Code snippet to Load PDF into iframe and call print. Also see: 10 JQUERY PRINT PAGE OPTIONS.
jQuery(document).ready(function($) {
function print(url)
{
var _this = this,
iframeId = 'iframeprint',
$iframe = $('iframe#iframeprint');
$iframe.attr('src', url);
$iframe.load(function() {
_this.callPrint(iframeId);
});
}
//initiates print once content has been loaded into iframe
function callPrint(iframeId) {
var PDF = document.getElementById(iframeId);
PDF.focus();
PDF.contentWindow.print();
}
});
Frequently Asked Questions (FAQs) about Loading and Printing PDFs in iFrame
How can I load a PDF into an iFrame using JavaScript?
Loading a PDF into an iFrame using JavaScript is quite straightforward. You can use the ‘src’ attribute of the iFrame to set the source to the PDF file. Here is a simple example:var iframe = document.getElementById('myIframe');
iframe.src = "path/to/your/pdf.pdf";
In this code, ‘myIframe’ is the ID of your iFrame, and “path/to/your/pdf.pdf” is the path to your PDF file. This will load the PDF into the iFrame when the script runs.
How can I print a PDF from an iFrame using JavaScript?
To print a PDF from an iFrame, you can use the ‘contentWindow’ property of the iFrame to access its ‘print’ method. Here is an example:var iframe = document.getElementById('myIframe');
iframe.contentWindow.print();
This code will trigger the print dialog for the PDF loaded in the iFrame when the script runs.
Why is my PDF not loading in the iFrame?
There could be several reasons why your PDF is not loading in the iFrame. One common reason is that the path to the PDF file is incorrect. Make sure that the path in the ‘src’ attribute of the iFrame is correct. Another reason could be that the PDF file is not accessible due to permissions or network issues. Check that the file is accessible and that you have the necessary permissions to access it.
Can I load and print a PDF from a different domain in an iFrame?
Due to the same-origin policy in web browsers, you may face restrictions when trying to load and print a PDF from a different domain in an iFrame. The same-origin policy prevents a document or script from one origin from accessing resources from another origin. However, if you have control over the server hosting the PDF, you can set the appropriate CORS headers to allow access from your domain.
How can I control the print settings when printing a PDF from an iFrame?
Unfortunately, you cannot control the print settings such as the printer, number of copies, or page range when printing a PDF from an iFrame using JavaScript. The print settings are controlled by the user’s browser and cannot be changed by JavaScript for security and privacy reasons.
Can I print a PDF from an iFrame without showing the print dialog?
No, it is not possible to print a PDF from an iFrame without showing the print dialog using JavaScript. This is a security feature of web browsers to prevent websites from sending print jobs without the user’s consent.
Why is the print dialog not showing when I try to print a PDF from an iFrame?
If the print dialog is not showing when you try to print a PDF from an iFrame, it could be due to a JavaScript error. Check the JavaScript console in your browser for any errors. Another reason could be that the ‘print’ method is being called before the PDF has finished loading in the iFrame. Make sure to call the ‘print’ method after the PDF has fully loaded.
Can I load a PDF into an iFrame and print it using jQuery?
Yes, you can load a PDF into an iFrame and print it using jQuery. Here is an example:$("#myIframe").attr("src", "path/to/your/pdf.pdf");
$("#myIframe").get(0).contentWindow.print();
In this code, ‘myIframe’ is the ID of your iFrame, and “path/to/your/pdf.pdf” is the path to your PDF file.
How can I load a PDF into an iFrame using HTML?
To load a PDF into an iFrame using HTML, you can use the ‘src’ attribute of the iFrame to set the source to the PDF file. Here is an example:<iframe id="myIframe" src="path/to/your/pdf.pdf"></iframe>
In this code, ‘myIframe’ is the ID of your iFrame, and “path/to/your/pdf.pdf” is the path to your PDF file.
Can I load and print multiple PDFs in an iFrame?
Yes, you can load and print multiple PDFs in an iFrame. However, you will need to load and print each PDF one at a time. After a PDF has finished printing, you can load the next PDF into the iFrame and print it.
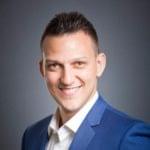
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.