This tutorial will show you how to encrypt arbitrarily large messages with asymmetric keys and a PHP library called phpseclib.
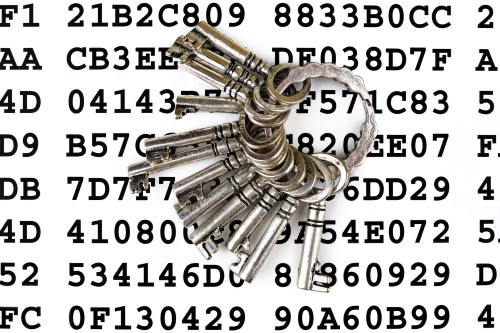
Introduction
Most of us understand the need to encrypt sensitive data before transmitting it. Encryption is the process of translating plaintext (i.e. normal data) into ciphertext (i.e. secret data). During encryption, plaintext information is translated to ciphertext using a key and an algorithm. To read the data, the ciphertext must be decrypted (i.e. translated back to plaintext) using a key and an algorithm.
An encryption algorithm is a series of mathematical operations applied to the numerical value(s) of the key and the numerical values of the characters in a string of plaintext. The results are the ciphertext. The larger the key, the more secure the ciphertext.
A core problem to be solved with any encryption algorithm is key distribution. How do you transmit keys to those who need them in order to establish secure communication?
The solution to the problem depends on the nature of the keys and algorithms.
Encryption Algorithms and Keys
There are two basic types of encryption algorithms:
1. Symmetric Algorithms that use the same key for both encryption and decryption.
2. Asymmetric Algorithms that use different keys for encryption and decryption.
If a symmetric algorithm is chosen, both the sender and the receiver must have the same key. If the sender and receiver are in different locations, the transmission of the key itself becomes a point of vulnerability.
If an asymmetric algorithm is chosen, there are two keys: a public key and a private key. Data encrypted with a public key can only be decrypted with the corresponding private key. The receiver first transmits their public key to the sender. The sender uses that public key to encrypt the message and then transmits the message to the receiver. The receiver decrypts the message with their private key.
With an asymmetric algorithm the receiver can transmit the public key to whomever they wish without fear of compromise because only the holder of the private key can decrypt the message.
Asymmetric algorithms solve the key distribution problem.
Selection of Encryption Algorithms
Strong cryptographic algorithms are based on advanced mathematics and number theory. Generally, a cryptographic algorithm is “strong” if, for a given key size, it would take an unfeasibly long time for someone without the key to decrypt the message.1
Because of the complexity of cryptographic algorithms, selecting one over another can be confusing to those without a strong background in mathematics. So, many commercial and government entities rely on the National Institute of Standards and Technology (NIST) – a non-regulatory US agency – for recommendations on strong cryptography.
NIST specifies three asymmetric algorithms: RSA, DSA and ECDSA. ECDSA is a relative new comer, so the two common asymmetric algorithms are RSA and DSA. RSA is often a preferred commercial algorithm because the standard easily allows for larger key size. 2.
In 2001, NIST selected the Rijndael algorithm as its preferred symmetric algorithm to replace its aging DES (Data Encryption Standard).3
The Problem with RSA Keys
Although asymmetric algorithms solve the key distribution problem, there is another problem. The nature of the RSA algorithm is such that it is only able to encrypt a limited amount of plaintext. For example, if your key size is 2048 bits, then you are limited to 256 bytes (at most) of plaintext data that can be encrypted.4
Here’s a summary our problem:
1. Asymmetric Keys are easy to exchange securely, but have a limited message size.
2. Symmetric Keys have an unlimited message size, but are difficult to exchange securely.
The Solution
The solution to the problem is to encrypt the message with a symmetric key, then asymmetrically encrypt the key and attach it to the message.
When the message arrives at the receiver, they will have both the symmetric key and the message. The receiver extracts the encrypted symmetric key, asymmetrically decrypts it, and then uses it to decrypt the rest of the message.
The following section explains both encryption and decryption processes step by step.
Encryption
- The receiver generates a key pair and transmits their public key to the sender.
- The sender generates a random symmetric key and uses it to encrypt the large message.
- The sender encrypts the message with the symmetric key.
- The sender encrypts the symmetric key with the receiver’s public key.
- The sender concatenates the encrypted symmetric key and the encrypted message.
- The sender transmits the encrypted message to the receiver.
Decryption
After the receiver gets the message, they will reverse the process:
1. The receiver extracts the encrypted symmetric key from the message.
2. The receiver decrypts the symmetric key using their private key.
3. The receiver decrypts the message with the symmetric key.
Programming the Solution in PHP
To demonstrate this technique we will use the PHP Secure Communications Library. This library (phpseclib) contains free and open source pure-PHP implementations of arbitrary-precision integers, RSA, DES, 3DES, RC4, Rijndael, AES, SSH-1, SSH-2, and SFTP.
The library is designed in such a way that it takes advantage of faster encryption functions (such as open_ssl and mcrypt), if they are available, for performance purposes. However, sometimes, these libraries and functions are not available to PHP programmers (in a shared hosting environment for example). So, phpseclib is designed to work reliably, albeit more slowly, if only PHP is available.
You can install it via Composer.
Documentation for phpseclib can be found at http://phpseclib.sourceforge.net/documentation/index.html
Sample Code
Let’s write our demo code step by step.
Generate Public and Private Keys
The RSA class contains a key generation method. In the example below, you see how we generate and save the key pair. In an actual production environment, the private key would be stored in a safe location until it was time to decrypt the message. The public key would be transmitted to the sender of the message.
$rsa = new Crypt_RSA();
$keys = $rsa->createKey(2048);
file_put_contents('key.pri',$keys['privatekey']);
file_put_contents('key.pub',$keys['publickey']);
Encrypt Function
The encrypt_message function takes three parameters:
1. $plaintext
– The plaintext message to be encrypted.
2. $asym_key
– This is the public key provided to the sender by the receiver
3. $key_length
– This is an arbitrary length key, but should be less than 240. The larger the number, the less likely a brute force attack is to succeed. This is an optional parameter.
function encrypt_message($plaintext,$asym_key,$key_length=150)
{
$rsa = new Crypt_RSA();
$rij = new Crypt_Rijndael();
// Generate Random Symmetric Key
$sym_key = crypt_random_string($key_length);
// Encrypt Message with new Symmetric Key
$rij->setKey($sym_key);
$ciphertext = $rij->encrypt($plaintext);
$ciphertext = base64_encode($ciphertext);
// Encrypted the Symmetric Key with the Asymmetric Key
$rsa->loadKey($asym_key);
$sym_key = $rsa->encrypt($sym_key);
// Base 64 encode the symmetric key for transport
$sym_key = base64_encode($sym_key);
$len = strlen($sym_key); // Get the length
$len = dechex($len); // The first 3 bytes of the message are the key length
$len = str_pad($len,3,'0',STR_PAD_LEFT); // Zero pad to be sure.
// Concatenate the length, the encrypted symmetric key, and the message
$message = $len.$sym_key.$ciphertext;
return $message;
}
Decrypt Function
The decrypt function takes two parameters:
1. $message
– The message to be decrypted
2. $asym_key
– The private key of the receiver.
function decrypt_message($message,$asym_key)
{
$rsa = new Crypt_RSA();
$rij = new Crypt_Rijndael();
// Extract the Symmetric Key
$len = substr($message,0,3);
$len = hexdec($len);
$sym_key = substr($message,0,$len);
//Extract the encrypted message
$message = substr($message,3);
$ciphertext = substr($message,$len);
$ciphertext = base64_decode($ciphertext);
// Decrypt the encrypted symmetric key
$rsa->loadKey($asym_key);
$sym_key = base64_decode($sym_key);
$sym_key = $rsa->decrypt($sym_key);
// Decrypt the message
$rij->setKey($sym_key);
$plaintext = $rij->decrypt($ciphertext);
return $plaintext;
}
Conclusion
As programs and web services become more connected, secure data communication between remote processes should become a routine. Since asymmetric keys can be transmitted securely, they are preferable. However, they have a limited message size. A hybrid approach, using both symmetric and asymmetric algorithms can address both of these limitations.
The PHP Secure Communications Library provides convenient, pure PHP implementations of symmetric and asymmetric industry standard algorithms. This provides us with the means of creating the hybrid solution in PHP.
The source code for this article is on github: https://github.com/sitepoint-editors/sitepointcrypto
Frequently Asked Questions (FAQs) about Asymmetric Keys and PHPseclib
What is the main difference between symmetric and asymmetric encryption?
Symmetric encryption uses the same key for both encryption and decryption. This means that the key must be shared between the sender and receiver, which can pose a security risk. On the other hand, asymmetric encryption uses two different keys: a public key for encryption and a private key for decryption. The public key can be freely distributed, while the private key remains secret. This eliminates the need for key exchange, making it more secure.
How does PHPseclib handle large messages with asymmetric keys?
PHPseclib uses a technique called “encryption with RSA and OAEP padding”. This method involves splitting the large message into smaller chunks, encrypting each chunk separately, and then combining them. This allows PHPseclib to handle messages of any size, even those larger than the key size.
What are the advantages of using PHPseclib for encryption?
PHPseclib is a pure-PHP implementation of many cryptographic operations, including encryption and decryption. It is highly portable and does not require any extensions or libraries. It also supports a wide range of encryption algorithms, including RSA, DES, and AES.
How secure is asymmetric encryption with PHPseclib?
Asymmetric encryption with PHPseclib is very secure, as long as the private key is kept secret. The public key can be freely distributed without compromising security. Furthermore, PHPseclib uses secure cryptographic algorithms and techniques, such as RSA and OAEP padding.
Can I use PHPseclib for symmetric encryption as well?
Yes, PHPseclib supports both symmetric and asymmetric encryption. It includes implementations of many symmetric encryption algorithms, such as DES, 3DES, and AES.
How can I choose the right key size for asymmetric encryption with PHPseclib?
The key size for asymmetric encryption depends on the level of security required. Larger keys provide more security but are slower to use. A key size of 2048 bits is generally considered a good balance between security and performance.
What is OAEP padding and why is it used in PHPseclib?
OAEP (Optimal Asymmetric Encryption Padding) is a padding scheme used in RSA encryption. It adds randomness to the encryption process, making it more secure against certain types of attacks. PHPseclib uses OAEP padding for RSA encryption to enhance security.
How can I ensure the security of my private key when using PHPseclib?
The security of your private key is crucial for the security of your encrypted data. You should store your private key in a secure location and protect it with a strong password. You should also limit access to the key as much as possible.
Can I use PHPseclib for other cryptographic operations besides encryption?
Yes, PHPseclib supports a wide range of cryptographic operations, including hashing, digital signatures, and key generation. It also includes functions for working with X.509 certificates and SSH-2 servers.
How can I get started with PHPseclib?
You can download PHPseclib from its official website or install it via Composer. The PHPseclib website also provides comprehensive documentation and examples to help you get started.
David Brumbaugh is CTO of SaaS provider 3B Alliance, LLC. He’s been involved in Internet development since 1994. His articles have been published by Dice, C/C++ User's Journal, PC Techniques and John Wiley & Sons. David specializes in software design in PHP/mySQL, HTML/Javascript, C++ and C#/SQL Server, focused on application security, eCommerce,and WordPress plugin development.