jQuery code snippet to loop through JSON data properties. You have an array of objects/maps so the outer loop loops through those. The inner loop loops through the properties on each object element.
Update: Check this post for 5 in depth examples of jQuery.each().
$.each(data, function() {
$.each(this, function(k, v) {
/// do stuff
});
});
Frequently Asked Questions (FAQs) about jQuery Loop Through JSON Data
How can I loop through a JSON object in jQuery?
Looping through a JSON object in jQuery is quite straightforward. You can use the $.each() function, which is a general function provided by jQuery to iterate over an object’s properties. Here’s a simple example:var jsonObject = { "name": "John", "age": "30", "city": "New York" };
$.each(jsonObject, function(key, value) {
console.log(key + ": " + value);
});
In this example, the $.each() function is used to loop through each property (key-value pair) in the jsonObject. The function takes two parameters: the first is the JSON object, and the second is a callback function that will be executed for each item in the object.
How can I loop through a JSON array in jQuery?
Looping through a JSON array in jQuery is similar to looping through a JSON object. You can use the $.each() function. Here’s an example:var jsonArray = ["John", "Jane", "Doe"];
$.each(jsonArray, function(index, value) {
console.log(index + ": " + value);
});
In this example, the $.each() function is used to loop through each item in the jsonArray. The function takes two parameters: the first is the JSON array, and the second is a callback function that will be executed for each item in the array.
How can I access nested JSON data in jQuery?
Accessing nested JSON data in jQuery can be done by using dot notation or bracket notation. Here’s an example:var jsonObject = {
"person": {
"name": "John",
"age": "30",
"city": "New York"
}
};
console.log(jsonObject.person.name); // Outputs: John
In this example, the nested JSON data (the “name” property) is accessed by using dot notation (jsonObject.person.name).
How can I handle errors when parsing JSON data in jQuery?
You can handle errors when parsing JSON data in jQuery by using a try-catch block. Here’s an example:try {
var jsonObject = $.parseJSON(jsonString);
} catch (e) {
console.error("Parsing error:", e);
}
In this example, if the jsonString is not a valid JSON string, the $.parseJSON() function will throw an error, which will be caught and handled by the catch block.
How can I filter JSON data in jQuery?
You can filter JSON data in jQuery by using the $.grep() function. Here’s an example:var jsonArray = ["John", "Jane", "Doe"];
var result = $.grep(jsonArray, function(value) {
return value !== "John";
});
console.log(result); // Outputs: ["Jane", "Doe"]
In this example, the $.grep() function is used to filter out the item “John” from the jsonArray. The function takes two parameters: the first is the JSON array, and the second is a callback function that will be executed for each item in the array. If the callback function returns true, the item will be included in the result array; if it returns false, the item will be excluded.
How can I convert a JSON string to a JSON object in jQuery?
You can convert a JSON string to a JSON object in jQuery by using the $.parseJSON() function. Here’s an example:var jsonString = '{"name":"John", "age":"30", "city":"New York"}';
var jsonObject = $.parseJSON(jsonString);
console.log(jsonObject); // Outputs: {name: "John", age: "30", city: "New York"}
In this example, the $.parseJSON() function is used to convert the jsonString into a JSON object.
How can I convert a JSON object to a JSON string in jQuery?
You can convert a JSON object to a JSON string in jQuery by using the JSON.stringify() function. Here’s an example:var jsonObject = {name: "John", age: "30", city: "New York"};
var jsonString = JSON.stringify(jsonObject);
console.log(jsonString); // Outputs: '{"name":"John", "age":"30", "city":"New York"}'
In this example, the JSON.stringify() function is used to convert the jsonObject into a JSON string.
How can I use AJAX to load JSON data in jQuery?
You can use the $.ajax() function to load JSON data in jQuery. Here’s an example:$.ajax({
url: "data.json",
dataType: "json",
success: function(data) {
console.log(data);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error("AJAX error: " + textStatus + ' : ' + errorThrown);
}
});
In this example, the $.ajax() function is used to send an AJAX request to the server to load the JSON data from the “data.json” file. If the request is successful, the data will be logged to the console; if there’s an error, the error message will be logged to the console.
How can I use the $.getJSON() function to load JSON data in jQuery?
You can use the $.getJSON() function to load JSON data in jQuery. Here’s an example:$.getJSON("data.json", function(data) {
console.log(data);
}).fail(function(jqXHR, textStatus, errorThrown) {
console.error("getJSON error: " + textStatus + ' : ' + errorThrown);
});
In this example, the $.getJSON() function is used to send an AJAX request to the server to load the JSON data from the “data.json” file. If the request is successful, the data will be logged to the console; if there’s an error, the error message will be logged to the console.
How can I pretty print JSON data in jQuery?
You can pretty print JSON data in jQuery by using the JSON.stringify() function with its second and third parameters. Here’s an example:var jsonObject = {name: "John", age: "30", city: "New York"};
var jsonString = JSON.stringify(jsonObject, null, 2);
console.log(jsonString);
In this example, the JSON.stringify() function is used to convert the jsonObject into a JSON string. The second parameter is a replacer function that alters the behavior of the stringification process, and the third parameter is a number that specifies the number of spaces to use for indentation. By passing null as the second parameter and 2 as the third parameter, the JSON string will be formatted with 2 spaces of indentation, making it easier to read.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
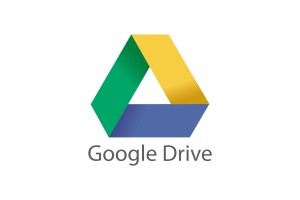
Published in
·APIs·Authentication·CMS & Frameworks·Frameworks·Laravel·Libraries·PHP·Web Services·July 22, 2016