Using HTML5 Video and Audio in Modern Browsers
Key Takeaways
- HTML5 video and audio tags allow developers to embed media directly into webpages without the need for third-party plugins like QuickTime, Flash or Silverlight, saving development time.
- Benefits of using HTML5 video and audio tags include hardware acceleration, which uses less CPU power and improves website responsiveness, and a plug-in free experience, eliminating the need for users to download the right plug-in.
- To ensure compatibility across different browsers, multiple video or audio sources can be added. The browser will work its way through the list until it finds a source it can play.
- The video and audio tags can be controlled programmatically through JavaScript, providing developers with the ability to create custom controls such as play, pause, and volume adjustment.
Video and audio tags provide the developer with a rich UI without having to install third-party plug-ins like QuickTime, Flash or Silverlight. This is because these tags are embedded directly into the webpage.
This in turn is a big deal because it can save you plenty of development time. Modern browsers like Chrome, Firefox, IE9 (and newcomer IE10) support a multitude of video and audio formats.
There are two big benefits to using video and audio tags:
- Hardware acceleration. Playing a video in a hardware accelerated browser will use significantly less CPU power than it would in another browser. If you’re controlling these tags in JavaScript, this rendering can be offloaded to the GPU which helps in the responsiveness of the website. This is important if you want the playback to be streamlined.
- Plug-in free. You don’t have to worry about users downloading the right plug-in or the complexities in supporting many of them.
If you’re unfamiliar with HTML5, before diving into this article you might like to read Yes, You Can Use HTML5 Today! and HTML5 and Even Fancier Forms.
Markup for Video
The video tag is awesome because it gives you the power to embed video content straight into the webpage without needing a third-party plug-in. To begin with, let’s take a look at the video tag and I’ll explain each attribute.
<video width="320"
src="intro.mp4"
height="240"
poster="intro.jpg"
autoplay
controls
loop
autobuffer
>
This content appears if the video tag or the codec is not supported.
</video>
Here’s the breakdown of the attributes:
- width – sets the width of the video element in pixels. If the width is omitted, the browser will use the default width of the video, if it’s available.
- height – sets the height of the video element. If the height is omitted, the browser will use the default height of the video, if it’s available.
- src – sets the video file to be played. For reach, you should supply video formats that are supported by the most popular browsers.
- poster – sets the image file that will be displayed while the video content is being loaded, or until the user plays the video. If a poster file is omitted, the browser will show the first frame of the video.
- autoplay – instructs the browser to automatically play the video when the page is loaded.
- controls – displays the video controls to control the video playback. The controls are visible when the user hovers over a video. It’s also possible to tab through the controls.
- loop – instructs the browser to loop the media playback.
- autobuffer – used when autoplay is not used. The video is downloaded in the background, so when the user does decide to watch the video, it starts immediately.
Let’s use IE9 as an example to represent modern browsers. They’ll see the video playing:
If the user is using an old browser, for example IE6, 7 or 8, they’ll see this message.
The reason earlier versions of IE display this message is simple: they don’t support the video tag. But what happens if the browser doesn’t support the video you’re trying to play? To work around this issue you simply add multiple video sources. The video tag supports child source elements. Here’s what HTML looks like.
<video width="320"
height="240"
poster="intro.jpg"
autoplay
controls
loop>
This content appears if the video tag or the codec is not supported.
<source src="intro.mp4" type="video/mp4" />
<source src="intro.webm" type="video/webm" />
<source src="intro.ogv" type="video/ogg" />
</video>
By adding multiple sources, the browser will work its way through the list from top to bottom until it finds a video source it can play. Here’s a breakdown of the attributes.
- src – sets the video file to be played. For reach, you should supply video formats that are supported by the most popular browsers.
- type – tells the browser what kind of container format is used.
It’s important to note the type attribute. If this is omitted, the browser needs to download a small piece of each file to work out if it’s supported or not. This is a complete waste of bandwidth and totally unnecessary.
In the code above we’ve removed the src attribute and replaced it with three source child elements. The main reason for supplying multiple video sources is reach. Not all browsers support the same codecs, so it’s important to create a video file and encode it with the correct codec that your target browser supports.
In this case, a modern browser would pick the first source and play its associated source file. Also in the code above, the video will automatically play when the page is loaded. This might not sit well with some of your users, so it’s a good option to turn this off and allow them to start the video when they want to. To give them this ability we use JavaScript.
The video element supports play and pause methods, which gives you the ability to programmatically control the video. The following example does just that.
<script type="text/javascript">
var vid = function() {
return {
play: function() {
var v = document.getElementById("myVideo");
v.play();
},
pause: function() {
var v = document.getElementById("myVideo");
v.pause();
}
}
} ();
</script>
<body>
<h1>Video and legacy browser fallback</h1>
<video width="320"
height="240"
poster="intro.jpg"
id="myVideo">
This content appears if the video tag or the codec is not supported.
<source src="intro.mp4" type="video/mp4" />
<source src="intro.webm" type="video/webm" />
<source src="intro.ogv" type="video/ogg" />
</video>
<br />
<button onclick="vid.play();">Play</button>
<button onclick="vid.pause();">Pause</button>
</body>
Here’s how the webpage will look with the play and pause buttons.
This is the best option as it gives the user the power to start the video playback when they decide. You can even turn the volume up or down by using the volume method. Here’s how to do that.
<script type="text/javascript">
var vid = function() {
return {
increase: function() {
var v = document.getElementById("myVideo");
v.volume += 0.2;
},
decrease: function() {
var v = document.getElementById("myVideo");
v.volume -= 0.2;
}
}
} ();
</script>
<body>
<h1>Video and legacy browser fallback</h1>
<video width="320"
height="240"
poster="intro.jpg"
id="myVideo"
>
This content appears if the video tag or the codec is not supported.
<source src="intro.mp4" type="video/mp4" />
<source src="intro.webm" type="video/webm" />
<source src="intro.ogv" type="video/ogg" />
</video>
<br />
<button onclick="vid.increase();">Volumne Up</button>
<button onclick="vid.decrease();">Volumne Down</button>
</body>
A complete list of all the methods and events you can use with the video tag can be found here.
Multiple Video Formats
At the end of the day, you need to have the correct video codec for the browser, otherwise the video won’t play. It doesn’t get much simpler than that. The hard thing to get your head around is which format is supported by which browser. Here are the popular video formats:
Because the HTML 5 specification is unfinished, the video codecs mentioned above may expand or decrease in size. Early drafts of the specification mandated that browsers should have built-in support for multimedia in two codecs; Ogg Vorbis for audio and Ogg Theora for video. The result is that the specification makes no reference to what codecs should be supported by the browser.
Google announced they’re open-sourcing a video codec called VP8. IE9 supports this codec if it’s installed. If you’d like to install VP8 for IE9, you can download it here.
Markup for Audio
Like the video tag, the audio tag is fantastic because it gives you the power to embed audio content into the webpage without needing a third-party plug-in. Let’s take a look at the audio tag and I’ll explain each attribute.
<audio src="elvis.mp3"
preload="auto"
controls
autoplay
loop
autobuffer
muted
crossorigin
>
This content appears if the audio tag or the codec is not supported.
</audio>
Here’s the breakdown of the attributes:
- src – sets the audio file to be played. For reach, you should supply audio formats that are supported by the most popular browsers
- preload – none / metadata / auto – where metadata means preload just the metadata and auto leaves the browser to decide whether to preload the whole file
- controls – displays the video controls to control the video playback. The controls are visible when the user hovers over a video. It’s also possible to tab through the controls
- autoplay – instructs the browser to automatically play the video when the page is loaded
- loop – instructs the browser to loop the media playback.
- autobuffer – used when autoplay is not used. The video is downloaded in the background, so when the user does decide to watch the video, it starts immediately.
- muted – sets the default audio output.
- crossorigin – a CORS settings attribute.
If the user is using a modern browser, they’ll hear the audio playing.
If the user is using an old browser, for example IE6, 7 or 8, they’ll see the following comments.
If the browser supports the audio tag, but not the audio codec, the user will see the following output.
To work around these issues you simply add multiple audio sources. The audio tag supports child source elements. Here’s what HTML looks like.
<audio preload="auto"
loop
autobuffer
controls
>
This content appears if the audio tag or the codec is not supported.
<source src="elvis.ogg" type="audio/ogg" />
<source src="elvis.mp3" type="audio/mpeg" />
</audio>
By adding multiple sources, the browser will work its way through the list from top to bottom until it finds an audio source it can play. Here’s a breakdown of the attributes.
- src – sets the audio file to be played. For reach, you should supply audio formats that are supported by the most popular browsers.
- type – tells the browser what kind of container format is used.
As with the video tag, it’s important to specify the type attribute. If this is omitted, the browser needs to download a small piece of each file to work out if it’s supported or not. The audio tag will run from the top source until it finds an audio file it can play.
The audio tag is available through JavaScript, so it’s possible to create some more aesthetic buttons to allow the user to play and pause the audio. For this example I’m using border-radius that’s available in CSS3 to give me some rounded div tags. Here’s the code.
<head>
<style type="text/css">
div
{
height: 1.5em;
width: 5em;
-moz-border-radius: 15px;
border-radius: 15px;
font-family: verdana;
font-size: 0.8em;
background-color: #e0e0e0;
text-align: center;
text-shadow: 0 -1px 1px #222;
float: left;
}
</style>
<script type="text/javascript">
var aud = function () {
return {
play: function () {
var v = document.getElementById("myAudio");
v.play();
},
pause: function () {
var v = document.getElementById("myAudio");
v.pause();
},
init: function () {
var play = document.getElementById("play");
var pause = document.getElementById("pause");
play.addEventListener("click", aud.play);
pause.addEventListener("click", aud.pause);
}
}
} ();
window.onload = aud.init;
</script>
</head>
<body>
<h1>
Audio and legacy browser fallback</h1>
<audio preload="auto"
loop
autobuffer
id="myAudio">
This content appears if the audio tag or the codec is not supported.
<source src="elvis.ogg" type="audio/ogg">
<source src="elvis.mp3" type="audio/mpeg">
</audio>
<div id="play">
Play
</div>
<div id="pause">
Pause
</div>
</body>
</html>
And the rounded play and pause buttons can be seen below.
I’ve shown you only two functions available through JavaScript. Rest assured there are many more for your to work with through JavaScript. A full list of them can be found here.
Supported Formats
The audio tag is only as good as the files you supply, so you need to be aware of which codecs are supported. Here are the popular audio formats:
This tutorial has been made possible by the support of Microsoft. In cooperation with Microsoft and independently written by SitePoint, we strive to work together to develop the content that’s most useful and relevant to you.
It’s important to remember that because the HTML5 specification is a work in progress, the audio codecs mentioned above may expand or decrease over time, but it’s a safe bet MP3 will be there when the specification is finally completed.
Further Reading
To learn more about HTML5 video and audio, check out these links:
- Unlocking the Power of HTML5 <audio>
- 5 Things You Need to Know to Start Using <video> and <audio> Today
- HTML5 Guide for Developers – video and audio Elements
Frequently Asked Questions about Using HTML5 Video and Audio in Modern Browsers
How can I make my HTML5 video autoplay when the page loads?
To make your HTML5 video autoplay when the page loads, you need to add the ‘autoplay’ attribute to the ‘video’ tag. This attribute doesn’t need a value, its presence in the tag will enable the autoplay feature. Here’s an example:<video src="myvideo.mp4" autoplay></video>
Please note that some browsers or user settings may prevent videos from autoplaying to improve user experience or save data.
How can I loop my HTML5 video?
To loop your HTML5 video, you need to add the ‘loop’ attribute to the ‘video’ tag. Like the ‘autoplay’ attribute, the ‘loop’ attribute doesn’t need a value. Here’s an example:<video src="myvideo.mp4" loop></video>
This will make the video start over again, every time it is finished.
How can I control the volume of my HTML5 video?
The volume of an HTML5 video can be controlled using JavaScript. Here’s an example:var vid = document.getElementById("myVideo");
vid.volume = 0.2;
This will set the volume of the video to 20%. The value can be any number between 0.0 and 1.0.
How can I add subtitles to my HTML5 video?
Subtitles can be added to HTML5 videos using the ‘track’ element. The ‘src’ attribute specifies the source file for the subtitles, the ‘kind’ attribute specifies the kind of text track, and the ‘srclang’ attribute specifies the language of the text track. Here’s an example:<video src="myvideo.mp4" controls>
<track src="subtitles_en.vtt" kind="subtitles" srclang="en" label="English">
</video>
This will add English subtitles to the video.
How can I make my HTML5 video responsive?
To make your HTML5 video responsive, you can use CSS. Here’s an example:video {
max-width: 100%;
height: auto;
}
This will make the video scale up and down with the width of its container.
How can I add a poster image to my HTML5 video?
A poster image can be added to an HTML5 video using the ‘poster’ attribute in the ‘video’ tag. The ‘poster’ attribute specifies an image to be shown while the video is downloading, or until the user hits the play button. Here’s an example:<video src="myvideo.mp4" poster="poster.jpg"></video>
This will display the image ‘poster.jpg’ until the video starts playing.
How can I add multiple source files to my HTML5 video?
Multiple source files can be added to an HTML5 video using the ‘source’ element. The browser will use the first recognized format. Here’s an example:<video controls>
<source src="myvideo.mp4" type="video/mp4">
<source src="myvideo.ogg" type="video/ogg">
</video>
This will provide two different formats of the video, and the browser will choose the one it supports.
How can I add a download link to my HTML5 video?
A download link can be added to an HTML5 video using the ‘a’ element. Here’s an example:<a href="myvideo.mp4" download>Download Video</a>
This will create a link that, when clicked, will download the video.
How can I add controls to my HTML5 video?
Controls can be added to an HTML5 video using the ‘controls’ attribute in the ‘video’ tag. Here’s an example:<video src="myvideo.mp4" controls></video>
This will display the default video controls, which include play, pause, and volume controls.
How can I play and pause my HTML5 video using JavaScript?
An HTML5 video can be played and paused using JavaScript. Here’s an example:var vid = document.getElementById("myVideo");
function playVid() {
vid.play();
}
function pauseVid() {
vid.pause();
}
These functions will play and pause the video when called.
Malcolm Sheridan is a Microsoft awarded MVP in ASP.NET, ASPInsider, Telerik Insider and a regular presenter at conferences and user groups throughout Australia and New Zealand. Follow him on twitter @malcolmsheridan.
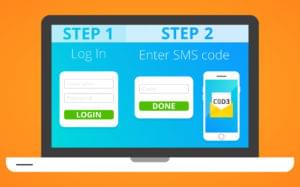
Published in
·APIs·Authentication·CMS & Frameworks·Frameworks·Laravel·Libraries·Patterns & Practices·PHP·Security·Web Services·February 28, 2017
Published in
·Design·HTML & CSS·Mobile·Mobile UX·Mobile Web Development·Responsive Web Design·July 2, 2014