Been looking at some code recently that provides a mechanism to localize a user interface for different human languages. The mechanism for doing so looks something like this (repeated many times over);
'Name',
'btn_ok' => 'OK',
);
?>
'Nombre',
'btn_ok' => 'AUTORIZACION',
);
The code in question isn’t an exception. I’ve seen many PHP applications handle localization in this way. In Internationalization and Localization with PHP the conclusion a “naive” reader might come to is this is the default best practice. Nothing wrong with the article but the necessity of simple example / short prose leaves other areas to the readers imagination.
The big problem with localization in this manner is that it’s happening at runtime. Every page request resulted in the localized version of the page being generated dynamically – see here for a rough idea of why that’s not so good.
Now it may be possible to cache the output HTML and store it as a static file but in a real example it’s likely we’ll have truly dynamic data mixed in there, such as something from a database, which makes caching tricky. Without caching the translation adds a significant baseline overhead to the generation of each page, which will increase the more complex the UI becomes.
Meanwhile there will be only a finite number of translations of the user interface, which will vary only when the UI itself changes (rarely – certainly not on a pre-request basis). So wouldn’t it be better to have multiple versions of the drawNameForm() function, one per language e.g.;
Harry Fuecks is the Engineering Project Lead at Tamedia and formerly the Head of Engineering at Squirro. He is a data-driven facilitator, leader, coach and specializes in line management, hiring software engineers, analytics, mobile, and marketing. Harry also enjoys writing and you can read his articles on SitePoint and Medium.
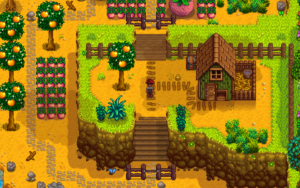
Published in
·Extensions·JavaScript·Laravel·Libraries·Miscellaneous·Performance·Performance & Scaling·PHP·Scaling·September 13, 2017