Android apps have a wide variety of features, functionalities, and purposes. These various Android apps require similarly varied support from Android system APIs. Many apps require storing data in short-term memory that lasts only as long as the app is running. But, some apps need to store data or user preferences persistently, so that user data and user preferences are maintained indefinitely. In this article, we are going to demonstrate how we can store data in files, and also how can we store user preferences using Android APIs. We’ll create two small apps: one to store an read a file from user input, and one to store user preferences.
Creating the UI of the File Store App
Lets start by creating a simple app that shows an text editor and allows the user can input any text to be saved as a file.The saved text will be saved within the text editor next time the application is started by reading from the storage file. First, we’ll create the UI for the app. Within the UI we are going to create a text editor and a save button on screen. The layout for this UI is as follows:<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Enter the text"
/>
<EditText
android:id="@+id/textbox"
android:singleLine="false"
android:gravity="top"
android:lines="10"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<Button android:id="@+id/save"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Save the notes"
android:onClick="saveClicked"/>
</LinearLayout>
Above, we create a text view using the tag <TextView>, which just displays the text as “Enter The Text”.
Next, we create an editor to take in the text from the user. We create this using the <EditText> tag, and because we want the text to be multi-line, we also set the properties android:singleLine=“false” and android:lines=“10” on the EditText. Then, we create a button using the <Button> tag, which will be used to save the modified text to a file.
Once the layout is created, we’ll use the function onCreate to set this layout of the content view as follows:
public class FileDemo extends Activity {
/** Called when the activity is first created. */
private final static String STORETEXT="storetext.txt";
private EditText txtEditor;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
txtEditor=(EditText)findViewById(R.id.textbox);
}
}
Now, if we run the app, the UI will look as follows:
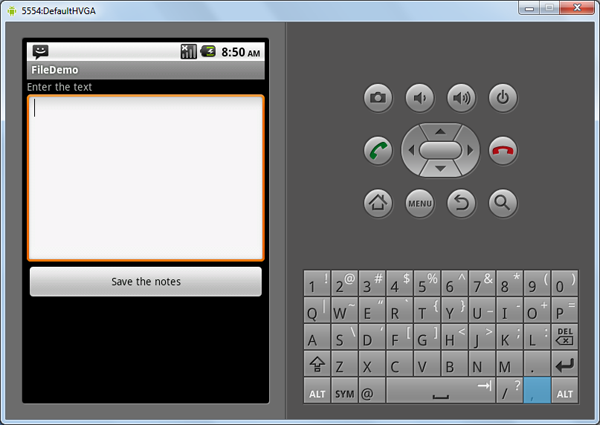
Saving the File
Once the UI is done, we need to add functionality to save the text contents to a file upon pressing the “Save” button. To do this, the code is as follows:public void saveClicked(View v) {
try {
OutputStreamWriter out=
new OutputStreamWriter(openFileOutput(STORETEXT, 0));
out.write(txtEditor.getText().toString());
out.close();
Toast
.makeText(this, "The contents are saved in the file.", Toast.LENGTH_LONG)
.show();
}
catch (Throwable t) {
Toast
.makeText(this, "Exception: "+t.toString(), Toast.LENGTH_LONG)
.show();
}
}
Once “Save” is clicked, the function saveClicked is called. In the function saveClicked, we open a file using the Android API openFileOutput by passing it the name of the file to open, which we defined in our class as
private final static String STORETEXT="storetext.txt";
Then, we create an object of OutputStreamWriter using the output of openFileOutput.
Finally, we get the text of the text editor and pass it to the write method of our OutputStreamWriter to write it to the file. Once the text is written to the file, we show a Toast to indicate that the text is saved. Below, the Toast reads “The contents are saved in the file.”
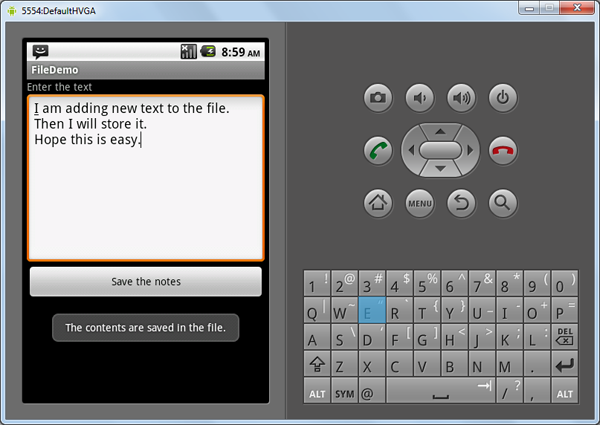
Reading the Text File Upon App Load
Now, let’s write a function that reads this newly-created file and populates the text editor with the contents of the file. We will call this function in the onCreate function so that as soon as the app launches, the editor is filled with the information from the file. The function is as follows:public void readFileInEditor()
{
try {
InputStream in = openFileInput(STORETEXT);
if (in != null) {
InputStreamReader tmp=new InputStreamReader(in);
BufferedReader reader=new BufferedReader(tmp);
String str;
StringBuilder buf=new StringBuilder();
while ((str = reader.readLine()) != null) {
buf.append(str+"n");
}
in.close();
txtEditor.setText(buf.toString());
}
}
catch (java.io.FileNotFoundException e) {
// that's OK, we probably haven't created it yet
}
catch (Throwable t) {
Toast
.makeText(this, "Exception: "+t.toString(), Toast.LENGTH_LONG)
.show();
}
}
In this function, we first open the file with the API openFileInput, and then we create an InputStream from it. From that, we create an InputStreamReader and a BufferedReader. Using the BufferedReader, we read line after line the text of the storage file, and we store the text in the buffer. Once the whole file is read, we send the text into the editor. Now, if the application is run, the application will load the the editor filed with the contents of the file, as shown below.
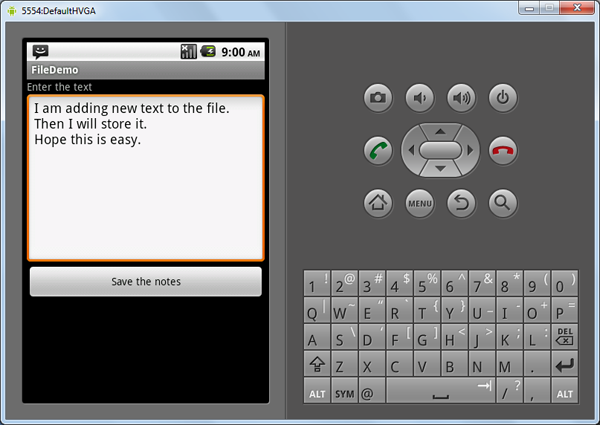
Shared Preferences Introduction
Shared preferences allow you to store key => value pairs of different settings that you want within your Android app, even if the app session is closed. You can store a number of native preference types, such as Boolean, int, strings, etc. Below, we are going to create a small app in which the user can store the preference of whether he wants the activities background color as blue or red.Creating a UI for storing Preferences
First, we must create the UI of the app. The layout for the app will be as follows:<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/myScreen"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Please select the preference of background color"
/>
<RadioGroup
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/prefgroup"
>
<RadioButton android:id="@+id/blue"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Blue" />
<RadioButton android:id="@+id/red"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Red" />
</RadioGroup>
<Button android:id="@+id/savepreferences"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Save Preferences"
android:onClick="savepreferencesClicked"
/>
</LinearLayout>
Above, we create a linear layout and then add a TextView to display the message that asks the user to select his background color preference. Then, we create a radio button group with two radio button to give the two color options to the user. Lastly, we create a button that will save the user’s color preference upon being pressed..
If we run the app now, the UI will look like this:
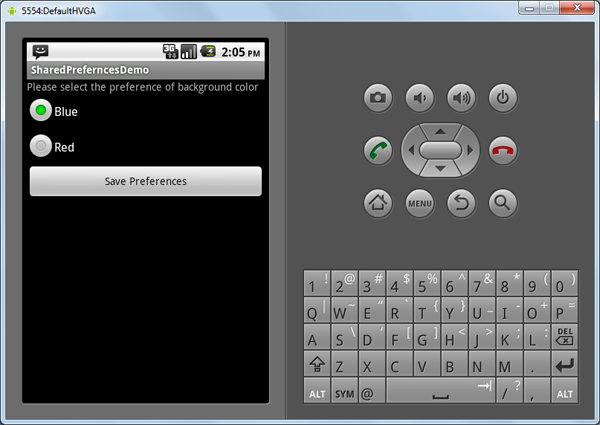
Saving the Preferences
Now, lets see how we can save the preference that the user has selected. The code to save the preference is as follows in the function saveBgColorPreference.public void saveBgColorPreference()
{
RadioGroup g = (RadioGroup) findViewById(R.id.prefgroup);
int selected = g.getCheckedRadioButtonId();
RadioButton b = (RadioButton) findViewById(selected);
String selectedValue = (String) b.getText();
SharedPreferences myPrefs = this.getSharedPreferences("myPrefs", MODE_WORLD_READABLE);
SharedPreferences.Editor prefsEditor = myPrefs.edit();
prefsEditor.putString("bgcolor", selectedValue);
prefsEditor.commit();
}
In this function, we get the RadioGroup, and we find the ID of the radio button that was selected. Then, we get the text of the selected radio button to check which color the user has selected. Then, we get the SharedPreferences object using the function getSharedPreferences. Because we want to edit the shared preferences, we have to get the SharedPreferences.Editor and then call the function putString on it to write the string and then call commit to finish the writing transaction. This will store the color value within the stored preferences.
Using the Saved Preferences to Change Properties of the App
Now that we’ve written code to store the user’s color preference, we will write a function setColorOnPreference to actually change the background color according to the preference. The function is as follows:public void setColorOnPreference()
{
mScreen = (LinearLayout) findViewById(R.id.myScreen);
SharedPreferences myPrefs2 = this.getSharedPreferences("myPrefs", MODE_WORLD_READABLE);
String prefName = myPrefs2.getString("bgcolor", "Blue");
if(prefName.equals("Blue"))
mScreen.setBackgroundColor(0xff0000ff);
else
mScreen.setBackgroundColor(0xffff0000);
}
In this function we get the linear layout using its ID. Then, we get the shared preferences object using the function getSharedPreferences. Then, using the function getString, we get the background color preference.
If the background color is blue, we set the background to blue. Otherwise, we set it to red.
Now, upon pressing the button, we will call the function savepreferencesClicked, which is as follows:
public void savepreferencesClicked(View v) {
saveBgColorPreference();
setColorOnPreference();
}
In order to start the app with the desired background color (of the users choice), we will call setColorOnPreference in onCreate as follows:
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
setColorOnPreference();
}
Now, if we run the app it will look as follows:
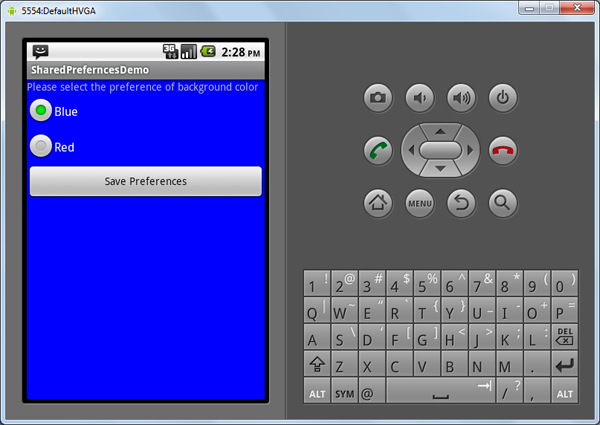
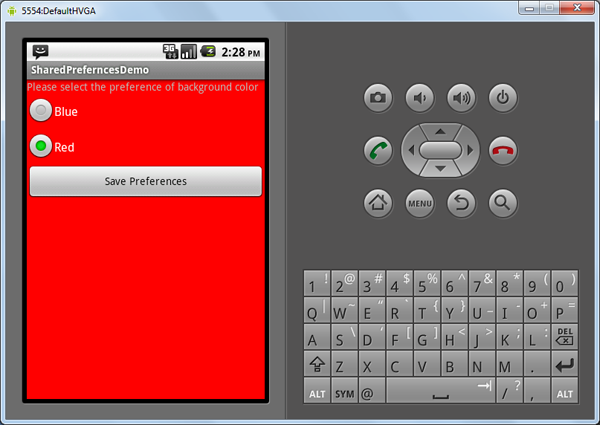
Conclusion
Android APIs provide good support for developers who want to share persistent data between two different sessions of an Android application. Using files and preferences APIs, we can store user data and preferences to make the application more personalized and practical for the end users. The flexibility with which the APIs are designed has made their use very simple, and apps can be developed very quickly using these powerful APIs. So, happy Android app development!Frequently Asked Questions (FAQs) about Storing User Data Using Simple Text Files and APIs in Android
What are the benefits of using simple text files for data storage in Android?
Simple text files are a straightforward and efficient way to store data in Android. They are easy to create, read, and write, making them ideal for storing small amounts of data. They don’t require complex queries or database management, which can simplify your code and reduce the potential for errors. Additionally, text files can be easily shared between different applications, making them a versatile choice for data storage.
How secure is data stored in simple text files in Android?
While simple text files are easy to use, they are not the most secure method for storing sensitive data. Anyone with access to the file system can read or modify these files. Therefore, it’s recommended to use them for non-sensitive data or to encrypt the data before storing it in a text file.
How can I use APIs for data storage in Android?
APIs, or Application Programming Interfaces, provide a set of rules and protocols for how software components should interact. In the context of data storage, APIs can be used to interact with databases, cloud storage services, or other data storage systems. For example, you might use an API to send a request to a database to store or retrieve data.
What are shared preferences in Android?
Shared preferences are a way to store private primitive data in key-value pairs in Android. They are ideal for storing small amounts of data like user settings or application state. The data stored in shared preferences persists across user sessions, even if your application is killed and restarted.
How can I secure data stored in shared preferences?
While shared preferences are private to your application, they are not encrypted and can be accessed if the device is rooted. To secure data in shared preferences, you can use Android’s built-in encryption APIs or third-party libraries to encrypt the data before storing it.
What is the difference between internal and external storage in Android?
Internal storage is private to your application and is not accessible by the user or other applications. External storage, on the other hand, is accessible by the user and other applications. While external storage provides more space, it’s less secure and the data can be lost if the user uninstalls your application or removes the external storage medium.
How can I store data in the cloud in Android?
Android provides several APIs and services for storing data in the cloud. These include Firebase, Google Drive API, and Google Cloud Storage. These services provide secure, scalable, and reliable storage for your application data.
How can I handle large amounts of data in Android?
For large amounts of data, it’s recommended to use a database. Android provides several options for this, including SQLite, Room, and Firebase Realtime Database. These databases provide powerful querying capabilities and can handle large amounts of data efficiently.
How can I share data between different Android applications?
Android provides several methods for sharing data between different applications. These include using intents, shared preferences, content providers, and file providers. The method you choose depends on the type of data you want to share and the level of security you need.
How can I backup and restore data in Android?
Android provides several APIs and services for backing up and restoring data. These include the Backup API, Google Drive API, and Firebase. These services can automatically backup your application data and restore it when the user reinstalls your application or switches to a new device.
Abbas is a software engineer by profession and a passionate coder who lives every moment to the fullest. He loves open source projects and WordPress. When not chilling around with friends he's occupied with one of the following open source projects he's built: Choomantar, The Browser Counter WordPress plugin, and Google Buzz From Admin.