Hello, Laravel? Communicating with PHP through SMS!

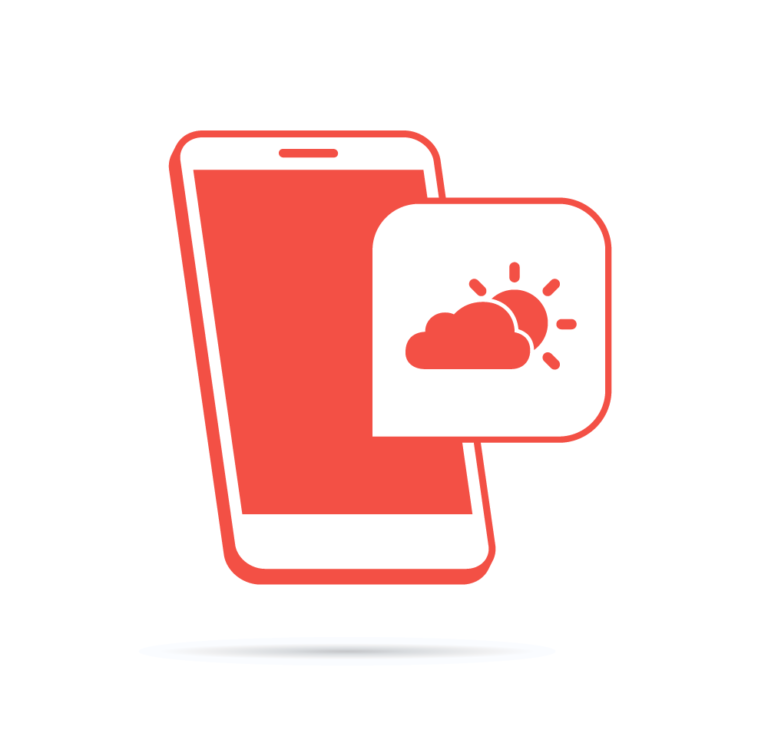
Key Takeaways
- The Laravel-powered weather forecast app can be modified to be accessible via SMS in addition to the voice telephone system, by adding routes and modifying the service layer to account for SMS communication.
- In the controller, when an SMS message comes in from a user, Twilio will always hit the same route, going through the showWeather method. The parseBody method is used to evaluate the user’s input and determine their intent, such as checking the weather for a specific day or requesting credits.
- The application created over the course of two articles demonstrates how to interact with users via the voice telephone system using voice menus and SMS, using Laravel for the application backend and Twilio for the telephone/SMS integration. The functionality of the voice app can be extended to users via SMS with additional code.
This article was peer reviewed by Wern Ancheta. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
In this article, we will modify our Laravel-powered phone-capable weather forecast app so that it is accessible via SMS (text message) in addition to the voice telephone system. It is recommended you read the previous post if you haven’t done so yet – it’s a 10 minute read for an excellent outcome.
Note: If you’re confused by the development environment we’re using, it’s Homestead Improved and you can learn more about it here, or go in detail by buying our book about PHP environments.
Adding Routes
To allow for SMS communication, we need some more routes. Open up the routes/web.php
file and append the following code to it:
Route::group(['prefix' => 'sms', 'middleware' => 'twilio'], function () {
Route::post('weather', 'SmsController@showWeather')->name('weather');
});
The prefix for the route is sms
, so that routes will have a path like /sms/weather
, as the one in the example. This is the only route we need for SMS, as Twilio will call the same route over and over again. Twilio will access it via HTTP POST
. We could also do this without the prefix, but it’s more flexible this way if we decide to add more functionality to the SMS side later.
Service Layer
Next, we’ll modify the service we wrote previously. Open up the app/Services/WeatherService.php
file and remove the current getWeather
method, then replace it with the one below:
public function getWeather($zip, $dayName, $forSms = false)
{
$point = $this->getPoint($zip);
$tz = $this->getTimeZone($point);
$forecast = $this->retrieveNwsData($zip);
$ts = $this->getTimestamp($dayName, $zip);
$tzObj = new \DateTimeZone($tz->timezoneId);
$tsObj = new \DateTime(null, $tzObj);
$tsObj->setTimestamp($ts);
foreach ($forecast->properties->periods as $k => $period) {
$startTs = strtotime($period->startTime);
$endTs = strtotime($period->endTime);
if ($ts > $startTs and $ts < $endTs) {
$day = $period;
break;
}
}
$weather = $day->name;
$weather .= ' the ' . $tsObj->format('jS') . ': ';
$response = new Twiml();
if ($forSms) {
$remainingChars = 140 - strlen($weather);
if (strlen($day->detailedForecast) > $remainingChars) {
$weather .= $day->shortForecast;
$weather .= '. High of ' . $day->temperature . '. ';
$weather .= $day->windDirection;
$weather .= ' winds of ' . $day->windSpeed;
} else {
$weather .= $day->detailedForecast;
}
$response->message($weather);
} else {
$weather .= $day->detailedForecast;
$gather = $response->gather(
[
'numDigits' => 1,
'action' => route('day-weather', [], false)
]
);
$menuText = ' ';
$menuText .= "Press 1 for Sunday, 2 for Monday, 3 for Tuesday, ";
$menuText .= "4 for Wednesday, 5 for Thursday, 6 for Friday, ";
$menuText .= "7 for Saturday. Press 8 for the credits. ";
$menuText .= "Press 9 to enter in a new zipcode. ";
$menuText .= "Press 0 to hang up.";
$gather->say($weather . $menuText);
}
return $response;
}
This function is very similar to the old one. The only difference is that it takes into consideration that the weather request might be coming form a telephone device via SMS, so it makes sure that the weather forecast isn’t too long and tries to limit it to less than 140 characters. The response for SMS is still TwiML, just formatted for SMS.
Controller
Create a file called SmsController.php
in the app/Http/Controllers
folder and put the following code into it:
<?php
namespace App\Http\Controllers;
use App\Services\WeatherService;
use Illuminate\Http\Request;
use Twilio\Twiml;
class SmsController extends Controller
{
protected $weather;
public function __construct(WeatherService $weatherService)
{
$this->weather = $weatherService;
}
public function showWeather(Request $request)
{
$parts = $this->parseBody($request);
switch ($parts['command']) {
case 'zipcode':
$zip = $parts['data'];
$request->session()->put('zipcode', $zip);
$response = $this->weather->getWeather($zip, 'Today', true);
break;
case 'day':
$zip = $request->session()->get('zipcode');
$response = $this->weather->getWeather($zip, $parts['data'], true);
break;
case 'credits':
$response = new Twiml();
$response->message($this->weather->getCredits());
break;
default:
$response = new Twiml();
$text = 'Type in a zipcode to get the current weather. ';
$text .= 'After that, you can type the day of the week to get that weather.';
$response->message($text);
break;
}
return $response;
}
private function parseBody($request)
{
$ret = ['command' => ''];
$body = trim($request->input('Body'));
if (is_numeric($body) and strlen($body) == 5) {
$ret['command'] = 'zipcode';
$ret['data'] = $body;
}
if (in_array(ucfirst(strtolower($body)), $this->weather->daysOfWeek) !== false) {
$ret['command'] = 'day';
$ret['data'] = ucfirst(strtolower($body));
}
if (strtolower($body) == 'credits') {
$ret['command'] = 'credits';
}
return $ret;
}
}
When an SMS message comes in from a user, Twilio will always hit the same route. This app does not have any redirects. That is why we only defined one route meaning all the requests will be going through the showWeather
method. There are different things a user can text the app, so we will parse the request body to figure out what they want using the parseBody
method.
The parseBody
method first creates a default return value. Then, it strips whitespace. This is so that if a user inputs “90210 ” (note the space), the program will still work as intended. Once the whitespace has been stripped, the body of the text is evaluated against three if
statements. The first if
statement checks to see if the user entered a zipcode. The second if
statement checks to see if the user entered in a day of the week. It normalizes the input by making sure that only the first letter is capitalized, and compares it to the contents of the $daysOfWeek
array property in the WeatherService
class to determine if a day of the week was mentioned. The last if
statement checks if a user requested the credits. If none of the three if
statements evaluate to true
then the program cannot figure out what the user wants and will return the default value. This default value will make the weather
method send the user a help message that explains how to use the app.
The parseBody
method returns an array with two keys in it. The command
key is what the user’s intention was determined to be. The data
key is the data that goes with the command. Inside the showWeather
method, after the parsebody
is called, a switch
statement is used to look at the value of the command
array key.
If the parser determines a user texted a zipcode, then we store the zipcode in a session and return today’s forecast for that zipcode. A sample TwiML response looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Message>This Afternoon the 31st: Sunny, with a high near 72. South southwest wind around 8 mph.
</Message>
</Response>
If it is determined a day of the week was entered, then that day’s forecast is returned. A sample TwiML response looks like this:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Message>Monday the 3rd: Sunny, with a high near 70.
</Message>
</Response>
If the parser determines the credits were asked for, then the app returns a TwiML response with the credits:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Message>Weather data provided by the National Weather Service. Zipcode data provided by GeoNames.
</Message>
</Response>
If the parser cannot determine the user’s intent, then a help message is returned with this TwiML:
<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Message>Type in a zipcode to get the current weather. After that, you can type the day of the week to get that weather.
</Message>
</Response>
Twilio
Login to your Twilio account and navigate to the settings for your phone number. You can see your number by going to this page. In the SMS section, put in the URL in the following format: http://NGROK_HOST/sms/weather
, where NGROK_HOST
is the hostname in the URL you noted from the Ngrok program.
Using the App
Open up the text messaging app on your phone and send a zipcode like 92010
to your Twilio phone number. In a couple of seconds, you should get a response with today’s forecast.
Next, you can send a day of the week to the number and it will respond with that day’s forecast.
You can also send the word credits
and it will return the credits.
If you enter in a command the weather app does not understand, it returns some help text.
Conclusion
Over the course of two articles, we have seen how to build an application that is able to interact with users via the voice telephone system using voice menus and to interact with them using SMS. This was implemented using Laravel for the application backend and Twilio for the telephone / SMS integration. With writing a little bit more code, we have seen that it is possible to extend the voice app to have the same functionality exposed to users via SMS.
You can find the example code for this article series on Github.
There are lots of possibilities for apps that you can implement with Twilio and PHP, this is just a little glimpse into what can be done. Check out the documentation here for some inspiration.
Frequently Asked Questions (FAQs) about Laravel PHP SMS Communication
How can I send an SMS using Laravel PHP?
Laravel PHP provides a simple and efficient way to send SMS messages. You can use the Nexmo SMS API, which is a popular choice among developers. First, you need to install the Nexmo client library for PHP using composer. Then, you can create a new instance of the Nexmo client, passing in your API key and secret. You can then use the ‘send’ method to send an SMS, specifying the recipient’s number, your Nexmo number, and the message text.
What are the prerequisites for sending SMS using Laravel PHP?
Before you can send SMS messages using Laravel PHP, you need to have a few things set up. First, you need to have Laravel installed on your server. You also need to have a Nexmo account, as this is the service that Laravel uses to send SMS messages. Finally, you need to have the Nexmo client library for PHP installed.
Can I send SMS messages to multiple recipients using Laravel PHP?
Yes, you can send SMS messages to multiple recipients using Laravel PHP. You can do this by passing an array of phone numbers to the ‘send’ method instead of a single number. Each number in the array will receive the same message.
How can I handle errors when sending SMS using Laravel PHP?
When sending SMS messages using Laravel PHP, errors can be handled using try-catch blocks. If an error occurs while sending the message, an exception will be thrown. You can catch this exception and handle it appropriately, such as by logging the error or displaying an error message to the user.
Can I send SMS messages internationally using Laravel PHP?
Yes, you can send SMS messages internationally using Laravel PHP. The Nexmo SMS API supports international messaging, so you can send messages to recipients in different countries. However, you should be aware that international messaging may incur additional costs.
How can I schedule SMS messages using Laravel PHP?
Laravel PHP does not natively support scheduling SMS messages. However, you can achieve this by using Laravel’s task scheduling feature. You can create a scheduled task that sends an SMS message at a specific time.
Can I receive SMS messages using Laravel PHP?
Yes, you can receive SMS messages using Laravel PHP. You can do this by setting up a webhook on your Nexmo account. When an SMS message is received, Nexmo will send a request to your webhook, and you can handle this request in your Laravel application.
How can I track the delivery status of SMS messages using Laravel PHP?
You can track the delivery status of SMS messages using Laravel PHP by setting up a delivery receipt webhook on your Nexmo account. When the status of an SMS message changes, Nexmo will send a request to your webhook, and you can handle this request in your Laravel application.
How secure is sending SMS messages using Laravel PHP?
Sending SMS messages using Laravel PHP is secure, as the Nexmo SMS API uses HTTPS for all requests. This means that the data sent between your application and Nexmo is encrypted and cannot be intercepted.
Can I send MMS messages using Laravel PHP?
Currently, the Nexmo SMS API does not support sending MMS messages. However, you can send SMS messages with links to media files, which is a common workaround for this limitation.
Chris is an App Development Manager for one of the top medical schools in Southern California. He has contributed to a few open source PHP projects.
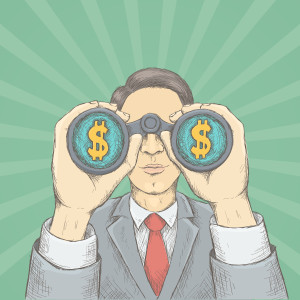
Published in
·APIs·CMS & Frameworks·E-Commerce·Frameworks·Laravel·Libraries·PHP·Web Services·January 17, 2017
Published in
·Development Environment·Extensions·Miscellaneous·Patterns & Practices·PHP·Programming·January 7, 2015
Published in
·APIs·Authentication·Frameworks·Laravel·Libraries·Patterns & Practices·PHP·Security·Standards·February 17, 2016