Keep up to date on current trends and technologies
Programming - Software Development
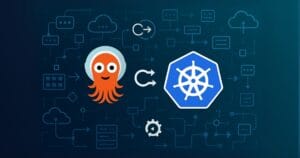
Unleashing the Power of ArgoCD by Streamlining Kubernetes Deployments
Raju Dandigam
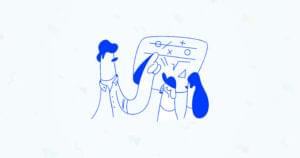
Which Programming Language Should I Learn First in 2024?
Joel Falconer
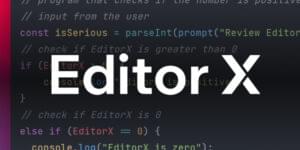
Review: Is Editor X a serious option for Web Developers?
Alex Walker
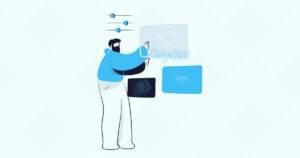
What Is an IDE? How Does It Enable Faster Development?
Joel Falconer
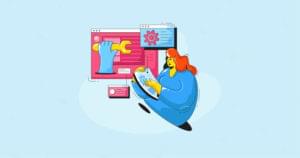
Cron Jobs: A Comprehensive Guide
Reza Lavarian
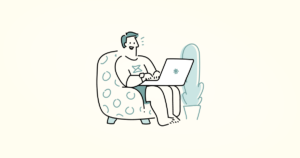
Why Learn to Code? 17 Benefits of Learning to Code
Joel Falconer
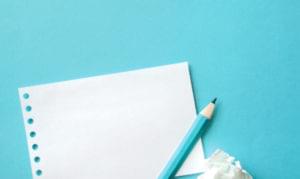
A Guide to Writing Your First Software Documentation
Maria Antonietta Perna
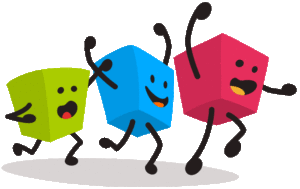
How to Easily Share Code Between Projects with Bit
Jonathan Saring
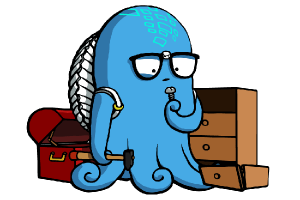
Deepstream: an Open-source Server for Building Realtime Apps
Wolfram Hempel
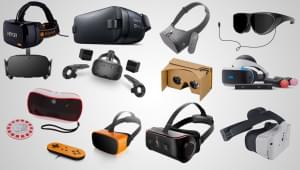
There Are More Virtual Reality Headsets Than You Realize!
Patrick Catanzariti
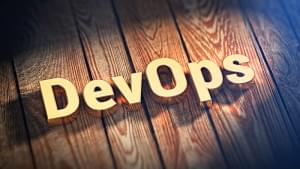
DevOps by Example: Tools, Pros and Cons of a DevOps Culture
Lucero del Alba
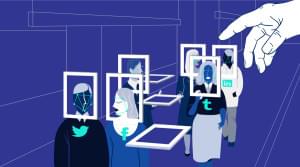
The Future of Doxing in a World of Facial Recognition
Charles Costa
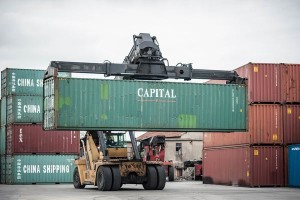
Understanding Docker, Containers and Safer Software Delivery
Lucero del Alba
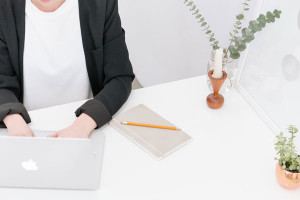
The Importance of Code Reviews
Kitty Giraudel
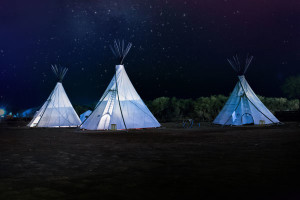
3 Ways to Work More Effectively in a Web Development Team
Camilo Reyes
JavaScript Testing: Unit vs Functional vs Integration Tests
Eric Elliott
Managing Data Storage with Blockchain and BigchainDB
Chris Ward
7 Simple Speed Solutions for MongoDB
Craig Buckler
Deploying from GitHub to a Server
Chris Ward
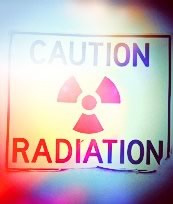
When Bad Software Kills
Alex Walker
How to Set Up Continuous Deployment with Ninefold
Glenn Goodrich
The 100 Year Old Trick to Writing at 240 Words Per Minute
Alex Walker
Increase Productivity with Komodo IDE
George Fekete
Continuous Delivery: The Right Way to Deploy Your Software
Craig Buckler
How to Supercharge Slack for Powerful Workplace Collaboration
Shaumik Daityari
Integrating VictorOps with Salesforce Using its REST Endpoint
Dhaivat Pandya
18 Critical Oversights in Web Development
George Fekete
8 Essential Skills Developers Can Learn in a Weekend
Shaumik Daityari
Debugging in Git with Blame and Bisect
Shaumik Daityari
Semantic Versioning: Why You Should Be Using it
Kitty Giraudel
Using Regular Expressions to Check String Length
M. David Green
10 Tips to Push Your Git Skills to the Next Level
Shaumik Daityari
Showing 32 of 43