This is how you can use Google Analytics API to get traffic statistics for your website. My original aim was to get live stats and display them on the blog… but as far as I am aware Google Analytics API doesn’t support real-time stats yet… but this is still useful if you want to display pageviews or number of visits on your site and have it automatically update.
In this post
- Setup your Google Analytics API Access
- Frontend JavaScript to call backend script and display data
- Backend PHP Script to connect to Google Analytics API
- Live Working Demo
- Download Package for you to try it yourself
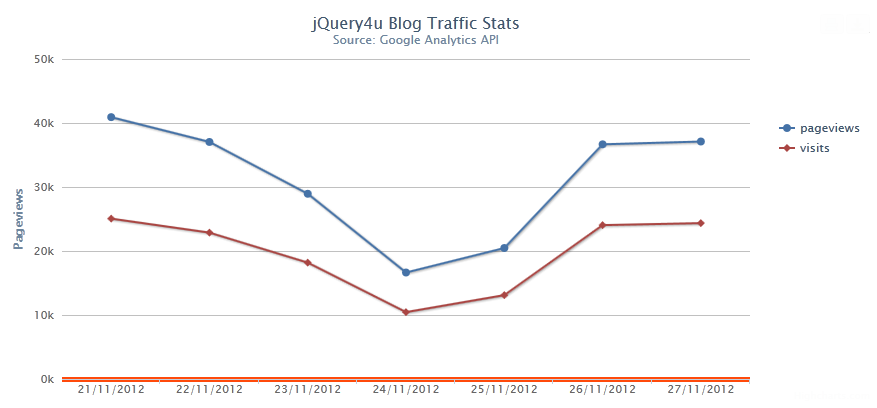
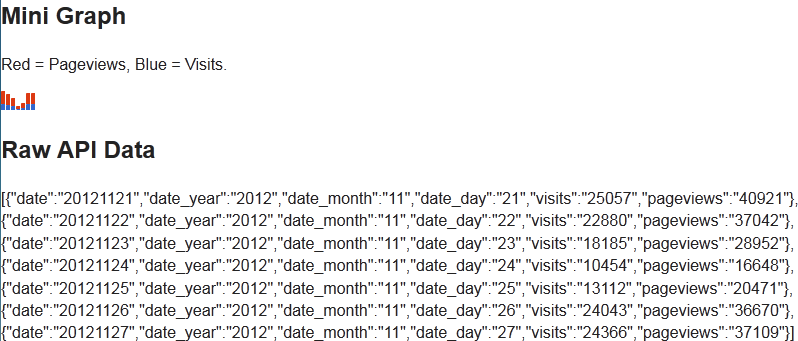
Setup your Google Analytics API Access
This is fairly straight forward and should only take 2 minutes. Go to https://code.google.com/apis/console/. Activate analytics api for your project.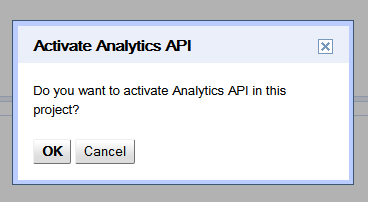
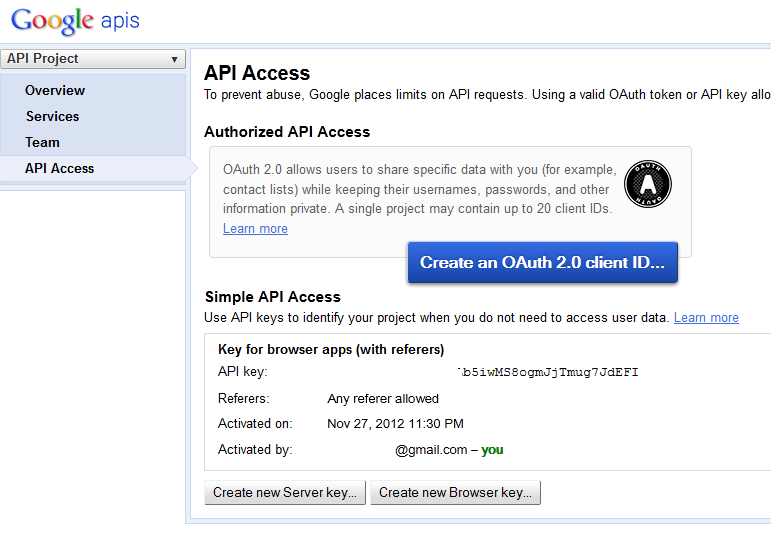
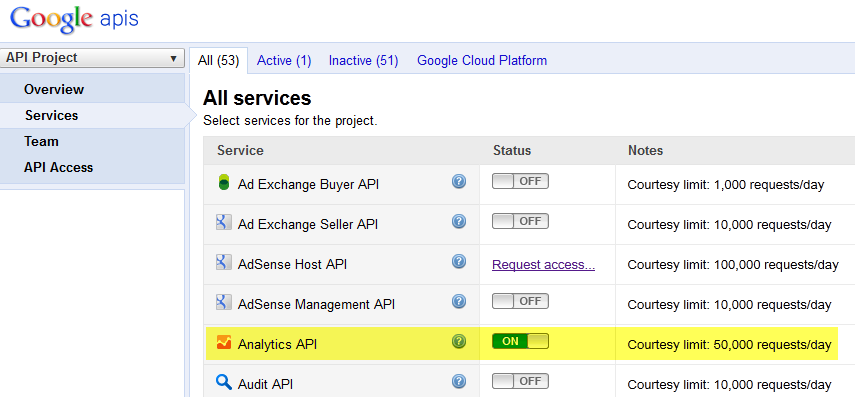
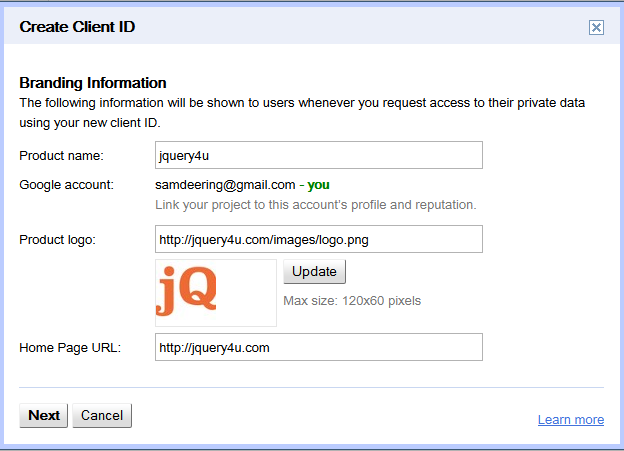
The Frontend Script
This script basically makes an ajax request to the backend script and then parses the JSON data to create the graphs.
/*
Author: Sam Deering 2012
Copyright: jquery4u.com
Licence: MIT.
*/
(function($)
{
$(document).ready(function()
{
//loading image for ajax request
var $loading = $('#galoading');
$.ajaxSetup(
{
start: function()
{
$loading.show();
},
complete: function()
{
$loading.hide();
}
});
$.getJSON('data.php', function(data)
{
// console.log(data);
if (data)
{
$('#results').show();
//raw data
$('#gadata').html(JSON.stringify(data));
//mini graph
var miniGraphData = new Array();
$.each(data, function(i,v)
{
miniGraphData.push([v["visits"], v["pageviews"]]);
});
// console.log(miniGraphData);
$('#minigraph').sparkline(miniGraphData, { type: 'bar' });
//get graph data
var xAxisLabels = new Array(),
dPageviews = new Array(),
dVisits = new Array();
$.each(data, function(i,v)
{
xAxisLabels.push(v["date_day"]+'/'+v["date_month"]+'/'+v["date_year"]);
dPageviews.push(parseInt(v["pageviews"]));
dVisits.push(parseInt(v["visits"]));
});
console.log(xAxisLabels);
console.log(dPageviews);
console.log(dVisits);
var chart = new Highcharts.Chart({
chart: {
renderTo: 'graph',
type: 'line',
marginRight: 130,
marginBottom: 25
},
title: {
text: 'jQuery4u Blog Traffic Stats',
x: -20 //center
},
subtitle: {
text: 'Source: Google Analytics API',
x: -20
},
xAxis: {
categories: xAxisLabels
},
yAxis: {
title: {
text: 'Pageviews'
},
plotLines: [{
value: 0,
width: 5,
color: '#FF4A0B'
}]
},
tooltip: {
formatter: function() {
return ''+ this.series.name +''+
this.x +': '+ this.y.toString().replace(/B(?=(d{3})+(?!d))/g, ",") +' pageviews';
}
},
legend: {
layout: 'vertical',
align: 'right',
verticalAlign: 'top',
x: -10,
y: 100,
borderWidth: 0
},
series: [
{
name: 'pageviews',
data: dPageviews
},
{
name: 'visits',
data: dVisits
}]
});
}
else
{
$('#results').html('error?!?!').show();
}
});
});
})(jQuery);
The Backend PHP Script
This script connects to Google Analytics API and grabs the data associated with your project (website).
< ?php
session_start();
require_once 'src/Google_Client.php';
require_once 'src/contrib/Google_AnalyticsService.php';
$scriptUri = "http://".$_SERVER["HTTP_HOST"].$_SERVER['PHP_SELF'];
$client = new Google_Client();
$client->setAccessType('online'); // default: offline
$client->setApplicationName('[INSERT YOUR APP NAME HERE]');
$client->setClientId('[INSERT YOUR KEY HERE]');
$client->setClientSecret('[INSERT YOUR KEY HERE]');
$client->setRedirectUri($scriptUri);
$client->setDeveloperKey('[INSERT YOUR KEY HERE]'); // API key
// $service implements the client interface, has to be set before auth call
$service = new Google_AnalyticsService($client);
if (isset($_GET['logout'])) { // logout: destroy token
unset($_SESSION['token']);
die('Logged out.');
}
if (isset($_GET['code'])) { // we received the positive auth callback, get the token and store it in session
$client->authenticate();
$_SESSION['token'] = $client->getAccessToken();
}
if (isset($_SESSION['token'])) { // extract token from session and configure client
$token = $_SESSION['token'];
$client->setAccessToken($token);
}
if (!$client->getAccessToken()) { // auth call to google
$authUrl = $client->createAuthUrl();
header("Location: ".$authUrl);
die;
}
try {
//specific project
$projectId = '[INSERT PROJECT ID HERE]'; //see here for how to get your project id: http://goo.gl/Tpcgc
// metrics
$_params[] = 'date';
$_params[] = 'date_year';
$_params[] = 'date_month';
$_params[] = 'date_day';
// dimensions
$_params[] = 'visits';
$_params[] = 'pageviews';
//for more metrics see here: http://goo.gl/Tpcgc
// $from = date('Y-m-d', time()-2*24*60*60); // 2 days ago
// $to = date('Y-m-d'); // today
$from = date('Y-m-d', time()-7*24*60*60); // 7 days ago
$to = date('Y-m-d', time()-24*60*60); // 1 days ago
$metrics = 'ga:visits,ga:pageviews,ga:bounces,ga:entranceBounceRate,ga:visitBounceRate,ga:avgTimeOnSite';
$dimensions = 'ga:date,ga:year,ga:month,ga:day';
$data = $service->data_ga->get('ga:'.$projectId, $from, $to, $metrics, array('dimensions' => $dimensions));
$retData = array();
foreach($data['rows'] as $row)
{
$dataRow = array();
foreach($_params as $colNr => $column)
{
$dataRow[$column] = $row[$colNr];
}
array_push($retData, $dataRow);
}
// var_dump($retData);
echo json_encode($retData);
} catch (Exception $e) {
die('An error occured: ' . $e->getMessage()."n");
}
?>
The script in debug:
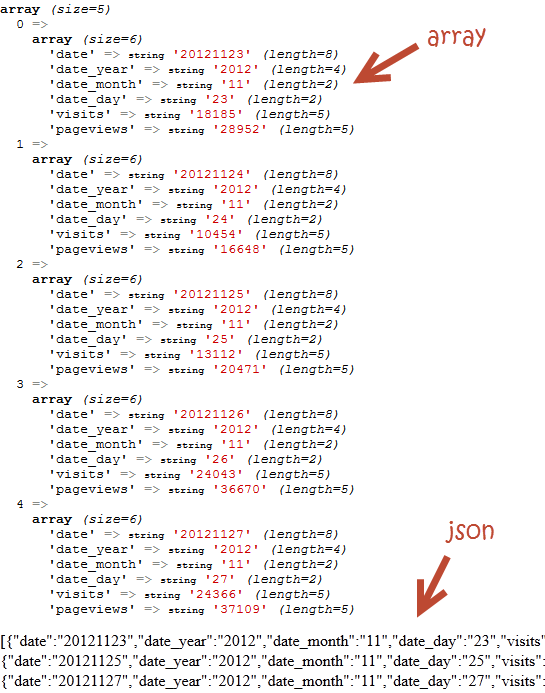
Demo
From time to time, SitePoint removes years-old demos hosted on separate HTML pages. We do this to reduce the risk of outdated code with exposed vulnerabilities posing a risk to our users. Thank you for your understanding.
You’ll need permission to view the demo. I’ll hook up a public demo soon.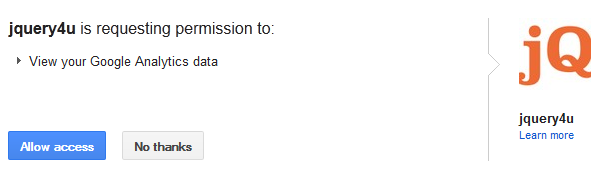
Download
Download Source Files from GitHubFrequently Asked Questions (FAQs) about Google Analytics API
What is the Google Analytics API and how does it work?
The Google Analytics API is a set of programming interfaces that allows developers to interact with Google Analytics data directly. It works by enabling the retrieval of specific types of data from Google Analytics accounts. Developers can use this data to create custom applications or integrate it into existing systems. The API provides access to different types of Analytics data, including user activity, traffic sources, and content performance.
How can I get started with the Google Analytics API?
To get started with the Google Analytics API, you first need to have a Google Analytics account. Once you have an account, you can access the Google Developers Console to create a project and enable the Analytics API. After enabling the API, you’ll need to create credentials which will be used to authenticate your application with Google.
What kind of data can I access with the Google Analytics API?
The Google Analytics API provides access to a wide range of data. This includes user activity data such as sessions, pageviews, and bounce rate; traffic source data like referrals and search keywords; and content performance data such as top landing pages and exit pages. You can also access demographic and geographic data about your users.
How can I use the Google Analytics API to improve my website?
The Google Analytics API can provide valuable insights that can help you improve your website. For example, you can use the API to identify which pages on your site are the most popular, where your traffic is coming from, and what users do on your site. This information can help you make data-driven decisions about how to improve your site.
Can I use the Google Analytics API to track multiple websites?
Yes, the Google Analytics API allows you to track data from multiple websites. Each website you want to track will need to have its own Google Analytics tracking code installed. You can then use the API to retrieve data for each site individually.
Is there a cost associated with using the Google Analytics API?
Google Analytics API is free to use, but there are limits on the amount of data you can request. If you exceed these limits, you may need to pay for additional capacity.
Can I use the Google Analytics API with other Google APIs?
Yes, the Google Analytics API can be used in conjunction with other Google APIs. For example, you could use the Google Maps API to visualize geographic data from your Google Analytics account.
How secure is the data I access with the Google Analytics API?
Google takes data security very seriously. All data accessed through the Google Analytics API is transmitted over HTTPS, a secure protocol. Additionally, you must authenticate your application with Google before you can access any data.
Can I use the Google Analytics API to create custom reports?
Yes, one of the key benefits of the Google Analytics API is the ability to create custom reports. You can use the API to retrieve exactly the data you need, and then use that data to create reports tailored to your specific needs.
What programming languages can I use with the Google Analytics API?
The Google Analytics API is language-agnostic, meaning you can use it with any programming language that can make HTTP requests and handle JSON responses. This includes languages like Python, JavaScript, Java, and many others.
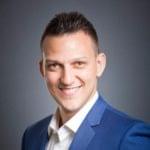
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.