Private Composer Packages with Gemfury
Key Takeaways
- Gemfury is a Platform-as-a-Service (PaaS) solution for hosting private Composer packages, offering an alternative to self-hosted options like Toran Proxy or static repositories like Satis. It supports various languages, including PHP Composer packages, Ruby Gems, Node.js npm, Python PyPi, APT, Yum and Nu-Get.
- To use Gemfury, you need to create an account, create a package, and upload it to the platform. This can be done by using Git and letting Gemfury handle the rest, or by manually zipping up your package’s source code and uploading it via the Gemfury dashboard.
- You can add your private repository to your project’s composer.json file. This allows you to use any of the private Composer packages you create with Gemfury without needing to add separate repositories each time you want to use a package.
- Gemfury also provides a command-line tool for managing your packages. The tool allows you to list your packages, see the versions of a specific package, and more. It can be installed with the command ‘sudo gem install gemfury’.
Hopefully you’re familiar with Composer, the latest – and probably greatest – PHP package manager. If not, check out Alexander’s introduction, and I’ve no doubt you’ll be sold on it immediately. You’ll need a working knowledge of it to get the most out of this article.
Composer works effectively and seamlessly in conjunction with Packagist, a comprehensive repository of public packages.
However, sooner or later the time will come when you’ve written your own package which, for whatever reason, cannot be open-sourced and shared freely via Packagist.
There are a few options for hosting these private packages. You can configure your projects’ composer.json
file by adding your packages’ repositories individually. Or, Satis allows you to generate your own static repositories. Alternatively, Toran Proxy allows you to create a self-hosted private version of Packagist, which once set up is much easier to manage than by specifying repositories in your composer.json
.
Gemfury is a PaaS alternative. Aside from the peace-of-mind that comes from a hosted solution – albeit one which comes at a price – one huge advantage is that it supports not just PHP Composer packages, but Ruby Gems, Node.js npm, Python PyPi, APT, Yum and Nu-Get. Great if you have a number of languages under your belt.
Let’s dive in and look at how to use it.
Setting up your Account
To begin with, you’ll need an account. There is a 14-day trial, a free account limited to one collaborator and a single hosted package, as well as a range of other plans starting at US $9 per month.
You can either register using your email address, or with your Github account. Head to the website to sign up.
Creating your First Package
In order to demonstrate how to use Gemfury for private Composer packages, let’s go through the process of creating a package from scratch, which we’ll later submit to the service for use in other projects.
The simplest way to create a new package is to use the following command:
composer init
It will ask you a series of questions; here’s an example transcript of the process:
Welcome to the Composer config generator
This command will guide you through creating your composer.json config.
Package name (<vendor>/<name>) [your-username/package]: your-username/coupon
Description []: Generates a discount coupon code using a super-secret algorithm
Author [Your Name <your-username@example.com>]:
Minimum Stability []: dev
License []: MIT
Define your dependencies.
Would you like to define your dependencies (require) interactively [yes]? yes
Search for a package []: faker
Found 15 packages matching faker
[0] fzaninotto/faker
[1] fzaninotto/faker
[2] bobthecow/faker
[3] tomaj/faker
[4] typo3/faker
[5] yiisoft/yii2-faker
[6] willdurand/faker-bundle
[7] denheck/faker-context
[8] coduo/tutu-faker-extension
[9] davidbadura/faker-bundle
[10] kphoen/faker-service-provider
[11] vegas-cmf/faker
[12] burriko/cake-faker
[13] bit3/faker-cli
[14] emanueleminotto/faker-service-provider
Enter package # to add, or the complete package name if it is not listed []: 0
Enter the version constraint to require []: *
Search for a package []:
Would you like to define your dev dependencies (require-dev) interactively [yes]? no
{
"name": "your-username/coupon",
"description": "Generates a coupon code",
"require": {
"fzaninotto/faker": "*"
},
"license": "MIT",
"authors": [
{
"name": "Your Name",
"email": "your-username@example.com"
}
],
"minimum-stability": "dev"
}
Do you confirm generation [yes]? yes
As you can see, we’re creating a simple package with one dependency, Faker.
Next, let’s add a line to the newly-created composer.json
to tell it where to look for the package’s source code.
"autoload": {
"psr-0": {
"Acme\\": "src/"
}
},
Now let’s create the package itself. We’re going to create a class with a single purpose; to employ a complex, top-secret proprietary algorithm to generate a discount coupon code for an e-commerce platform.
In your working directory, create the src
and src/Acme
directories, then the following file named Coupon.php
:
<?php namespace Acme;
use Faker\Factory;
class Coupon {
public static function generate($percent)
{
$faker = Factory::create();
return sprintf('%s-%s-%d',
strtoupper(date('M')),
strtoupper($faker->word()),
intval($percent));
}
}
Now run composer install
to load our only dependency and to configure the autoloader.
That’s our package built. Now to upload it to Gemfury.
To continue, you’ll need your API key. If you go to your Dashboard, you’ll find it under Settings.
The simplest way to build and upload a package is simply to use Git, and let Gemfury take care of the rest.
Start by creating a .gitignore
file with the following contents:
vendor/
composer.lock
Now initialize the repository:
git init
Add the files:
git add src
git add composer.json
Now we’ll add a Git remote. You can find the relevant URL by selecting Get Started on the Gemfury dashboard, then selecting the “PHP Composer” tab. It will look a little like this:
https://your-username@git.fury.io/your-username/<package-name>.git
Be sure to substitute your-username
with your Gemfury username – if you signed up using Github, it’ll be the same as your Github username – and add it as a remote:
git remote add fury https://your-username@git.fury.io/your-username/coupon.git
At this point, you have two options. The first is to use explicit versioning, where you specify the version in your composer.json
file, like so:
{
"name": "your-username/coupon",
"description": "Generates a coupon code",
"version": "1.0.0",
...
It’s important that you use semantic versioning. Otherwise your package may not build properly; this can also result in some strangely worded error messages.
Alternatively, you can use Git tags. For example, create a new version using a tag as follows:
git tag -a 1.0.0 -m "Version 1.0.0"
Whichever approach you take, the next step is to commit:
git commit -a -m "Initial commit"
Finally, run the following command:
git push fury master --tags
This will push your code up to Gemfury, which will then automatically build it as a package for you.
Now, if you go to your dashboard you should see your new repository listed. Next up, let’s look at how you might use it in a project.
Using a Private Package
If you go back to your dashboard and select “Repos” on the left-hand side, you’ll find your private repo URL. This should remain private, so keep it safe. It’ll look a little like this:
https://php.fury.io/SECRET-CODE/your-username/
It’s the SECRET-CODE
which makes it un-guessable, and therefore effectively private.
Now add it your your project’s composer.json
:
"repositories": [{
"type": "composer",
"url": "https://php.fury.io/SECRET-CODE/your-username/"
}],
You only need add this one repository in order to use any of the private Composer packages you create with Gemfury. No need to add separate repositories each time you want to use a package.
Now you can require
your private packages as if they were on Packagist. Here’s a complete example of a project’s composer.json
:
{
"name": "your-username/my-ecommerce-platform",
"authors": [
{
"name": "Your Name",
"email": "your-username@example.com"
}
],
"repositories": [{
"type": "composer",
"url": "https://php.fury.io/SECRET-CODE/your-username/"
}],
"require": {
"your-username/coupon": "1.0.1"
}
}
Other Approaches
Personally, I believe using Git and tags is the simplest and most effective way to manage your packages.
Alternatively, should you prefer, you can build it yourself by zipping up your package’s source code and uploading it via the Gemfury dashboard.
The Command Line Tool
Gemfury also provides a command-line tool. To install it:
sudo gem install gemfury
To list your packages, you can use the following command:
fury list
Too see the versions of a specific package:
fury versions package-name
For more information about the CLI, visit the relevant section of the documentation.
Summary
In this article I’ve looked at Gemfury, one of a number of options for managing private repositories. As a PaaS solution, it comes without the additional burden of a self-hosted option such as Toran, and it’s simpler to use than Satis. It also has the great benefit of supporting packages across various languages, from PHP Composer packages to Ruby Gems and Node.js npm. Of course, being a PaaS solution, it does come at a cost – but why not try it out using the free trial or the free single-package plan, and see if it works for you.
Frequently Asked Questions (FAQs) about Private Composer Packages and Gemfury
How do I create a private composer package?
Creating a private composer package involves several steps. First, you need to create a new directory for your package and initialize it with a composer.json file. This file will contain all the metadata about your package, such as its name, description, version, and dependencies. You can create this file manually or use the composer init
command to generate it interactively. Once your package is ready, you can publish it to a private repository like Gemfury.
What is Gemfury and how does it work?
Gemfury is a cloud-based package repository that allows you to securely store and share your private packages. It supports several package managers, including Composer for PHP. You can upload your packages to Gemfury using its web interface or command-line tool. Once your package is on Gemfury, you can install it in your projects using the composer require
command, just like any other Composer package.
How do I add a private GitHub repository as a composer dependency?
To add a private GitHub repository as a composer dependency, you need to add it to the repositories section of your composer.json file. The repository should be defined as a vcs (version control system) type and include the URL of the GitHub repository. You also need to provide your GitHub username and token for authentication. Once the repository is added, you can require the package using the composer require
command.
How can I use my private repository in Composer without SSH keys?
If you don’t want to use SSH keys for authentication, you can use a personal access token instead. You can generate a token in your GitHub or Bitbucket account settings. Once you have the token, you can add it to your composer.json file or use it with the composer config
command to set it as a global configuration. This will allow Composer to authenticate with the repository and access your private packages.
What are the benefits of using private composer packages?
Private composer packages allow you to encapsulate and reuse your code across multiple projects. They also provide a way to manage dependencies and ensure that all your projects are using the same versions of libraries. By using a private repository like Gemfury, you can securely share your packages with your team and control who has access to them.
How do I update a private composer package?
To update a private composer package, you need to make your changes in the package’s directory and then update the version number in the composer.json file. Once you’ve done that, you can push your changes to the repository and then update the package in your projects using the composer update
command.
Can I use private composer packages with continuous integration (CI) systems?
Yes, you can use private composer packages with CI systems. You just need to provide the system with the necessary credentials to access your private repository. This can usually be done through environment variables or configuration files.
How do I remove a private composer package?
To remove a private composer package from your project, you can use the composer remove
command followed by the package name. This will remove the package from your composer.json file and uninstall it. If you want to remove the package from your repository, you can do so through the repository’s web interface or command-line tool.
How do I troubleshoot issues with private composer packages?
If you’re having issues with a private composer package, you can use the composer diagnose
command to check for common problems. You can also use the composer show
command to view detailed information about the package. If you’re having issues with authentication, make sure your credentials are correct and that you have access to the repository.
Can I use private composer packages with other package managers?
While Composer is specifically designed for PHP, private packages hosted on repositories like Gemfury can also be used with other package managers that support the same repository format. This includes npm for Node.js, pip for Python, and gem for Ruby. However, the process for using private packages may vary between different package managers.
Lukas is a freelance web and mobile developer based in Manchester in the North of England. He's been developing in PHP since moving away from those early days in web development of using all manner of tools such as Java Server Pages, classic ASP and XML data islands, along with JavaScript - back when it really was JavaScript and Netscape ruled the roost. When he's not developing websites and mobile applications and complaining that this was all fields, Lukas likes to cook all manner of World foods.
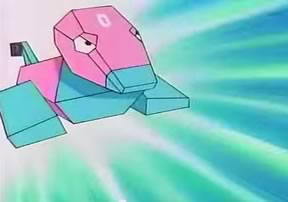
Published in
·APIs·Authentication·CMS & Frameworks·Database·Frameworks·PHP·Web Services·August 26, 2016
Published in
·Accessibility·Community·Patterns & Practices·Statistics and Analysis·UX·Web·March 31, 2014