There are a number of different ways to check if a checkbox is checked (ticked). You can use either jQuery or plain JavaScript it doesn’t really matter. Here are 3 examples to wet your appetite. Could be useful when you need to check that a user has ticked a checkbox before submitting a form.
Check if a checkbox IS CHECKED
// First way
$('#checkBox').attr('checked');
// Second way
$('#edit-checkbox-id').is(':checked');
// Third way for jQuery 1.2
$("input[@type=checkbox][@checked]").each(
function() {
// Insert code here
}
);
// Third way == UPDATE jQuery 1.3
$("input[type=checkbox][checked]").each(
function() {
// Insert code here
}
);
Check if a checkbox IS NOT CHECKED
if ($('input[name=termsckb]').attr('checked') != true) {
$('#captcha-wrap').hide();
}
or
if ($('#email_ckb').attr('checked') != true) {
$('#captcha-wrap').hide();
}
Frequently Asked Questions (FAQs) about jQuery Checkbox Checking
How can I check if a checkbox is checked using jQuery?
To check if a checkbox is checked using jQuery, you can use the .is() function with the :checked selector. Here’s an example:if ($('#checkbox_id').is(':checked')) {
// Checkbox is checked.
} else {
// Checkbox is not checked.
}
In this code, ‘#checkbox_id’ is the id of your checkbox. The .is() function returns true if the checkbox is checked and false if it’s not.
How can I check or uncheck a checkbox using jQuery?
To check or uncheck a checkbox using jQuery, you can use the .prop() function. Here’s how you can do it:// To check a checkbox.
$('#checkbox_id').prop('checked', true);
// To uncheck a checkbox.
$('#checkbox_id').prop('checked', false);
In this code, ‘#checkbox_id’ is the id of your checkbox. The .prop() function sets the checked property of the checkbox to either true (checked) or false (unchecked).
How can I toggle a checkbox using jQuery?
To toggle a checkbox using jQuery, you can use the .click() function. Here’s an example:$('#checkbox_id').click();
In this code, ‘#checkbox_id’ is the id of your checkbox. The .click() function simulates a mouse click on the checkbox, which toggles its checked state.
How can I get the value of a checked checkbox using jQuery?
To get the value of a checked checkbox using jQuery, you can use the .val() function. Here’s how you can do it:var value = $('#checkbox_id:checked').val();
In this code, ‘#checkbox_id’ is the id of your checkbox. The .val() function returns the value of the checked checkbox.
How can I handle the change event of a checkbox using jQuery?
To handle the change event of a checkbox using jQuery, you can use the .change() function. Here’s an example:$('#checkbox_id').change(function() {
if ($(this).is(':checked')) {
// Checkbox is checked.
} else {
// Checkbox is not checked.
}
});
In this code, ‘#checkbox_id’ is the id of your checkbox. The .change() function is triggered when the state of the checkbox changes.
How can I select all checkboxes using jQuery?
To select all checkboxes using jQuery, you can use the :checkbox selector. Here’s how you can do it:$('input:checkbox').prop('checked', true);
In this code, ‘input:checkbox’ selects all checkboxes. The .prop() function sets the checked property of all checkboxes to true, which checks them.
How can I deselect all checkboxes using jQuery?
To deselect all checkboxes using jQuery, you can use the :checkbox selector. Here’s an example:$('input:checkbox').prop('checked', false);
In this code, ‘input:checkbox’ selects all checkboxes. The .prop() function sets the checked property of all checkboxes to false, which unchecks them.
How can I enable or disable a checkbox using jQuery?
To enable or disable a checkbox using jQuery, you can use the .prop() function. Here’s how you can do it:// To enable a checkbox.
$('#checkbox_id').prop('disabled', false);
// To disable a checkbox.
$('#checkbox_id').prop('disabled', true);
In this code, ‘#checkbox_id’ is the id of your checkbox. The .prop() function sets the disabled property of the checkbox to either false (enabled) or true (disabled).
How can I check if a checkbox is enabled or disabled using jQuery?
To check if a checkbox is enabled or disabled using jQuery, you can use the .is() function with the :disabled selector. Here’s an example:if ($('#checkbox_id').is(':disabled')) {
// Checkbox is disabled.
} else {
// Checkbox is enabled.
}
In this code, ‘#checkbox_id’ is the id of your checkbox. The .is() function returns true if the checkbox is disabled and false if it’s enabled.
How can I handle the click event of a checkbox using jQuery?
To handle the click event of a checkbox using jQuery, you can use the .click() function. Here’s how you can do it:$('#checkbox_id').click(function() {
if ($(this).is(':checked')) {
// Checkbox is checked.
} else {
// Checkbox is not checked.
}
});
In this code, ‘#checkbox_id’ is the id of your checkbox. The .click() function is triggered when the checkbox is clicked.
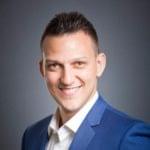
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.