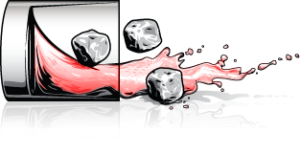
Padrino is an excellent website framework that makes it easy to implement complex web applications in a lightweight environment. Although it’s built on Sinatra, Padrino uses a Rails-like approach when generating code, establishing an organized file structure, and implementing database functionality.
This article briefly introduces the Padrino framework and how it can be used to create a simple admin interface within a Sinatra-like environment.
A Brief Introduction to Sinatra
In a nutshell, Sinatra is a DSL (Domain-Specific Language) that processes HTTP connections. Specifically, Sinatra compares incoming user requests with entries in a list. When the first match is found, Sinatra executes the associated code and sends the results back to the user.
For example, consider an HTTP GET
request arriving on this URL:
www.example.com/product/shoehorn/index.html
The DSL code within your Sinatra implementation may look like this:
get 'product/laces' do
haml :show_laces
end
get 'product/shoehorn' do
haml :shoehorn
end
put 'order/shoppingcart' do
haml :cart
end
In the above code, the words get
and put
may look like Ruby commands, but in reality, they’re part of a filter that looks for HTTP verbs like GET
, PUT
, POST
and DELETE
.
The second filter entry in the above DSL code matches the /product/shoehorn
URL, so anything between the do
and its matching end
will get executed, and the results will be sent back to the viewer’s browser. In this case, a reference is made to a file that contains Haml code, which Ruby will render into a web page.
Actual DSL structures are typically a lot more complex than the above example. For instance, before
and after
filters can be used to pre-process and post-process the results. Also, match filters can contain regular expressions as well a several other types of directives. Furthermore, the value returned to the viewer’s browser need not be HTML; in the case of a RESTful API implementation, it will consist of some JSON-encapsulated data, straight from the database, without being rendered into a view.
The bottom line with Sinatra is that the DSL implements an HTTP router. It is a very light web-processing engine that is very flexible. The downside, as you might imagine, is that the developer is forced to do everything. It can therefore be very difficult to build complex web applications. For example, it can cost days of development time to do something as simple as an administrative interface.
Padrino: Sinatra Extended
Padrino extends Sinatra by creating a simple file structure around it and providing generators to quickly get your code up and running.
For example, after installing Padrino (gem install padrino
), you can create a basic web application with the following command:
padrino g project my_website -d activerecord -b
This is similar to code generation in Rails, where the system generates a group of directories and files intended to get you up and running quickly. In this case, a root directory, my_website
, is created, and other files and subdirectories are stored within. Notice how the -d
switch brings in the ActiveRecord ORM, and the -b
switch instructs bundler to install all the dependencies.
The important subdirectories created within my_website are:
app
— where the configuration and controllers for your primary application are stored. (More on “primary” below)config
— where files pertaining to the all internal applications are storedpublic
— where images, style sheets and JavaScript files are stored
Note the use of the word “primary” above. One of the great things about Padrino is that it can package other applications into the main application. In this case, the main app
directory is used to keep track of the primary my_website
application, whereas information within the config
directory tracks mounted applications. (We’ll see how this all works when mounting the admin application below.)
At this point, we have a minimally functional web application, albeit without any type of scaffolding that you get with Rails. To make this application actually do something, we’ll implement a minimal Sinatra-like DSL. Just to get started, we’ll put something into the bottom of the ‘my_website/app/app.rb’ file as shown below.
get '/' do
'Hello World!'
end
Start the web server by executing the following command from within the my_website
directory:
padrino start
Then we navigate to https://localhost:3000 to see the results of our genius at work. Indeed, if all worked out well, we see the classic, “Hello World!” statement within the browser.
At this point, we could continue on our merry way, writing Sinatra code and tucking it away into the my_website/app/app.rb
file; however, we would be missing the point. The real beauty of Padrino lies in its ability to create applications and mount them into the framework.
The Admin Application
Let’s build a website that restricts access to a range of URL addresses. More specifically, we’ll allow all users to access the /
area, but restrict access to the /admin
area. In other words, everyone can access URLs starting with:
https://localhost:3000/
except for addresses starting with:
https://localhost:3000/admin
Luckily, the Padrino developers have already done most of the hard work. All we need to do is install the admin
application that they created.
Keep in mind that the admin application will need to reference a database in order to store usernames and passwords. As per the information we used when generating this Padrino application, the database will use the ActiveRecord ORM and create a default sqlite adapter. (Note: We have many choices on databases and adapters.)
Install the admin application with this commande, again executed from within the my_website
main directory:
padrino g admin
Note that immediately after executing this command, we now see new subdirectories:
db
— containing database migrations under the subdirectorymigrate
as well as some database seeding code,seeds.rb
.admin
— containing code for the installed admin application. Note that this subdirectory contains theapp.rb
file, defining the specifics of the protected and unprotected URL routes.models
— containing model information
Also, notice that the apps.rb
code within the config
directory has been changed to include a mount point for the new admin application. By default, the mount point was changed to /admin
. In other words, accesses to the location
https://localhost:3000/admin
will now be restricted to those who have admin privileges. Of course, you are free to change the mount point, if you wish. (Technically, this mount point puts the code within the /admin/app.rb
file in control of access rights within the https://localhost/admin
URL path.)
Additionally, you are provided a default configuration for the database migration, as appears in the db/migration/001_create_accounts.rb
file shown below. The database initially tracks, among other things, the user’s email address, password, and role. The email address and password are used for login information, and the role determines the set of privileges. Initially, the role will simply be “admin,” but you can create more roles with different access rights.
class CreateAccounts < ActiveRecord::Migration
def self.up
create_table :accounts do |t|
t.string :name
t.string :surname
t.string :email
t.string :crypted_password
t.string :role
t.timestamps
end
end
def self.down
drop_table :accounts
end
end
Run the database migration using the command:
padrino rake ar:migrate
Obviously, when you initially create the admin account, you’re going to have to specify the admin user’s email address and password. This process takes place when you seed your database, as shown below:
padrino rake seed
After executing the above command, you’ll be guided through the process of creating the admin account information, as guided by the information in the db/seeds.rb
file.
If everything works correctly, you should be able to go to the location:
https://localhost:3000/admin
where you’ll get redirected to the admin/sessions/new
page that provides a login form. When you login with the admin identity, you’ll get the default admin page as shown below. You can click on the accounts tab and get a list of the current account holders. You can even add more admins or change the profile of existing admins.
There is much you can do to customize your administrative interface. By implementing simple changes in the admin-related files, you can add different roles for different classes of users, and you can associate groups of these roles with different routes.
Summary
Padrino is used to implement complex code in a lightweight environment. You can begin with a basic Padrino installation, and then load applications in a modular fashion. This article uses this simple approach to create a basic Padrino application and demonstrate that its operation is very similar to Sinatra. It then installs a complete administrative interface with just a few lines of code.
Frequently Asked Questions about Simple Admin Padrino
What is Simple Admin Padrino and how does it work?
Simple Admin Padrino is a powerful tool that allows developers to create admin interfaces for their applications. It works by providing a simple and intuitive interface that can be easily customized to suit the needs of any application. This tool is built on the Padrino framework, which is a lightweight and flexible Ruby web framework. With Simple Admin Padrino, developers can manage their application’s data, monitor user activity, and perform other administrative tasks with ease.
How do I install Simple Admin Padrino?
Installing Simple Admin Padrino is a straightforward process. First, you need to add the gem ‘padrino-admin’ to your Gemfile. Then, run the command ‘bundle install’ to install the gem. After that, you can use the Padrino generator to create a new admin application. The command ‘padrino g admin’ will generate a new admin application with a default layout and set of views.
How can I customize the interface of Simple Admin Padrino?
Simple Admin Padrino provides a high level of customization. You can customize the interface by modifying the views and layouts. The views are written in ERB (Embedded Ruby), which is a templating system that embeds Ruby code in HTML. You can modify these views to change the appearance and functionality of the admin interface. The layouts define the overall structure of the interface, and you can modify them to change the layout of the admin interface.
How do I manage data with Simple Admin Padrino?
Simple Admin Padrino provides a powerful and intuitive interface for managing data. You can create, read, update, and delete data using the admin interface. The interface provides a form for creating new records, a table for viewing existing records, and forms for updating and deleting records. You can also search for records using the search bar.
How do I monitor user activity with Simple Admin Padrino?
Simple Admin Padrino provides a feature for monitoring user activity. You can view a log of user activity in the admin interface. This log includes information about the actions that users have performed, the time of these actions, and the users who performed them. This feature can be useful for tracking user behavior and identifying potential issues.
How does Simple Admin Padrino compare to other admin tools?
Simple Admin Padrino stands out for its simplicity and flexibility. Unlike some other admin tools, it does not impose a specific structure or workflow. Instead, it provides a flexible framework that you can customize to suit your needs. It also provides a simple and intuitive interface that makes it easy to manage your application’s data and monitor user activity.
What are the requirements for using Simple Admin Padrino?
To use Simple Admin Padrino, you need to have Ruby installed on your system. You also need to have the Padrino gem installed. If you are using Bundler, you can add the gem to your Gemfile and run ‘bundle install’. If you are not using Bundler, you can install the gem manually by running ‘gem install padrino’.
Can I use Simple Admin Padrino with other frameworks?
Simple Admin Padrino is built on the Padrino framework, and it is designed to work seamlessly with this framework. However, it is also possible to use Simple Admin Padrino with other Ruby frameworks. You may need to make some modifications to the code to ensure compatibility with other frameworks.
How do I troubleshoot issues with Simple Admin Padrino?
If you encounter issues with Simple Admin Padrino, you can start by checking the error messages. These messages can provide clues about the cause of the issue. You can also check the documentation for information about common issues and their solutions. If you are still unable to resolve the issue, you can seek help from the community. There are many forums and discussion groups where you can ask questions and get help from other developers.
How can I contribute to the development of Simple Admin Padrino?
If you are interested in contributing to the development of Simple Admin Padrino, you can start by checking the project’s GitHub page. There, you can find information about the project’s development process, including how to submit bug reports and feature requests. You can also contribute by submitting pull requests with improvements to the code.
Dan Schaefer is a web designer living in Ventura County, California. He’s been a part of several startups, doing everything from engineering to sales to marketing.