- Reasons for URL Shortening
- Acquiring a Google URL Shortener API Key
- Plugin Directory and Files
- Create a Plugin Settings Page
- An Overview of the Google URL Shortener API
- Displaying a Shortened URL in a Meta Box
- Displaying the Short URL in the Front End
- Popular URL Shortener Plugins in the WordPress.org Plugin Directory
- Conclusion
- Frequently Asked Questions (FAQs) about Creating a URL Shortener Plugin for WordPress
URL shortening is a technique in which a URL is made substantially shorter in length and still links to the required page. This is achieved by using a redirect on a domain name that is short, which links to the web page that has the longer URL.
In this tutorial, I’ll show you how to create a URL shortening plugin for WordPress using Google’s URL Shortener API.
Reasons for URL Shortening
Before we start building a URL shortening plugin let’s see a few examples as to why we may need this plugin:
- On Twitter and some other messaging services there is a limit to number of characters a message can contain. So if you’re sending long URLs then it will occupy most of the message.
- Printed books or display signage will often use shortened URLs as they are easy to be read and type.
- QR codes have a character limit. Very long URLs don’t fit, therefore shortening of the URL is required.
Acquiring a Google URL Shortener API Key
To use the Google URL Shortener API you’ll need to acquire an API Key. This API key is used by Google to keep track of your application.
Here are the steps to acquire your API key:
- Visit Google Developers Console.
- Select an existing project or create a new one.
- In the left sidebar, click and expand APIs & auth.
- Next, click APIs. In the list of APIs, make sure the status is ON for the Google URL Shortener API.
- In the sidebar on the left, select Credentials. Then generate a public access key if you haven’t already. This public access key is the API key.
Plugin Directory and Files
Our plugin will contain one directory and one file. Here is the structure:
--url-shortener
-url-shortener.php
To make the plugin installable, we put this code in the url-shortener.php file:
<?php
/*
Plugin Name: URL Shortener
Plugin URI: http://www.sitepoint.com
Description: Create's a Shortened URL of every post.
Version: 1.0
Author: Narayan Prusty
*/
Create a Plugin Settings Page
We need to create a settings page for our plugin where administrator’s can enter the URL Shortener API key. Here is the code to create a settings page using the WordPress Settings API:
<?php
function url_shortener_settings_page()
{
add_settings_section("section", "Enter Key Details", null, "url-shortener");
add_settings_field("url-shortener-input-field", "API Key", "url_shortener_input_field_display", "url-shortener", "section");
register_setting("section", "url-shortener-input-field");
}
function url_shortener_input_field_display()
{
?>
<input type="text" name="url-shortener-input-field" value="<?php echo get_option('url-shortener-input-field'); ?>" />
<?php
}
add_action("admin_init", "url_shortener_settings_page");
function url_shortener_page()
{
?>
<div class="wrap">
<h1>URL Shortener Setting</h1>
<form method="post" action="options.php">
<?php
settings_fields("section");
do_settings_sections("url-shortener");
submit_button();
?>
</form>
</div>
<?php
}
function menu_item()
{
add_submenu_page("options-general.php", "URL Shortener", "URL Shortener", "manage_options", "url-shortener", "url_shortener_page");
}
add_action("admin_menu", "menu_item");
We are storing the API key as a WordPress option with the name url-shortener-input-field
.
This is what the settings page should look like:
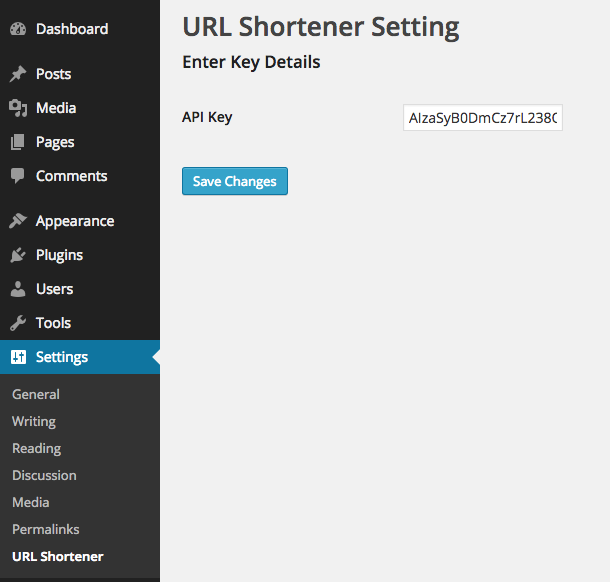
An Overview of the Google URL Shortener API
To shorten a long URL you need to send a POST request to the https://www.googleapis.com/urlshortener/v1/url
URL with your API key and long URL.
Here’s what a sample request looks like:
POST https://www.googleapis.com/urlshortener/v1/url
Content-Type: application/json
{"longUrl": "https://www.sitepoint.com/"}
Here is the sample response looks like:
{
"kind": "urlshortener#url",
"id": "http://goo.gl/fqsT",
"longUrl": "https://www.sitepoint.com/"
}
Note: You cannot send more than 1 million requests using the same API key in a day.
Displaying a Shortened URL in a Meta Box
We want to display the shortened URL in a meta box in the post edit screen. For creating a meta box, we’ll use the Meta Box API and for the URL shortening we’ll use the WordPress HTTP API.
Here’s the code to display our shortened URL in a meta box:
function url_shortener_meta_box_markup($object)
{
$key = get_permalink($object->ID);
if(get_option('url-shortener-input-field', '') != "")
{
if(get_option($key, "") != "")
{
echo get_option($key, "");
return;
}
$url = 'https://www.googleapis.com/urlshortener/v1/url';
$result = wp_remote_post(
add_query_arg(
'key',
get_option('url-shortener-input-field'),
'https://www.googleapis.com/urlshortener/v1/url'
),
array(
'body' => json_encode(array('longUrl' => esc_url_raw($key))),
'headers' => array( 'Content-Type' => 'application/json')
)
);
if(is_wp_error($result)){echo "Error"; return;}
$result = json_decode($result['body']);
$shortlink = $result->id;
update_option($key, $shortlink);
echo $shortlink;
}
}
function url_shortener_meta_box()
{
add_meta_box("url-shortener-meta-box", "Shorten URL", "url_shortener_meta_box_markup", "post", "side", "default", null);
}
add_action("add_meta_boxes", "url_shortener_meta_box");
Here’s how this code works:
- We created a meta box using the
add_meta_box
function. - We’re retrieving the long URL of the post using the
get_permalink()
function. - Then, we’re checking if we already have a short URL of this long URL in the database as a WordPress option. If not, then we’re retrieving it using the HTTP API and storing it as a WordPress option. Otherwise we use the existing short URL.
- This plugin plays nicely with the Google URL shortener web service as it doesn’t request a new URL every time, instead it stores it once it’s retrieved.
This is what the meta box looks like in post edit screen:

Displaying the Short URL in the Front End
We also want to display the shortened URL below every post. Here’s the code to do just that:
function url_shortener_content_filter($content)
{
if($GLOBALS['post']->post_type != "post")
return;
$key = get_permalink($GLOBALS['post']->ID);
if(get_option('url-shortener-input-field', '') != "")
{
if(get_option($key, "") != "")
{
$content = $content . "<p><b>Shortern URL: </b>" . get_option($key, "") . "</p>";
return $content;
}
$url = 'https://www.googleapis.com/urlshortener/v1/url';
$result = wp_remote_post(
add_query_arg(
'key',
get_option('url-shortener-input-field'),
'https://www.googleapis.com/urlshortener/v1/url'
),
array(
'body' => json_encode(array('longUrl' => esc_url_raw($key))),
'headers' => array( 'Content-Type' => 'application/json')
)
);
if(is_wp_error($result)){echo "Error"; return;}
$result = json_decode($result['body']);
$shortlink = $result->id;
update_option($key, $shortlink);
$content = $content . "<p><b>Shortern URL: </b>" . $shortlink . "</p>";
}
return $content;
}
add_filter("the_content", "url_shortener_content_filter");
This is how this code works:
- We are first checking to make sure WordPress is processing a post. If it’s a page or custom post type, then we aren’t displaying the short URL. However, if you want to display it in every page then remove the first two lines from the function code.
- Then we’re doing everything the same as we did while displaying the short URL in the meta box. The only difference is that instead of echoing it, we are concatenating it to the post content.
Here is how it looks on front end:
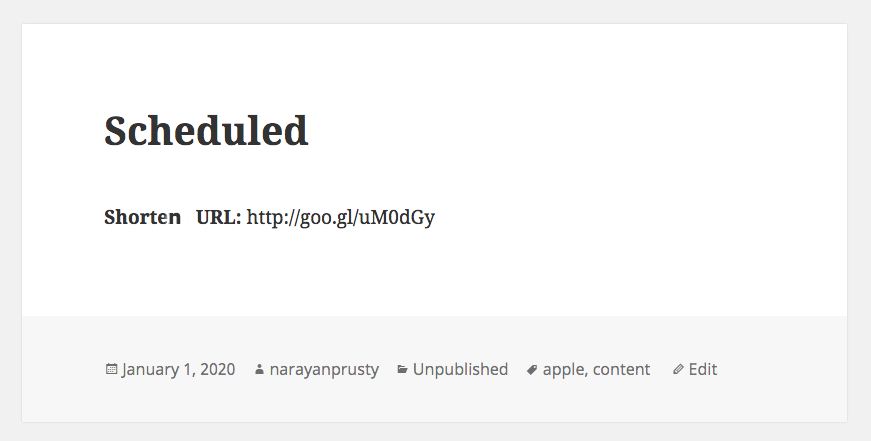
Now we’re done with building a awesome URL shortener plugin for WordPress!
Popular URL Shortener Plugins in the WordPress.org Plugin Directory
If you’d like to check out existing plugins, two of the most popular URL Shortener plugins in the WordPress.org Plugin Directory are URL Shortener and WP URL Shorten.
WP URL Shorten uses ref.li to shorten URLs. Ref.li provides real time stats and other traffic information of people visiting your site via their shortened URL.
URL Shortener plugin lets you choose between Bit.ly, Su.pr, YOURLS, Goo.gl and many other services. This plugin can also generate QR Codes.
Conclusion
In this tutorial, I’ve shown you how to easily build your own URL shortening plugin. You can now go ahead and expand on this to add more features such as QR code support and use other URL shortening services. Please share your experience with your own plugins below.
Frequently Asked Questions (FAQs) about Creating a URL Shortener Plugin for WordPress
What is a URL shortener plugin and why do I need it for my WordPress site?
A URL shortener plugin is a tool that helps you create shorter, more manageable URLs for your WordPress posts and pages. These shortened URLs are easier to share on social media, in emails, and other platforms. They also make your links look cleaner and more professional. If you have a WordPress site with long and complex URLs, a URL shortener plugin can greatly improve your user experience and SEO.
How does a URL shortener plugin work?
A URL shortener plugin works by creating a unique, shorter version of your original URL. When a user clicks on the shortened URL, they are redirected to the original long URL. This process is seamless and does not affect the user’s browsing experience. The plugin also tracks the number of clicks on the shortened URL, providing valuable data for your marketing efforts.
Can I customize my shortened URLs?
Yes, most URL shortener plugins allow you to customize your shortened URLs. You can add your own keywords to make the URLs more meaningful and relevant. This not only makes your URLs easier to remember but also improves your SEO.
Is it possible to restore the ‘Get Shortlink’ button in WordPress?
Yes, it is possible to restore the ‘Get Shortlink’ button in WordPress. This button was removed in WordPress 4.4 but you can bring it back by using a plugin or adding a code snippet to your theme’s functions.php file.
Are there any free URL shortening plugins for WordPress?
Yes, there are several free URL shortening plugins available for WordPress. Some of the popular ones include URL Shortify, Pretty Links, and Bitly. These plugins offer basic URL shortening features and are a good starting point if you are new to URL shortening.
How can I track the performance of my shortened URLs?
Most URL shortener plugins provide analytics features that allow you to track the performance of your shortened URLs. You can see how many times a URL has been clicked, the geographical location of the users, the referral sources, and more. This data can help you understand your audience better and optimize your marketing strategies.
Can I use a URL shortener plugin if I’m not tech-savvy?
Absolutely! Most URL shortener plugins are designed to be user-friendly and do not require any technical knowledge. They come with easy-to-use interfaces and detailed instructions. If you can navigate your WordPress dashboard, you can use a URL shortener plugin.
Can a URL shortener plugin improve my SEO?
Yes, a URL shortener plugin can improve your SEO. Shortened URLs are easier for search engines to crawl and index. They also improve user experience by making your links more manageable and shareable, which can boost your site’s ranking in search engine results.
Can I use a URL shortener plugin for my affiliate links?
Yes, you can use a URL shortener plugin for your affiliate links. In fact, it’s highly recommended. Shortened URLs look cleaner and more professional, which can increase click-through rates and conversions. Some plugins also allow you to add a nofollow attribute to your affiliate links, which is good for SEO.
Are there any risks associated with using a URL shortener plugin?
While URL shortener plugins offer many benefits, there are also some risks. For example, if the plugin’s server goes down, your shortened URLs may stop working. Also, some users may be wary of clicking on shortened URLs because they can’t see the destination URL. Therefore, it’s important to choose a reliable plugin and use shortened URLs judiciously.

Narayan is a web astronaut. He is the founder of QNimate. He loves teaching. He loves to share ideas. When not coding he enjoys playing football. You will often find him at QScutter classes.