$(document).ready(function() {
//jquery
$(location).attr('href');
//pure javascript
var pathname = window.location.pathname;
// to show it in an alert window
alert(window.location);
});
There is also this function that may help when determining absolute paths.
function getAbsolutePath() {
var loc = window.location;
var pathName = loc.pathname.substring(0, loc.pathname.lastIndexOf('/') + 1);
return loc.href.substring(0, loc.href.length - ((loc.pathname + loc.search + loc.hash).length - pathName.length));
}
Frequently Asked Questions (FAQs) about jQuery Current Page URL
How can I get the current URL in jQuery?
To get the current URL in jQuery, you can use the window.location.href
property. This property returns the URL of the current page. Here is a simple example:$(document).ready(function(){
var currentURL = window.location.href;
console.log(currentURL);
});
In this code, window.location.href
gets the current URL and stores it in the currentURL
variable. The console.log(currentURL)
line then prints this URL to the console.
Can I get the current URL without the query string in jQuery?
Yes, you can get the current URL without the query string by using the window.location.origin
and window.location.pathname
properties. Here is an example:$(document).ready(function(){
var currentURL = window.location.origin + window.location.pathname;
console.log(currentURL);
});
In this code, window.location.origin
gets the origin (protocol, hostname, and port) of the URL, and window.location.pathname
gets the pathname. The +
operator concatenates these two parts to form the URL without the query string.
How can I get the query string from the current URL in jQuery?
You can get the query string from the current URL by using the window.location.search
property. This property returns the query string, including the leading question mark. Here is an example:$(document).ready(function(){
var queryString = window.location.search;
console.log(queryString);
});
In this code, window.location.search
gets the query string and stores it in the queryString
variable. The console.log(queryString)
line then prints this query string to the console.
Can I get the current URL in jQuery without the hash?
Yes, you can get the current URL without the hash by using the window.location.href
property and the split()
method. Here is an example:$(document).ready(function(){
var currentURL = window.location.href.split('#')[0];
console.log(currentURL);
});
In this code, window.location.href.split('#')[0]
gets the current URL and splits it at the hash (#
). The [0]
part then gets the first part of the split URL, which is the URL without the hash.
How can I get the hash from the current URL in jQuery?
You can get the hash from the current URL by using the window.location.hash
property. This property returns the hash, including the leading hash sign. Here is an example:$(document).ready(function(){
var hash = window.location.hash;
console.log(hash);
});
In this code, window.location.hash
gets the hash and stores it in the hash
variable. The console.log(hash)
line then prints this hash to the console.
Can I change the current URL in jQuery without reloading the page?
Yes, you can change the current URL in jQuery without reloading the page by using the history.pushState()
method. This method pushes a new entry into the history stack. Here is an example:$(document).ready(function(){
history.pushState(null, null, '/new-page.html');
});
In this code, history.pushState(null, null, '/new-page.html')
changes the current URL to ‘/new-page.html’ without reloading the page.
How can I get the protocol of the current URL in jQuery?
You can get the protocol of the current URL by using the window.location.protocol
property. This property returns the protocol of the URL, including the trailing colon. Here is an example:$(document).ready(function(){
var protocol = window.location.protocol;
console.log(protocol);
});
In this code, window.location.protocol
gets the protocol and stores it in the protocol
variable. The console.log(protocol)
line then prints this protocol to the console.
How can I get the hostname of the current URL in jQuery?
You can get the hostname of the current URL by using the window.location.hostname
property. This property returns the hostname of the URL. Here is an example:$(document).ready(function(){
var hostname = window.location.hostname;
console.log(hostname);
});
In this code, window.location.hostname
gets the hostname and stores it in the hostname
variable. The console.log(hostname)
line then prints this hostname to the console.
How can I get the port of the current URL in jQuery?
You can get the port of the current URL by using the window.location.port
property. This property returns the port of the URL. Here is an example:$(document).ready(function(){
var port = window.location.port;
console.log(port);
});
In this code, window.location.port
gets the port and stores it in the port
variable. The console.log(port)
line then prints this port to the console.
How can I get the pathname of the current URL in jQuery?
You can get the pathname of the current URL by using the window.location.pathname
property. This property returns the pathname of the URL. Here is an example:$(document).ready(function(){
var pathname = window.location.pathname;
console.log(pathname);
});
In this code, window.location.pathname
gets the pathname and stores it in the pathname
variable. The console.log(pathname)
line then prints this pathname to the console.
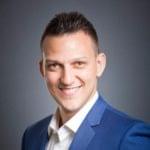
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.