Use jQuery to check your browser version in just a few lines of code you can optimise code for different browsers such as Firefox, IE, Safari, Chrome and more. The jQuery functions jQuery.Browser() and jQuery.Browser.Version() do all the hard work for us! Here is how you do it. //Example: output all version information about current browser
jQuery.each(jQuery.browser, function(i, val) {
$("
" + i + " : " + val + "")
.appendTo( document.body );
});
Example outputs:
Internet Explorer: 6.0, 7.0, 8.0
Mozilla/Firefox/Flock/Camino: 1.7.12, 1.8.1.3, 1.9
Opera: 10.06, 11.01
Safari/Webkit: 312.8, 418.9
//Example: Alerts “Do stuff for firefox 3” only for firefox 3 browsers.
var ua = $.browser;
if ( ua.mozilla && ua.version.slice(0,3) == "1.9" ) {
alert( "Do stuff for firefox 3" );
}
//Example: Set a CSS property to specific browser.
if ( $.browser.msie ) {
$("#div ul li").css( "display","inline" );
} else {
$("#div ul li").css( "display","inline-table" );
}
Frequently Asked Questions (FAQs) about jQuery and Browser Version Detection
How can I use jQuery to detect the browser version? jQuery provides a property called $.browser which can be used to detect the browser version. However, it’s important to note that this property was removed in jQuery 1.9 and is available only through the jQuery Migrate plugin. Here’s a simple example of how you can use it:if ($.browser.msie && $.browser.version < 9) { // actions to take for IE less than 9}This code checks if the browser is Internet Explorer and if its version is less than 9. Why is browser detection generally not recommended? Browser detection is generally not recommended because it can lead to brittle code. Browsers are constantly being updated and new ones are being released, so maintaining a list of all possible browser and version combinations can be challenging. Instead, feature detection is usually a better approach. This involves testing whether a specific feature is supported by the browser, rather than checking the browser itself. How can I perform feature detection using jQuery? jQuery provides a utility function $.support for feature detection. This function tests the current browser against various HTML and CSS features to see if they’re supported. Here’s an example:if ($.support.ajax) { // actions to take if AJAX is supported}This code checks if AJAX is supported by the browser. What is the jQuery Migrate plugin and why would I need it? The jQuery Migrate plugin is a tool that helps you to update older jQuery code to work with newer versions of jQuery. It restores deprecated features and behaviors so that older code will still run properly. If you’re using the $.browser property to detect the browser version, you’ll need the jQuery Migrate plugin because this property was removed in jQuery 1.9. How can I check what version of jQuery is loaded? You can check the loaded version of jQuery by using the $.fn.jquery property. This property returns a string containing the version number. Here’s how you can use it:console.log($.fn.jquery);This code will print the jQuery version number to the console. How can I load a specific version of jQuery? You can load a specific version of jQuery by including the appropriate script tag in your HTML. The script tag should point to the URL of the jQuery version you want to use. Here’s an example:<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>This code will load jQuery version 3.5.1. Can I use multiple versions of jQuery on the same page? Yes, you can use multiple versions of jQuery on the same page by using the $.noConflict method. This method releases the control of the `= variable, allowing you to use it with another library or another version of jQuery. Here’s an example:var jq1 = $.noConflict(true);In this code, jq1 is a new alias for the first loaded jQuery, and the `= variable is free to be used with another version. How can I use jQuery to detect the browser type? You can use the $.browser property to detect the browser type. This property contains flags for the browser, and it is available through the jQuery Migrate plugin. Here’s an example:if ($.browser.chrome) { // actions to take if the browser is Chrome}This code checks if the browser is Chrome. How can I use jQuery to detect mobile browsers? You can use the navigator.userAgent property in combination with a regular expression to detect mobile browsers. Here’s an example:if (/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)) { // actions to take if the browser is a mobile browser}This code checks if the browser is a mobile browser. How can I use jQuery to detect if JavaScript is enabled? You can use the <noscript> HTML tag to provide an alternative content for users who have JavaScript disabled. However, if you want to perform a check using jQuery, you can add a class to the <body> tag using JavaScript, and then check for this class using jQuery. Here’s an example:<body class="no-js"><script>document.body.className = '';</script>if ($('body').hasClass('no-js')) { // actions to take if JavaScript is disabled}This code checks if JavaScript is enabled. If it is, the no-js class will be removed by the script, and the jQuery check will return false. If JavaScript is disabled, the no-js class will remain, and the jQuery check will return true.
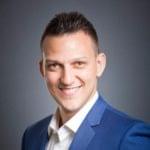
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
jQuery
Share this article