes want people to be able to embed parts of our web app/website into their own. That could be an iframe holding a ‘like’ button, a simple image that they want to reuse or even our entire app embedded in an iframe.
But how do we control who has access, who is allowed to use up our bandwidth and query our service?
We define the problem as controlling access to assets
By assets we mean: anything that can be queried from our site.
Access Restriction: Allow some, block all
When talking about access control, we enter the domain of security. And when talking security, whitelisting should be the approach taken to tackle the problem. It is easier to control who is allowed to access your assets than it is to control who is not. It is simply impossible to know all the boogie monsters of the internet.
To protect our assets, we hire a gatekeeper to only let the ones we trust in. Once hired, we give him access to a whitelist we control, and let him do all the heavy lifting. Problem solved. But how should the gatekeeper lift?
Lifting tactics
Depending on how secure you want the gatekeeper to be and what the client asked for, different tactics can be used.
A common approach used is checking the Referer header. That method has 3 big drawbacks:
- The referer is also set when people access your website using a link
- The referer is sent to your server by the client, and could be altered
- The referer might not be set at all
However, for static assets such as images, js and css, those drawbacks are not an issue. Your assets should only be loaded when users are visiting our website directly (or from a trusted site). The general idea is to block others hotlinking them. The referer will thus always be on your whitelist. Unless you don’t trust yourself – but then you have bigger issues.
Bro, do you even lift?
Depending on the setup used, the query made passes through a series of gates. The simple setup is: Client -> HTTP server -> application code
So where does your gatekeeper sit? The client is a de facto no go for access control because he is an unreliable lying piece of human. The HTTP server and the application code on the other hand are useful options. Both give us strong tools to check the HTTP_HOST
.
HTTP servers know how to lift
The strength in having your HTTP server handle your access control is speed. There is no need to fire up the application code for every request. This can drastically improve performance because we do not need to load an entire application stack/thread (e.g. mod_php) into memory.
Depending on your HTTP server, different solutions are available.
Apache
In Apache, there are two different methods. We can use mod_rewrite or Allow/Deny.
The mod_rewrite method:
# Turn mod_rewrite on
RewriteEngine On
# if it is not trusted.domain.tld block it
RewriteCond %{HTTP_REFERER} !^trusted\.domain\.tld$ [NC]
RewriteCond %{HTTP_REFERER} !^trusted\.tld$ [NC]
RewriteRule ^ - [F]
mod_rewrite is supported by most hosting providers.
The Allow/Deny method:
#specify the files to guard, block all the assets
<files "*.*">
#block everyone
Deny from all
#allow trusted ones
Allow from trusted.tld trusted.domain.tld
</files>
Not all hosts support those settings.
Nginx
The HttpRefererModule in nginx gives us the really cool valid_referers
: So all that we need to do is, return http code 444 when a non-trusted domain tries to access our assets:
… nonstandard code 444 closes the connection without sending any headers…
location / {
valid_referers trusted.tld trusted.domain.tld;
if ($invalid_referer) {
return 444;
}
}
HTTP servers don’t think
The big problem here is scalability: what if we have 1000 domains that need to be able to access our assets? What if the list of domains changes frequently?
For every little edit, we would need to dive into our configuration files – and the more you change manually, the more can go wrong.
Application code knows what to do
Having your access control on your application code level means a lot more flexibility. One could have his gatekeeper up and running in no time:
<?php
//the whitelist we control
$whitelist = array(
'trusted.tld',
'trusted.domain.tld'
);
//the referer
$referer = parse_url($_SERVER["HTTP_REFERER"], PHP_URL_HOST);
//the gatekeeper
if ( !in_array($referer, $whitelist) )
{
throw new GateKeeperDoesNotApprove;
}
What about those iframes?
As mentioned, relying on the referer isn’t always a good idea. It is not only data from our unreliable human, it also gives us no clue as to whether we are in an iframe or not. There is simply no way of knowing.
We could however hire a hitman to help our GateKeeper. Our hitman will be dispatched to the humans that look suspicious (e.g. the ones with an untrusted referer). The hitman will use JS as his weapon:
document.getElementById('container').innerHTML = '';
alert('You just got killed');
Sadly enough, someone arriving from an untrusted domain has the same referer as someone else that accesses us using an iframe from that untrusted domain. Assets, however, will have the referer set to our domain (even in an iframe situation) – so sending a hitman here is overkill. Simply denying access is enough – or you could send a random kitten image.
That is why we have our hitman check if we are in an iframe. If so, we have him kill our target:
if (top.location.href != self.location.href) {
//kill the target
}
The only thing we need to do know is to have our GateKeeper add the hitman to the payload sent to the client. Easy!
//template.tpl
If ( Gatekeeper::doesNotApprove() )
{
Gatekeeper::sendHitman();
}
//gatekeeper
class Gatekeeper
{
private static $whitelist = array();
public static function doesNotApprove()
{
return !in_array(
parse_url($_SERVER["HTTP_REFERER"], PHP_URL_HOST),
self::$whitelist
);
}
public static function sendHitman()
{
print '<script>if (top.location.href != self.location.href) { document.getElementById('container').innerHTML = '';alert('You just got killed');}</script>';
}
}
This code is not production proof. It serves as an example.
What about real security?
The solutions supplied here will guard you against most boogie monsters. But both solutions are not fool proof. The first uses data from the client, the second is javascript that is run by the client.
The secure way is to use a token-based GateKeeper. OAuth is probably the guy you want for the job here, but that is outside of the scope of this article.
Frequently Asked Questions (FAQs) on Asset Access Restriction Methods
What are the different methods of asset access restriction?
Asset access restriction methods are strategies used to prevent unauthorized access to digital assets. These methods include IP blocking, which involves blocking access from specific IP addresses; Geo-blocking, which restricts access based on geographical location; and User-Agent blocking, which prevents access from specific browsers or devices. Other methods include HTTP referrer blocking, which restricts access based on the referring website, and Password protection, which requires users to enter a password to gain access.
How does IP blocking work in asset access restriction?
IP blocking is a method used to prevent specific IP addresses from accessing a digital asset. This is done by adding the IP addresses to a ‘blacklist’ in the server’s configuration file. Any requests coming from these IP addresses will be denied, effectively blocking them from accessing the asset.
What is the purpose of Geo-blocking in asset access restriction?
Geo-blocking is used to restrict access to digital assets based on geographical location. This is often used to comply with regional licensing agreements or to prevent access from regions known for cyber attacks. Geo-blocking works by determining the user’s location based on their IP address.
How can User-Agent blocking be used in asset access restriction?
User-Agent blocking is a method used to prevent specific browsers or devices from accessing a digital asset. This is done by identifying the User-Agent string, which is sent by the browser or device when making a request to the server. If the User-Agent string matches one on the server’s ‘blacklist’, the request will be denied.
What is the role of HTTP referrer blocking in asset access restriction?
HTTP referrer blocking is a method used to restrict access based on the referring website. This is done by checking the HTTP referrer header, which contains the URL of the website that the request came from. If the referrer is on the server’s ‘blacklist’, the request will be denied.
How does password protection work in asset access restriction?
Password protection is a method used to restrict access to a digital asset by requiring users to enter a password. This is often used for private or sensitive assets. The server will prompt the user for a password when they try to access the asset, and only those who enter the correct password will be granted access.
Can multiple asset access restriction methods be used together?
Yes, multiple asset access restriction methods can be used together to provide a higher level of security. For example, you could use IP blocking to prevent access from specific IP addresses, and then use password protection to further restrict access to authorized users only.
How can I implement asset access restriction methods on my website?
Implementing asset access restriction methods on your website typically involves modifying your server’s configuration file. The exact process will depend on the server software you are using and the specific restriction method you want to implement.
Are there any downsides to using asset access restriction methods?
While asset access restriction methods can provide a high level of security, they can also potentially block legitimate users. For example, IP blocking can block users who are using a VPN or proxy, and Geo-blocking can block users who are traveling abroad. Therefore, it’s important to carefully consider the potential impact on users before implementing these methods.
What are some alternatives to asset access restriction methods?
Alternatives to asset access restriction methods include using a Content Delivery Network (CDN) to distribute your assets, using a firewall to protect your server, or using a security plugin or service that provides a range of security features. These alternatives can provide a high level of security without the potential downsides of asset access restriction methods.
Jeroen Meeus is a developer that knows how to appreciate his glass of CH3CH2OH with well written code to read on a lazy sunday. When frustrated, he tends to share those frustrations at his so-called blog.
Published in
·Bootstrap·Cloud·Miscellaneous·Open Source·Patterns & Practices·PHP·Programming·Web·June 21, 2014
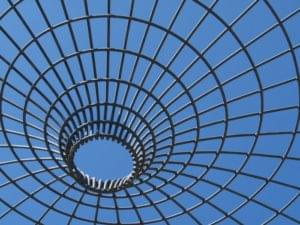
Published in
·CMS & Frameworks·Development Environment·Frameworks·Laravel·News & Opinion·PHP·Standards·Symfony·Web·March 3, 2017
Published in
·CMS & Frameworks·Development Environment·Miscellaneous·Patterns & Practices·PHP·September 29, 2014