Simple jQuery code snippet to wait for an image to load before running some code. The only drawback is you would need to supply the image name (including extension).
$('#myImage').attr('src', 'image.jpg').load(function() {
//run code
alert('Image Loaded');
});
Frequently Asked Questions (FAQs) about jQuery Image Load
What is the jQuery .load() event and how does it work?
The jQuery .load() event is a powerful tool that triggers a specific function whenever an element, such as an image, has been completely loaded on a webpage. This event is particularly useful when you want to perform certain actions only after an image has fully loaded. For instance, you might want to display a loading animation until an image is fully loaded, and then hide the animation. The .load() event makes this possible. It’s important to note that the .load() event doesn’t work consistently with all elements across all browsers. It works best with elements that have a src attribute, like images.
How can I use jQuery to detect when an image has loaded?
You can use the .load() event in jQuery to detect when an image has loaded. Here’s a simple example:$('img').load(function() {
// Your code here
});
In this example, the code inside the function will run as soon as the image has loaded.
Why isn’t my .load() event firing in jQuery?
There could be several reasons why your .load() event isn’t firing. One common reason is that the image you’re trying to target has already loaded before your script runs. This can happen if your script is located in the head of your HTML document, and the image is located in the body. To avoid this, you can place your script at the end of the body, or use the $(document).ready() function to ensure your script runs only after the entire page has loaded.
Can I use the .load() event with elements other than images?
Yes, you can use the .load() event with any element that has a src attribute. However, it’s important to note that the .load() event doesn’t work consistently with all elements across all browsers. It works best with elements like images.
How can I handle errors with the .load() event in jQuery?
You can handle errors with the .load() event by using the .error() event. This event triggers a function whenever an error occurs while loading an element. Here’s an example:$('img').error(function() {
// Your code here
});
In this example, the code inside the function will run if there’s an error loading the image.
Is it possible to use the .load() event with cached images?
Yes, it’s possible to use the .load() event with cached images. However, keep in mind that if an image is cached, it might load before your script runs. To handle this, you can check if the image is complete using the .complete property. If it’s complete, you can run your function immediately. Otherwise, you can use the .load() event.
How can I use the .load() event to perform an action after multiple images have loaded?
You can use the .load() event in combination with the .promise() method to perform an action after multiple images have loaded. The .promise() method returns a promise that resolves when all actions of a certain type bound to the collection, queued or not, have ended.
Can I use the .load() event in combination with AJAX?
Yes, you can use the .load() event in combination with AJAX. The .load() event can be used to run a function whenever an AJAX request completes. This can be useful if you want to perform an action after data has been loaded from a server.
How can I use the .load() event to load data from a server?
You can use the .load() event to load data from a server by passing a URL as an argument to the .load() method. This will send an AJAX request to the server, and load the data into the selected element.
Is the .load() event deprecated in jQuery?
Yes, the .load() event has been deprecated as of jQuery 1.8 and removed in jQuery 3.0. It’s recommended to use the .on() method instead to attach event handlers.
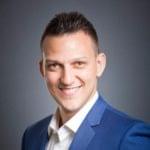
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.