Here is some quick JavaScript code snippets to find out and log the current function name in JavaScript.
//way 1
var fName = arguments.callee.toString();
console.log(fName);
fName = fName.substr('function '.length);
console.log(fName);
fName = fName.substr(0, fName.indexOf('('));
console.log(fName);
//way 2
var fName = arguments.callee.toString().match(/functions+([^s(]+)/);
console.log(fName);
console.log(fName[1]);
//way 3
var fName = arguments.callee.toString(),
regEx = /^functions+([^(]+)/
console.log(regEx.exec(fName)[1]);
Does anyone know of a way to get the function name for a object literal syntax function?? I don’t think it’s possible…
Frequently Asked Questions (FAQs) about jQuery Console Log and Current Function
What is the purpose of using console.log in jQuery?
The console.log() function in jQuery is a powerful debugging tool that developers use to print output or log information from JavaScript code to the console. This function is particularly useful for tracking variables, displaying error messages, or understanding the flow of code execution. It helps developers identify and fix issues in their code more efficiently.
How can I get the name of the currently running function in JavaScript?
In JavaScript, you can get the name of the currently running function using the ‘arguments.callee’ property. This property refers to the currently executing function. To get the function name, you can use ‘arguments.callee.name’. However, please note that this method is not recommended for strict mode in ECMAScript 5 and is removed in ECMAScript 6.
What is the use of scrollTop in jQuery?
The scrollTop() method in jQuery is used to get or set the vertical scrollbar position for the selected elements. When used as a setter, it scrolls the selected elements to the specified position. When used as a getter, it returns the current vertical position of the first matched element’s scroll bar.
How can I debug jQuery using the console?
Debugging jQuery using the console involves using various console methods like console.log(), console.error(), console.warn(), and console.info(). These methods allow you to log information related to your code’s execution in the console, helping you identify and fix any issues or bugs.
What is the difference between console.log and console.error in jQuery?
Both console.log and console.error are used to display messages in the console. However, console.log is used for general output of logging information, while console.error is used specifically to output error messages. Messages outputted with console.error are styled differently in the console to make them stand out.
How can I use console.log to track variables in jQuery?
To track variables using console.log, you simply need to pass the variable as an argument to the console.log function. For example, if you have a variable ‘x’, you can track its value by using ‘console.log(x);’. This will print the current value of ‘x’ to the console.
Can I use console.log in production code?
While you can use console.log in production code, it is generally not recommended. Console.log is primarily a debugging tool and leaving it in production code can lead to performance issues and potentially expose sensitive information.
How can I clear the console in jQuery?
You can clear the console in jQuery using the console.clear() method. This method clears the console of any previous logs, making it easier to read new logs.
Can I use console.log to log more than one variable at a time?
Yes, you can log multiple variables at once using console.log. Simply pass the variables as arguments separated by commas. For example, ‘console.log(x, y, z);’ will log the values of ‘x’, ‘y’, and ‘z’ to the console.
What is the difference between console.log and document.write in jQuery?
Console.log and document.write are both used to output information, but they do so in different ways. Console.log outputs information to the browser’s console, which is typically used for debugging purposes. Document.write, on the other hand, writes information directly to the HTML document, which can be seen by the user.
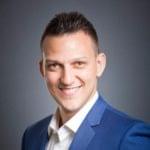
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.