jQuery code snippet to either display a default image for those that are broken or detect and hide all broken images. Hide all those red crosses! ;)
// Replace source
$('img').error(function(){
$(this).attr('src', 'missing.png');
});
// Or, hide them
$("img").error(function(){
$(this).hide();
});
Frequently Asked Questions on Detecting and Hiding Broken Images
How can I detect broken images on my website using jQuery?
Detecting broken images on your website using jQuery is quite simple. You can use the .error()
method which triggers when an error occurs while loading an external file. Here’s a simple code snippet that can help you detect broken images:$('img').error(function(){
alert('Broken Image Detected!');
});
This code will alert you whenever a broken image is detected on your website.
How can I hide broken images using jQuery?
Hiding broken images can be done using the same .error()
method in jQuery. Here’s how you can do it:$('img').error(function(){
$(this).hide();
});
This code will automatically hide any broken images on your website.
Can I replace broken images with a default image using jQuery?
Yes, you can replace broken images with a default image using jQuery. Here’s a simple code snippet:$('img').error(function(){
$(this).attr('src', 'default.jpg');
});
This code will replace any broken images with a default image named ‘default.jpg’.
How can I detect and hide broken images using only CSS/HTML?
While jQuery provides a more dynamic solution, you can also use CSS/HTML to hide broken images. Here’s a simple CSS solution:img {
font-size: 0;
}
img:after {
content: " ";
font-size: 16px;
}
This code will hide the broken image icon and replace it with a space.
How can I find broken images on my website?
There are several ways to find broken images on your website. You can manually check each image, use a website crawler tool, or use a jQuery script to detect broken images.
What causes broken images on a website?
Broken images can be caused by several factors including incorrect image URLs, the image file being moved or deleted, or issues with the server hosting the image.
How can I prevent broken images on my website?
To prevent broken images, ensure that all image URLs are correct, avoid moving or deleting image files after they have been linked, and ensure your server is running smoothly.
Can broken images affect my website’s SEO?
Yes, broken images can negatively impact your website’s SEO as they can lead to a poor user experience. Search engines like Google prioritize websites that provide a good user experience.
How can I fix broken images on my website?
Fixing broken images involves identifying the cause of the issue and then resolving it. This could involve correcting the image URL, replacing the image file, or resolving server issues.
Can I use JavaScript instead of jQuery to detect and hide broken images?
Yes, you can use JavaScript to detect and hide broken images. However, the process is a bit more complex compared to using jQuery.
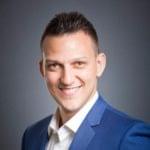
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.