Simple JavaScript code snippet to remove the first element of an array. You can also remove the last element of the array just as easy. Just use the JavaScript array.shift() and array.pop() methods. These methods changes the length of the array!
var myarray = ["item 1", "item 2", "item 3", "item 4"];
//removes the first element of the array, and returns that element.
alert(myarray.shift());
//alerts "item 1"
//removes the last element of the array, and returns that element.
alert(myarray.pop());
//alerts "item 4"
Don’t forget to put your jQuery code inside a document ready function. See 4 different jQuery document ready examples for more info.
Frequently Asked Questions (FAQs) about jQuery Array Element Removal
How can I remove a specific element from an array in jQuery?
To remove a specific element from an array in jQuery, you can use the $.grep() method. This method is used to filter the elements of an array based on a certain condition. Here’s an example:var array = [1, 2, 3, 4, 5];
var removeItem = 3;
array = $.grep(array, function(value) {
return value != removeItem;
});
In this example, the $.grep() method is used to filter out the number 3 from the array. The resulting array will be [1, 2, 4, 5].
What is the difference between shift() and pop() methods in jQuery?
The shift() and pop() methods are used to remove elements from an array in jQuery. The shift() method removes the first element from an array and returns that removed element. This method changes the length of the array. On the other hand, the pop() method removes the last element from an array and returns that element. This method also changes the length of the array.
How can I remove multiple elements from an array in jQuery?
To remove multiple elements from an array in jQuery, you can use the splice() method. The splice() method changes the contents of an array by removing or replacing existing elements. Here’s an example:var array = [1, 2, 3, 4, 5];
array.splice(1, 2);
In this example, the splice() method removes two elements from the array starting from index 1. The resulting array will be [1, 4, 5].
How can I remove all elements from an array in jQuery?
To remove all elements from an array in jQuery, you can simply set the length of the array to 0. Here’s how you can do it:var array = [1, 2, 3, 4, 5];
array.length = 0;
After executing the above code, the array will be empty.
How can I remove duplicate elements from an array in jQuery?
To remove duplicate elements from an array in jQuery, you can use the $.unique() method. This method sorts an array of DOM elements, in place, with the duplicates removed. Note that this only works on arrays of DOM elements, not strings or numbers.
How can I remove an element from an array without changing the original array in jQuery?
To remove an element from an array without changing the original array in jQuery, you can use the filter() method. This method creates a new array with all elements that pass the test implemented by the provided function.
How can I remove an element from an array by its index in jQuery?
To remove an element from an array by its index in jQuery, you can use the splice() method. The first argument defines the location at which to begin adding or removing elements. The second argument defines the number of elements to remove. The splice() method returns an array with the deleted items.
How can I check if an array is empty in jQuery?
To check if an array is empty in jQuery, you can use the length property. If the length property returns 0, it means the array is empty.
How can I remove an element from an array and return the new array in jQuery?
To remove an element from an array and return the new array in jQuery, you can use the filter() method. This method creates a new array with all elements that pass the test implemented by the provided function.
How can I remove the first and last element from an array in jQuery?
To remove the first and last element from an array in jQuery, you can use the shift() and pop() methods respectively. The shift() method removes the first element from an array, and the pop() method removes the last element from an array.
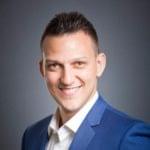
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.