jQuery Check if Window is in iFrame
Simple JavaScript code snippet to check whether or not a window is in an iFrame. Very useful for some functions that may make use of the URL in the address bar of the page.
Simple as this.
var isInIframe = (window.location != window.parent.location) ? true : false;
Also See:
Frequently Asked Questions (FAQs) about jQuery and iFrames
How can I use jQuery to check if a webpage is loaded inside an iFrame?
To check if a webpage is loaded inside an iFrame using jQuery, you can use the window.self
and window.top
properties. If a webpage is loaded inside an iFrame, window.self
will not be equal to window.top
. Here’s a simple code snippet to illustrate this:if (window.self !== window.top) {
// The page is in an iFrame
} else {
// The page is not in an iFrame
}
What is the difference between window.self
and window.top
in JavaScript?
In JavaScript, window.self
refers to the current window, while window.top
refers to the topmost window in the window hierarchy. If a webpage is loaded directly in the browser, window.self
and window.top
will be the same. However, if the webpage is loaded inside an iFrame, window.self
will refer to the iFrame window, and window.top
will refer to the main browser window.
Can I use jQuery to manipulate content inside an iFrame?
Yes, you can use jQuery to manipulate content inside an iFrame. However, due to the same-origin policy, you can only do this if the iFrame and the parent document are from the same domain. Here’s an example of how you can change the HTML of a body tag inside an iFrame:$("#myIframe").contents().find("body").html("Hello, World!");
How can I detect an iFrame using pure JavaScript, without jQuery?
You can detect an iFrame using pure JavaScript by comparing window.self
and window.top
, similar to the jQuery method. Here’s how you can do it:if (window.self !== window.top) {
// The page is in an iFrame
} else {
// The page is not in an iFrame
}
What is the same-origin policy, and how does it affect iFrames?
The same-origin policy is a security feature implemented in web browsers to prevent scripts from different domains from interacting with each other. This policy affects iFrames because it prevents scripts in the parent document from accessing or manipulating the content inside the iFrame if they are not from the same domain.
Can I bypass the same-origin policy to access an iFrame from a different domain?
Bypassing the same-origin policy is generally not recommended due to security reasons. However, if you have control over both the parent document and the iFrame content, you can use the document.domain
property or the postMessage
method to communicate between the two.
How can I use the postMessage
method to communicate between an iFrame and the parent document?
The postMessage
method allows you to send data between windows, even if they are not from the same domain. Here’s an example of how you can use it:// In the parent document
window.addEventListener("message", function(event) {
console.log("Received message:", event.data);
}, false);
// In the iFrame
window.parent.postMessage("Hello, parent!", "*");
Can I use jQuery to detect if a webpage is loaded inside an iFrame in all browsers?
Yes, the method of comparing window.self
and window.top
works in all modern browsers, including Chrome, Firefox, Safari, and Edge.
Can I load a webpage inside an iFrame using jQuery?
Yes, you can load a webpage inside an iFrame using the attr
method in jQuery to set the src
attribute of the iFrame. Here’s an example:$("#myIframe").attr("src", "https://www.example.com");
Can I use jQuery to resize an iFrame based on its content?
Yes, you can use jQuery to resize an iFrame based on its content. However, due to the same-origin policy, you can only do this if the iFrame and the parent document are from the same domain. Here’s an example:$("#myIframe").height($("#myIframe").contents().find("body").height());
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
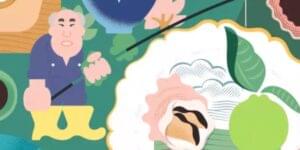
Published in
·Animation·Canvas & SVG·Design·Design & UX·HTML & CSS·Illustration·UI Design·UX·Web·June 9, 2022