21 Node.js Interview Questions with Solutions
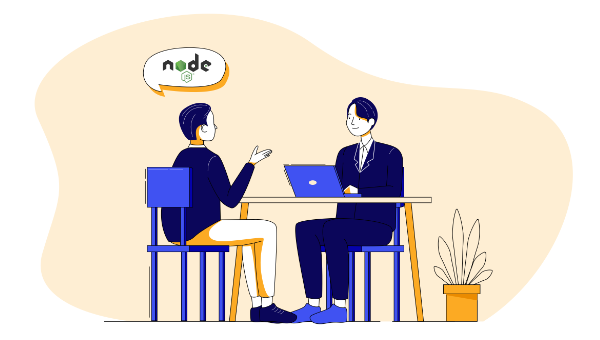
Preparing for a job interview is always a daunting task. Most likely you don’t know exactly what you’ll be asked and nerves can easily take over, making you forget even your own name. I’ve compiled 21 Node.js questions for job interviews that go from very simple stuff to some more technically advanced topics to help you in the process.
Node.js is not exclusively used in the back end. We also use it to create front-end applications, and this has become a very important part of the Web Development ecosystem. This means that it’s very useful for a Node.js developer to be familiar with the role this technology plays in different JavaScript environments. For this reason, I’ve included some questions and answers along those lines.
Key Takeaways
- The article provides 21 Node.js interview questions that range from basic to advanced topics, stressing the importance of understanding Node.js’s role in different JavaScript environments, including both back-end and front-end applications.
- The author advises job seekers to not only focus on answering the questions correctly but also pay attention to the details and ignite a conversation that could potentially turn a stressful experience into a casual chat.
- For interviewers, the questions provided can serve as a starting point for assessing a candidate’s knowledge level. The author also emphasizes the importance of creating a comfortable environment for the interviewee to truly showcase their skills and knowledge.
Guidelines
I would recommend trying to answer the questions yourself before reading the answers. If you didn’t get them all, try again tomorrow to see how much you’ve retained.
There’s also the chance you’re here looking for interview question examples for your candidates. I believe these should be varied enough as a starting point to help you assess their level.
More than just answering a question correctly, I think it’s the details that show how much someone knows. A good answer might ignite a conversation that could potentially render a stressful experience into a casual chat with a colleague. That’s an ideal result for both parties.
Node.js Questions
What is Node.js?
Node.js is a JavaScript runtime environment based on the V8 engine. It allows us to run JavaScript outside of the browser — typically, in a web server.
What is Node.js good for?
Node.js is great at handling multiple connections with low cyclomatic complexity, given that its single-threaded nature requires that we liberate the event loop as soon as possible. This makes Node.js an ideal choice for microservices and real-time applications.
What is npm?
npm stands for Node.js Package Manager. It consists of a command-line interface that we can use to access an online registry of public and private packages.
How do you create a Node.js app from scratch?
We can start by creating a project folder. Then, we navigate to that folder in the command line and run npm init
. Finally, we follow the steps to fill our app information.
What does “npm install” do?
It installs the dependencies found in the package.json
file.
How do you install a library in Node.js?
npm install name-of-the-library
will install our library and include it as a dependency
. If we add the --save-dev
parameter it will be included as a devDependency
.
How do you create a custom script?
We need to go into the package.json
and add our custom script within the scripts
field. We can then run our script by going to the terminal and running npm run name-of-script
.
Is it possible to create a front-end application with Node.js?
The browser can’t run a Node.js application, but you could use something like webpack or Parcel to bundle the code and turn it into something a browser could run. It’s very common nowadays to use a Node.js environment for building front-end applications. A good example of Node.js in the front end is the Electron framework, which makes use of both Node.js and chromium to build “native” apps like, for instance, VS Code.
Can you mention three popular Node.js frameworks?
Express.js is probably the most popular framework to date. Koajs is probably one of the fastest and Sails.js works great for real-time bilateral communication apps given that use socket.io.
What is Express.js good for?
Express.js makes it dead easy to set routes for our web app, which makes it an obvious choice to create REST APIs. It’s quite flexible and easy to use, and its middleware architecture helps to keep a simple and scalable system.
What is Crypto?
Crypto is a Node.js internal library that provides cryptographic functionality to do things like, for example, encrypting and decrypting passwords.
How do we handle local and global scope in Node.js?
Unlike client-side JavaScript, in Node.js variables declared with var
at the highest scope are not global; they’re local to the module they’re in. On the browser, we have access to the window
object where our global variables reside, and Node.js has an object for this called global
.
Does Node.js have access to the file system?
Yes. We can make use of the fs module to read, write, copy, and delete files and folders.
What does non-blocking mean?
It means that a piece of code like, for instance, an asynchronous function, is scheduled to run in the next iteration of the event loop, hence unblocking the rest of the code and allowing it to keep running.
What is the event loop and how does it work?
The event loop is what gives Node.js its asynchronous nature. It schedules the execution of a set of five phases in a loop. The first phase runs the scheduled setTimeout and setInterval callbacks. The second one runs the IO callbacks scheduled to run on the current iteration. The third one polls the events that will be executed in the next iteration. The fourth one runs the setImmediate() callbacks. Finally, the fifth one runs all the “close” callbacks.
Do Asynchronous functions run in parallel?
No. An asynchronous function will execute in the next event loop iteration while a Parallel process runs in its own process or thread.
Is Node.js Multithreaded?
A Node.js process runs in a single thread, but we could use the child_process
module to run multiple processes in parallel or Workers
to run multiple threads.
What is the child_process module?
The child_process module lets us spawn and fork child processes. These are independent processes that run in their own CPU and give us access to system commands.
What’s the difference between a web worker and a worker thread?
Web workers are implemented in the browser and worker threads are implemented in Node.js. They both resolve the same issue, which is to provide parallel processing. In fact, the Worker Thread API is based on the Web Workers implementation.
What are the advantages of using a worker thread vs a child process?
While a child process runs its own process with its own memory space, a worker thread is a thread within a process that can share memory with the main thread. This helps to avoid expensive data serializations back and forth.
What would you use to open a two-way, real-time connection with a client over HTTP?
We could use WebSockets or long polling. There are libraries like soket.io and SignalR that simplify this for us. They even provide clients that fall back to long polling if WebSockets isn’t available in the browser.
Conclusion
We’ve reached the end of the road. I hope you found these questions useful. Could you get them all right? If you couldn’t, don’t worry. Unless you’re aiming for a senior position, you’re not expected to know all of them. Just make sure you grasp the fundamentals, and wherever you find a knowledge gap, make an effort to push your boundaries. I assure you it won’t go unnoticed.
I wish you the best of luck with your interview. Keep calm, trust what you know and be nice — the latter being probably the most important. Most people would rather fill the gaps in the knowledge of a nice, humble person than being in an office every day with an arrogant, selfish individual that is difficult to work with despite them being a genius.
If you’re an interviewer, remember nerves might get in the way of someone showing how good they are. Make them feel as comfortable as possible and let them know you’re on their side and you want them to nail this!
That’s all folks. We’ll be back with a future piece that covers common Node.js interview code challenges, and the skills and mental patterns you’ll need to ace them. See you in the next one!
FAQs About Preparing for a Node.js Job Interview
Preparation involves reviewing Node.js fundamentals, practicing coding challenges, understanding common libraries and frameworks, and being ready to discuss your past projects and experiences.
You should understand asynchronous programming, event-driven architecture, the event loop, callbacks, Promises, error handling, and the core modules of Node.js.
Yes, a strong understanding of JavaScript is crucial, as Node.js is based on JavaScript. You may be asked about closures, hoisting, scoping, and other JavaScript-specific concepts.
Focus on challenges related to asynchronous programming, building RESTful APIs with Express.js, file I/O, and data manipulation with JSON and databases like MongoDB.
Yes, understanding popular libraries and frameworks is essential. Express.js, for example, is commonly used for building web applications and APIs in Node.js.
Be ready to discuss your past projects and experiences. You can explain how you used Node.js to solve specific problems, the architecture of your applications, and any challenges you encountered.
Review sample interview questions related to Node.js, asynchronous programming, and web development.
You might be asked about your experience working in teams, how you handle difficult situations, your problem-solving approach, and your passion for web development and Node.js.
Break down problems into smaller, manageable parts, communicate your thought process clearly, and consider discussing potential trade-offs and optimizations when presenting solutions.
Your enthusiasm for the role, your willingness to learn and adapt, and your ability to communicate effectively are equally important in an interview.
Practice coding exercises, review data structures and algorithms, and focus on time management to complete tasks within the given time frame.
Aside from being a software developer, I am also a massage therapist, an enthusiastic musician, and a hobbyist fiction writer. I love traveling, watching good quality TV shows and, of course, playing video games.