Simple JavaScript code snippets to delare an array. Like other JavaScript variables, you do not have to declare arrays before you can use them. I like to declare them just so I can easily read whats going on, it’s a good practice!
Example 1 – Array Constructor
// Declare an array (using the array constructor)
var arlene1 = new Array();
var arlene2 = new Array("First element", "Second", "Last");
Example 2 – Literal notatoin
// Declare an array (using literal notation)
var arlene1 = [];
var arlene2 = ["First element", "Second", "Last"];
Example 3 – Implicit Declaration
// Create an array from a method's return value
var carter = "I-learn-JavaScript";
var arlene3 = carter.split("-");
To avoid script errors, you should get into the habit of initializing an array when you declare it, like so:
// Declare an empty array using literal notation:
var arlene = [];
// The variable now contains an array of length zero
After checking my code on jslint.com I found out it says declaring an array with an array constructor is seen as bad practise! It suggests using literal notation.
FAQs on Declaring and Initializing Arrays in jQuery and JavaScript
What is the basic syntax for declaring an array in jQuery?
In jQuery, arrays are declared in a similar way to how they are declared in JavaScript. The basic syntax for declaring an array in jQuery is as follows:var arrayName = [];
In this syntax, “arrayName” is the name of the array. The square brackets denote an empty array. You can also initialize the array with values at the time of declaration like this:var arrayName = [value1, value2, value3];
In this syntax, “value1”, “value2”, and “value3” are the initial values of the array.
How can I add elements to a jQuery array?
You can add elements to a jQuery array using the push() method. Here is an example:var arrayName = [];
arrayName.push(value);
In this example, “value” is the value you want to add to the array. The push() method adds the value to the end of the array.
How can I remove elements from a jQuery array?
You can remove elements from a jQuery array using the splice() method. Here is an example:var arrayName = [value1, value2, value3];
arrayName.splice(index, 1);
In this example, “index” is the index of the element you want to remove. The second parameter of the splice() method is the number of elements you want to remove.
How can I access elements in a jQuery array?
You can access elements in a jQuery array using their index. Here is an example:var arrayName = [value1, value2, value3];
var value = arrayName[index];
In this example, “index” is the index of the element you want to access. Array indices start at 0, so the first element of the array is at index 0.
How can I find the length of a jQuery array?
You can find the length of a jQuery array using the length property. Here is an example:var arrayName = [value1, value2, value3];
var length = arrayName.length;
In this example, the length property returns the number of elements in the array.
How can I iterate over a jQuery array?
You can iterate over a jQuery array using a for loop or the jQuery each() function. Here is an example using a for loop:var arrayName = [value1, value2, value3];
for (var i = 0; i < arrayName.length; i++) {
console.log(arrayName[i]);
}
In this example, the for loop iterates over each element in the array and logs it to the console.
How can I sort a jQuery array?
You can sort a jQuery array using the sort() method. Here is an example:var arrayName = [value3, value1, value2];
arrayName.sort();
In this example, the sort() method sorts the elements of the array in ascending order.
How can I reverse a jQuery array?
You can reverse a jQuery array using the reverse() method. Here is an example:var arrayName = [value1, value2, value3];
arrayName.reverse();
In this example, the reverse() method reverses the order of the elements in the array.
How can I join the elements of a jQuery array into a string?
You can join the elements of a jQuery array into a string using the join() method. Here is an example:var arrayName = [value1, value2, value3];
var string = arrayName.join(separator);
In this example, “separator” is the string to use between each element in the resulting string.
How can I check if a value exists in a jQuery array?
You can check if a value exists in a jQuery array using the indexOf() method. Here is an example:var arrayName = [value1, value2, value3];
var index = arrayName.indexOf(value);
In this example, “value” is the value you want to check for. The indexOf() method returns the index of the first occurrence of the value in the array, or -1 if the value is not found.
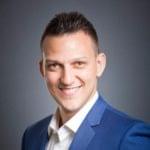
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.