jQuery function to check if horizontal scroll is present – hasHScrollBar() – (or vertical check below also, util function to check if an element has a scrollbar present).
jQuery hasHScrollBar() Function
//util function to check if an element has a scrollbar present
jQuery.fn.hasScrollBar = function(direction)
{
if (direction == 'vertical')
{
return this.get(0).scrollHeight > this.innerHeight();
}
else if (direction == 'horizontal')
{
return this.get(0).scrollWidth > this.innerWidth();
}
return false;
}
//$('#c3 .mbcontainercontent').hasScrollBar('horizontal');
Similar functions:
//util function to check if an element has a horizontal scrollbar present
jQuery.fn.hasHScrollBar = function()
{
// log(this.get(0).scrollWidth);
// log(this.width());
// log(this.innerWidth());
return this.get(0).scrollWidth > this.innerWidth();
}
$('#c3 .mbcontainercontent').hasScrollBar();
//util function to check if an element has a vertical scrollbar present
jQuery.fn.hasVScrollBar = function()
{
// log(this.get(0).scrollHeight);
// log(this.height());
// log(this.innerHeight());
return this.get(0).scrollHeight > this.innerHeight();
}
$('#c3 .mbcontainercontent').hasScrollBar();
Another version…
function hasScroll(el, direction) {
direction = (direction === 'vertical') ? 'scrollTop' : 'scrollLeft';
var result = !! el[direction];
if (!result) {
el[direction] = 1;
result = !!el[direction];
el[direction] = 0;
}
return result;
}
alert('vertical? ' + hasScroll(document.body, 'vertical'));
alert('horizontal? ' + hasScroll(document.body, 'horizontal'));
Frequently Asked Questions (FAQs) about jQuery and Horizontal Scroll
How can I check if a horizontal scroll is present using jQuery?
To check if a horizontal scroll is present using jQuery, you can use the scrollWidth property. This property returns the entire width of an element in pixels, including padding, border, and scroll bar. If the scrollWidth is greater than the clientWidth, it means a horizontal scroll is present. Here’s a simple code snippet:if(document.documentElement.scrollWidth > document.documentElement.clientWidth){
// Horizontal Scroll is present.
}
What is the difference between scrollWidth and clientWidth?
The scrollWidth property returns the entire width of an element in pixels, including padding, border, and scroll bar. On the other hand, clientWidth returns the viewable width of an element in pixels, including padding, but not the border, scrollbar or margin.
Can I use jQuery to check if a vertical scroll is present?
Yes, you can use a similar method to check for a vertical scroll. Instead of comparing scrollWidth and clientWidth, you would compare scrollHeight and clientHeight.
How can I hide the horizontal scroll bar using jQuery?
You can hide the horizontal scroll bar by setting the overflow property to ‘hidden’ using the css() method in jQuery. Here’s a simple code snippet:$("body").css("overflow-x", "hidden");
How can I force a horizontal scroll bar to appear using jQuery?
You can force a horizontal scroll bar to appear by setting the overflow property to ‘scroll’ using the css() method in jQuery. Here’s a simple code snippet:$("body").css("overflow-x", "scroll");
How can I check if a scroll bar is visible using JavaScript?
You can check if a scroll bar is visible using JavaScript by comparing the offsetHeight and clientHeight properties for a vertical scroll bar, or offsetWidth and clientWidth properties for a horizontal scroll bar.
Can I use jQuery to check if a scroll bar is present on a specific element?
Yes, you can use jQuery to check if a scroll bar is present on a specific element. You just need to replace ‘document.documentElement’ with the selector for your specific element in the code snippets provided above.
How can I make a scroll bar always visible using jQuery?
You can make a scroll bar always visible by setting the overflow property to ‘scroll’ using the css() method in jQuery. This will force a scroll bar to appear even if it’s not necessary.
How can I check if a scroll bar is present without using jQuery?
You can check if a scroll bar is present without using jQuery by using pure JavaScript. You can compare the scrollWidth and clientWidth properties for a horizontal scroll bar, or scrollHeight and clientHeight properties for a vertical scroll bar.
How can I control the scroll position using jQuery?
You can control the scroll position using the scrollTop() or scrollLeft() methods in jQuery. These methods set or return the vertical and horizontal scroll position of an element.
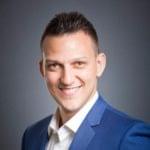
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.