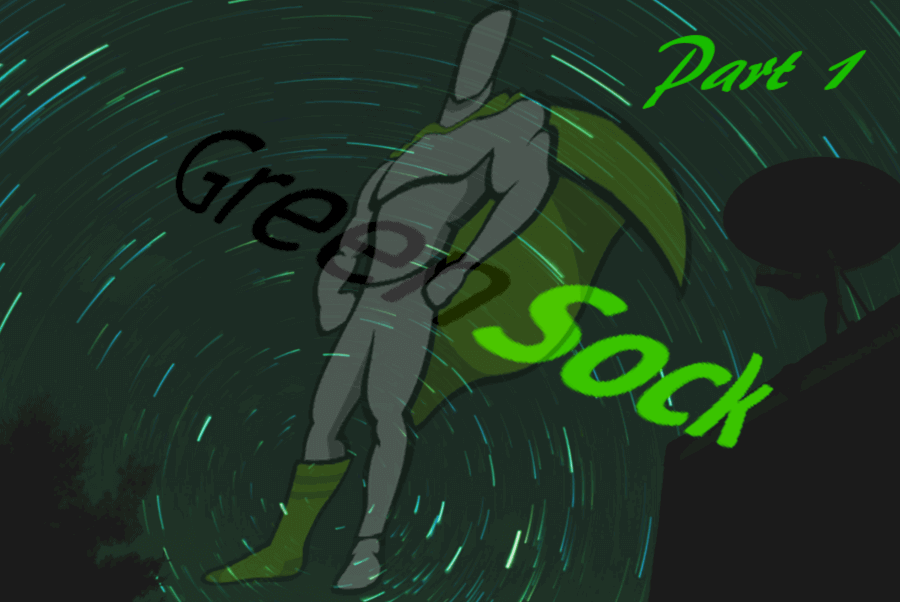
My aim in this article is to give you a thorough introduction to GreenSock, also known as GSAP (GreenSock Animation Platform), a super performant, professional-grade HTML5 animation engine for the modern web.
This is the fourth article in the series Beyond CSS: Dynamic DOM Animation Libraries.
Here’s what I covered in the past issues:
- Animating the DOM with Anime.js touches on how to make the best use of animation on the web and when you could consider using a JavaScript animation library instead of CSS-only animation. It then focuses on Anime.js, a free and lightweight JavaScript animation library
- Fun Animation Effects with KUTE.js introduces you to KUTE.js, a free and feature-rich JavaScript animation library
- Make Your Website Interactive and Fun with Velocity.js (No jQuery) shows you how to use Velocity.js, an open source, robust free animation library, to create performant web animations.
GSAP has too many features to fit in one article. This is why I’ve opted for a multi-part article all dedicated to various areas of the GreenSock library.
In more detail:
- By the end of this first part, you’ll have learned about GreenSock’s capabilities and features, licensing model, core components, and basic syntax to tween and stagger DOM elements
- In part 2, I’ll introduce GreenSock’s native timeline functionality
- Finally, part 3 will focus on some powerful bonus plugins GreenSock makes available to accomplish complex animation tasks easily with a few lines of code.
If you’re already a GSAP Ninja, check out Animating Bootstrap Carousels with the GSAP Animation Library, where George Martsoukos illustrates an effective use of GreenSock for UI animation.
Without further ado, brace yourself, the journey is about to begin!
What is GreenSock and What Is It Good For?
GreenSock is the de facto industry-standard JavaScript animation platform available today.
It’s a mature JavaScript library that has its roots in Flash-based animation. This means the guys behind GreenSock know web animation inside-out, the library has been around for a long time and is not going anywhere any time soon.
GSAP includes a comprehensive suite of tools, utilities, and plugins you can leverage to handle any web animation challenge you happen to face, from animating SVGs consistently across multiple browsers to coding complex animation sequences, dragging elements across the screen, splitting and scrambling text, and so much more.
To mention just three things I particularly love about GreenSock:
- Although the library is mega rich in terms of features, the learning curve is relatively shallow because it uses an intuitive and consistent syntax across all its various implementations and plugins. In addition, it offers awesome documentation, tutorials, and support via the GSAP Forums. Also, the library itself is continually updated and maintained. These are all crucial factors when building a project which relies on an external library for any of its key features and user experience
- It’s modular and light-weight, which means it won’t add bloat to your web projects
- Thanks to its powerful timeline functionality, GSAP affords precise control over the timings not only of single tweens, but also of multiple tweens as part of a whole animation flow.
Core GreenSock Tools
These are GreenSock’s core modules:
- TweenLite — the foundation of GSAP, a lightweight and fast HTML5 animation library
- TweenMax — TweenLite’s extension, which besides comprising TweenLite itself, includes:
- TimelineLite
- TimelineMax
- CSSPlugin
- AttrPlugin
- RoundPropsPlugin
- DirectionalRotationPlugin
- BezierPlugin
- EasePack
- TimelineLite — a lightweight timeline to take control of multiple tweens and/or other timelines
- TimelineMax — an enhanced version of TimelineLite, which offers additional, non essential capabilities like
repeat
,repeatDelay
,yoyo
, and more.
You can choose to include TweenLite in your project and then add other modules separately as you need them. Alternatively, you can choose to include TweenMax (the approach I’m going to take in this multi-part series), which packages all the core modules in one optimized file.
It’s also worth noting that GSAP offers some paid extras like the DrawSVGPlugin to create animated line drawing effects with SVGs, the powerful MorphSVGPlugin to morph one SVG shape into another (even without requiring that the two shapes have the same number of points), and others. Although you need to be a paying member of the Club GreenSock to use these plugins, GSAP makes available to you for free a special CodePen-based version so that you can try them out. This is a cool offer I’m going to take full advantage of later on in part 3 (and you with me).
License
GSAP hasn’t adopted a free open-source license like MIT largely for reasons that concern keeping the product’s quality high and its maintenance financially sustainable over the long term.
GreenSock makes available two licenses:
- Standard License — use of the library is totally free in digital products that are free to use (even if developers get paid to build them)
- Business Green — this license includes three tiers with the middle tier, Shockingly Green, being the most popular. As a Shockingly Green member you’ll gain access to all the bonus plugins and extras, but without the commercial license.
Despite the non-adherence to MIT and similar free use licenses, by letting you peek into its raw code both on the project’s GitHub repo and in your downloads, GreenSock encourages you to learn from its developers’ code and their mastery of JavaScript animation, which is one of the best features of the open source philosophy.
Tweening with GreenSock
A simple animation consists of some kind of change that takes place over time from point A to point B. Animators have a special name for the states in between A and B, i.e., tween.
TweenLite and TweenMax are the two powerful tweening tools GreenSock puts at your disposal. As I said above, I’m going to focus on TweenMax here, but remember that the basic syntax is the same in both libraries.
To load GSAP in your project, add this <script>
tag before the closing </body>
tag of your HTML document:
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/1.19.1/TweenMax.min.js"></script>
You can find the latest version available on the CDN here: cdnjs.com.
If you use npm
, type this in your terminal:
npm install gsap
On CodePen, you’ll find the link to GSAP TweenMax in the Quick add box inside the JavaScript Settings:
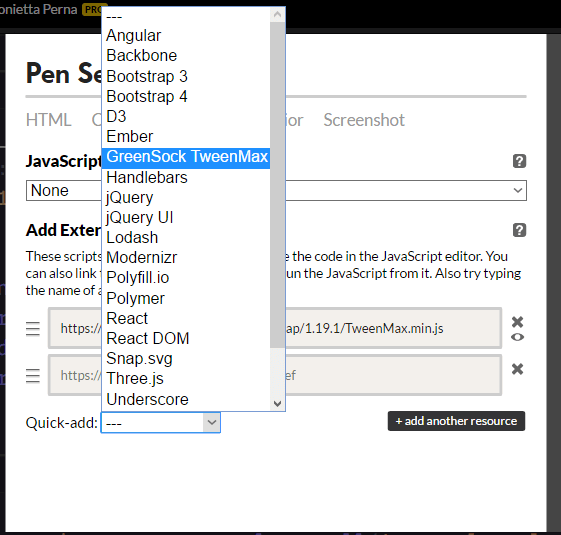
A Basic Tween with GreenSock: the Syntax
A simple tween with GSAP looks like this:
TweenMax.to('.my-element', 1, {opacity: 0});
The snippet above animates a DOM element with the class of my-element
from the current default opacity value to the opacity value of 0 over the course of 1 second. In other words, at the end of a 1-second long tween, the element will no longer be visible.
Let’s go into a bit more detail.
TweenMax.to()
tells the browser you’re using the to()
method of the TweenMax library (the syntax for TweenLite is the same, only replace TweenMax with TweenLite). This method needs a few comma-separated arguments:
- The element you want to animate (‘.my-element’)
- The duration of the tween (in this case is 1 second)
- The vars object {}, that is, the properties you want to tween (in this case the
opacity
property). This last part goes into curly braces and takes the form of key/value pairs. The value expresses the final state of the tween. There are also other properties you can use inside thevars object {}
like callback functions, delays, etc.
As its name suggests, the to()
method animates tweenable properties from their default values to what you want those values to be at the end of the animation.
GSAP offers tons of methods, but the core ones besides to()
are:
from()
— lets you decide the starting values in your tween. Therefore the element will animate starting from the values you specify insidefrom()
towards its default values, which are usually those you set in your CSS rules. The syntax looks like this:
TweenMax.from('.my-element', 1, {opacity: 0});
fromTo()
— with this method, you specify the values for both the starting point and end point in your tween, which affords closer precision. The syntax is similar to the previous methods, but it includes the vars object{} both for the from (opacity: 1
) and to parts (opacity: 0
):
TweenMax.fromTo('.my-element', 1, { opacity: 1 // from state }, { opacity: 0 // to end state });
Here are all three methods in action as they are applied on the rotation and translation properties:
See the Pen Basic GreenSock Tweens by SitePoint (@SitePoint) on CodePen.
GSAP breaks away from the CSS syntax by using rotation
instead of rotate
.
Also, notice that to translate an element along the X or Y axis, GreenSock doesn’t use transform: translateX()
or transform: translateY()
. Rather, it uses x
(as in the live demo), y
, xPercent
, and yPercent
.
While the x
and y
properties are mostly pixel-based, xPercent
and yPercent
use percentage values to translate elements along the X and Y axis respectively (CSSPlugin docs).
Finally, keep in mind that to translate the element on the X or Y coordinates, you need to set the element’s CSS position
property either to relative
or absolute
.
Which CSS Properties Does GSAP Animate?
With GreenSock all CSS animatable properties are at your fingertips. You can animate CSS transforms, as discussed above, but also other complex properties such as box-shadow: 1px 1px 1px rgba(0,0,0,0.5)
and border: 1px solid red
. Just remember to use camelCase wherever the standard CSS property name uses a dash (-), e.g., backgroundColor
instead of background-color
.
Using GreenSock’s set()
Method
If you need to set some properties with specific values before animating them, you can do so either in your CSS or using GreenSock’s set()
method. This last option offers the advantage of keeping all your animation-related code in one place, i.e., the JavaScript document.
For instance, the first example in the live demo above starts with the image set in a slightly slanted position. This initial choice is not specified in the CSS document but in the JavaScript document using GSAP’s set()
method, like this:
TweenMax.set(toElement, {rotation: -45});
The syntax is the same as a regular tween, but without the duration parameter. This ensures that the change takes place immediately.
Creating Sequences of Tweens with GreenSock
You can create sequences of tweens with GreenSock and coordinate their interaction by adjusting each tween’s duration
and delay
properties.
For instance, in the code sample below, clicking the Notify Me button triggers the following animation sequence:
- An input box appears
- As the input box gets longer, the Notify Me button disappears
- Finally, a smaller Send button pops into view inside the input box.
Here’s the GSAP code for the sequence (the code goes inside a click handler for the button):
// input box appears and gets bigger
TweenMax.to(emailInput, 0.5, {
autoAlpha: 1,
scale: 1
});
// the button being clicked disappears
TweenMax.to(this, 1, {
autoAlpha: 0
});
// the send button appears and gets bigger
TweenMax.to(sendButton, 0.5, {
autoAlpha: 1,
scale: 1,
delay: 0.5,
ease: Back.easeOut.config(3)
});
In the snippet you can see two new properties — autoAlpha
and ease
.
You can use autoAlpha
instead of opacity
when you need to make sure the element not only disappears from view, but users can’t interact with it. In fact, autoAlpha
adds visibility: hidden
to opacity: 0
.
The ease
property lets you choose easings for your animations, which control the rate of change throughout the duration of a tween. This is a crucial ingredient for natural, realistic and smooth animations, and GreenSock offers the Ease Visualizer to give you huge control on the kind of ease that best fits your animation.
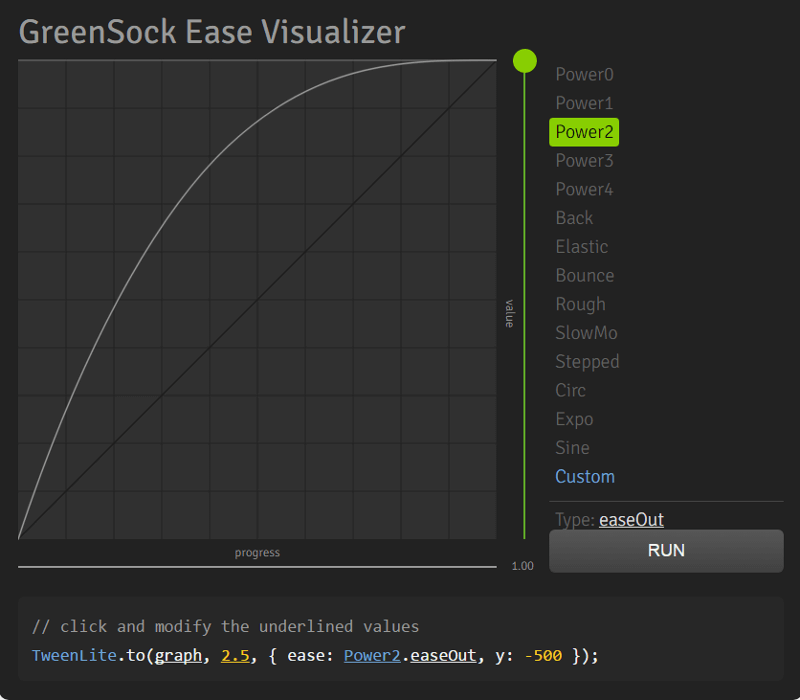
After a few tries, I chose the Back.easeOut
ease for this particular tween, but you can have a play and come up with something different.
Here’s the full demo on CodePen:
See the Pen Sequences of Tweening with GreenSock by SitePoint (@SitePoint) on CodePen.
Staggering Animations with GreenSock
The staggering effect consists in applying the same animation to a group of elements. However, the animation affects each element in succession, that is, one element at a time rather than all elements at once.
GSAP TweenMax offers the staggerTo()
, staggerFrom()
, and staggerFromTo()
methods, which work similarly to the methods discussed above. Only the staggering effect is different, and it requires an extra argument just after the vars object {} to indicate the amount of time you want to elapse between each stagger.
Here’s an example:
TweenMax.staggerFrom(alice, 0.5 {
rotation: 0,
delay: 1.5,
ease: Power4.easeIn
}, 0.5);
In the snippet above, each instance of Alice will rotate from 0 degrees to the values I have set in my CSS with half a second between each rotation:
And here’s the demo using the full code:
See the Pen Simple GSAP stagger method by SitePoint (@SitePoint) on CodePen.
Taking Control of Your GSAP Tweens
GreenSock has a few methods up its sleeve to let you take full control of your tweens.
The ones I’m going to consider here are:
play()
— plays the animation from wherever the playhead is or from a specific time:
// plays from the playhead's current location myAnimation.play(); // starts playing from 2 seconds into myAnimation // (all events and callbacks before then don't get fired) myAnimation.play(2); // starts playing from 2 seconds into myAnimation but // doesn't suppress events or callbacks in the initial // part of myAnimation (earlier than 2 seconds) myAnimation.play(2, false);
pause()
— pauses your tween, either completely or starting from a specific point:
// pauses myAnimation in all its entirety myAnimation.pause(); // jumps 2 seconds into myAnimation and then pauses myAnimation.pause(2); // jumps 2 seconds into myAnimation then pauses but // doesn't suppress events/callbacks during the // initial phase of the tween (earlier than 2 seconds) myAnimation.pause(2, false);
reverse()
— moves your tween backwards to the initial frame, but you can choose to set a specific time to start from before reversing
// moves backwards from wherever the playhead currently is myAnimation.reverse(); // moves backwards from exactly 2 seconds into myAnimation myAnimation.reverse(2); // moves backwards from exactly 2 seconds into myAnimation // but doesn't suppress events/callbacks before then myAnimation.reverse(2, false);
- restart() — restarts your tween and plays it from the beginning:
// restarts myAnimation, bypassing any predefined delay myAnimation.restart(); // restarts myAnimation, including any predefined delay, // and doesn't suppress events/callbacks during // the initial move back to time:0 myAnimation.restart(true, false);
- resume() — resumes playing, either forwards or backwards depending on the current direction of the animation. You can also set the animation to jump to a specific time before resuming it:
// resumes myAnimation from the playhead's current position myAnimation.resume(); // jumps to exactly 2 seconds into myAnimation // and then resumes playback: myAnimation.resume(2); // jumps to exactly 2 seconds into myAnimation // and resumes playback but doesn't suppress // events/callbacks during the initial move: myAnimation.resume(2, false);
See the Pen Controlling GSAP Tweens by SitePoint (@SitePoint) on CodePen.
It’s impossible to list all methods GSAP’s TweenLite and TweenMax make available to you. Therefore, I encourage you to check out the docs — they’re super friendly.
TweenMax yoyo, repeat, and repeatDelay
I’d like to close this first article dedicated to GreenSock with three useful features you can use in your TweenMax-powered animations:
repeat
— use this if you want your tween to play a set number of times or indefinitely. In this last case, setrepeat
to the value of -1:
// the tween repeats 3 times on top of the default // time (4 times overall): TweenMax.to(element, 1, { opacity: 0, repeat: 3 });
repeatDelay
— you can use this property to set a specific delay between each of your animation’s repetitions:
// the tween repeats 3 times on top of the default // time (4 times overall) with one a second delay // between each repetition: TweenMax.to(element, 1, { opacity: 0, repeat: 3, repeatDelay: 1 });
yoyo
— is very handy when you want your animation to go back and forth a set number of times, or indefinitely. Use it in conjunction withrepeat
to create movements that alternate between two states:
// the tween repeats indefinitely with one second's delay // between each repetition. The element alternates // between being fully opaque and fully transparent: TweenMax.to(element, 1, { opacity: 0, repeat: -1, repeatDelay: 1 });
See the Pen Yoyo, repeat, and repeatDelay in GSAP TweenMax by SitePoint (@SitePoint) on CodePen.
Conclusion
In this first part dedicated to the GreenSock library, I’ve discussed what GreenSock excels at, its capabilities, core components, and licensing model.
I’ve also introduced you to GSAP’s basic syntax for tweening DOM elements with TweenMax using a number of live demos.
However, although you can create some cool animations just sequencing GSAP tweens, there are a couple of shortcomings to this approach:
- If you change a tween’s
duration
ordelay
in a sequence of tweens, it’s likely most of the overall animation will be messed up and in need of further adjustments - A sequence of tweens is made up of independent tweens, therefore you can’t pause, resume, reverse, etc. the entire sequence as a whole.
To give you extra control, GSAP offers robust and flexible timeline functionality, which I discuss in the second instalment dedicated to this awesome library.
Have you used GreenSock in your web animation work? I’d love to see what you’ve created with it.
If you’re curious about JavaScript-based animation and performance, the JS with Performance: requestAnimationFrame screencast is available to all SitePoint Premium members.
Ready for more GreenSock power? Then, head over to Part 2 and learn all about using GSAP Timeline.
Frequently Asked Questions (FAQs) about Web Animation
What is GSAP and why is it important in web animation?
GSAP, or GreenSock Animation Platform, is a powerful JavaScript library used for creating high-performance animations that work in all major browsers. It’s important in web animation because it provides a flexible and efficient way to animate HTML elements, SVG, React, Vue, and other platforms. GSAP is known for its speed, reliability, and flexibility, making it a preferred choice for many web developers and designers.
How can I get started with GSAP?
To get started with GSAP, you first need to include the GSAP library in your project. You can do this by downloading the library from the official GSAP website or by using a CDN link. Once you’ve included the library, you can start creating animations by selecting elements and defining animation properties such as duration, delay, and easing.
What are the key components of GSAP?
GSAP consists of several key components including TweenLite, TweenMax, TimelineLite, and TimelineMax. TweenLite is the core engine for creating simple animations, while TweenMax extends TweenLite and provides additional features like repeat, yoyo, and more. TimelineLite and TimelineMax are used for sequencing animations.
How can I animate an element with GSAP?
To animate an element with GSAP, you first need to select the element using standard JavaScript or jQuery selectors. Then, you can use the GSAP methods like TweenLite.to or TweenMax.fromTo to animate the element. You can specify the duration of the animation, the properties to animate, and the easing function to use.
What is Tweening in GSAP?
Tweening in GSAP refers to the process of creating an animation by interpolating between two or more values over a certain period of time. GSAP provides several methods for tweening including TweenLite.to, TweenLite.from, and TweenMax.fromTo.
How can I control the timing of animations in GSAP?
GSAP provides a powerful sequencing tool called Timeline. With Timeline, you can control the timing of multiple animations, group animations together, and even nest timelines within other timelines. You can also control the playback of a timeline using methods like play, pause, reverse, and seek.
Can I use GSAP with React or Vue?
Yes, GSAP can be used with any JavaScript framework or library, including React and Vue. GSAP provides a plugin called ScrollTrigger that makes it easy to create scroll-driven animations in React and Vue.
How can I create complex animations with GSAP?
GSAP provides a feature called Stagger that allows you to create complex animations with ease. With Stagger, you can animate multiple elements with a delay between each animation, creating a ripple or domino effect.
Can I use CSS properties in GSAP animations?
Yes, GSAP allows you to animate almost any CSS property. You can animate properties like width, height, color, opacity, transform, and more. GSAP also provides a CSSPlugin that makes it easy to animate CSS properties.
How can I optimize my GSAP animations for better performance?
To optimize your GSAP animations, you should minimize the number of animated properties, use the will-change CSS property to hint browsers about upcoming animations, and use the GSAP performance monitor to identify and fix performance bottlenecks.
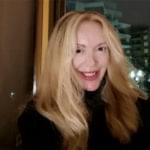
Maria Antonietta Perna is a teacher and technical writer. She enjoys tinkering with cool CSS standards and is curious about teaching approaches to front-end code. When not coding or writing for the web, she enjoys reading philosophy books, taking long walks, and appreciating good food.