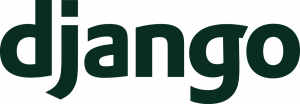
What Do I Need to Get Started?
We will assume that you are an experienced developer and so spend no time in explaining the basics such as installing Django or Python. If you need help with that, please look here. We will also not focus on creating a basic app using Django. Django docs already cover that, if you need help read this article. If you are still reading, I assume you can build a basic Django app, and you now want to map your Rails concepts to Django. Lets get started.You Say MVC, I Say MTV
Rails is an MVC framework. You write your data interactions inapp/models/model_name.rb
, you write your controllers in app/controllers/controller_name.rb
and you render the data using a view from app/views/
. Django is a MVC framework, but it differs from standard MVC terminology.
In Django, the controllers become the views, and views become the template.
So in
Rails: Controller is the ruby code which decides what data gets shown.
Django: View is the Python code which determines what data gets shown.
and
Rails: Views decide how the data is rendered. This can be erb or haml code.
Django: Templates decide how the data is rendered. This is html with Django specific tags.
Django calls this the MTV architecture and has more details about this in their faqs.
At Least the Models Are the Same?
You would hope so, but then life wouldn’t be so much fun. In Railsdb/schema.rb
is the authoritative source for your database schema. To add a new model or to make changes to your existing model, you create and run a migration which changes the schema. The schema.rb
file is auto-generated from the current state of the database. You would generally not edit the schema.rb manually. You keep your model validations and business logic in app/models/model_name.rb
.
In Django, the authoritative source of thie database schema is app_name/models.py
. To create a new table, you edit your models.py and then run either the syncdb
or the migrate
command. You will need the very popular south app for migrate command.
In Rails, the DB is the authoritative source and schema.rb
is autogenerated from it. In Django, models.py
is the authoritative source and DB is synced from it.
Not in a Complicated Relationship
In Rails you would model foreign key relationships viahas_many
, belongs_to
, has_one
, has_and_belongs_to_many
. In Django you specify them as attributes on your models as models.ForeignKey
, models.OneToOneField
, models.ManyToManyField
. In Django you only need to specify one side of the relationship, the other Model object will automatically get the related methods. Here is the mapping.
belongs_to -> ForeignKey
has_many -> (Not needed, as Django automatically add these after getting a ForeignKey)
has_one -> OneToOneField (Only needed to specify on one of the models)
has_and_belong_to_many -> ManyToManyField (Only needed to specify on one of the models)
Model Objects and Querysets.
Once you have defined the model, they have many equivalent methods. Lets say you have a User model available in both Rails and Django. Rails: User.create(..) Django: User.objects.create(..) Saving a model after making some changes Rails:user.save!
Django: user.save()
Django queryset and Rails AR relations are also pretty much equivalent, offering similar methods.
Rails: Model.find(10)
Django: Model.objects.get(id=10)
Rails: Model.where(..conditions)
Django: Model.objects.filter(..conditions)
Rails: Model.order(“createdat”)
Django: Model.objects.orderby(“created_at”)
The big difference is that, in Django, the methods are on a manager. (Generally called objects
), instead of the Model class itself.
Routing and Config
In Rails, the config files are inconfig/*
and each environment, such as development, test, and production, get their own config file. In Django most of the configuration is in settings.py
. Rails puts routing in config/routes.rb
. Django puts rouring/dispatch in urls.py and is regex based.
Templating and Views
Rails views are ERB or haml files. Django templates have template tags, which correspond to Rails helpers and the Django{% include ..%}
template tag corresponds to Rails partials.
Some More Mappings.
Development Server
Rails: rails server Django: python manage.py serverDevelopment Console
Rails: rails console Django: python manage.py shellDatabase Migrations
Rails: rake db:create && rake db:migrate Django: python manage.py syncdb && python manage.py migrateTasks
Django comes with a manage.py script which performs many actions available on rails/rake.Routing
Rails: config/routes.rb Django: urls.pyCallbacks
Rails: callbacks (such as beforesave) Django: signals (Such as presave) or overriding lifecycle methods such as .saveObservers
Rails: observers Django: signalsCustom Tasks
Rails: custom rake tasks Django: Custom management commandsWhat Next?
This article should give you enough pointers to apply your Rails knowledge to start working on Django. This article is specifically not a Rails vs Django post. Rails and Django are both amazing frameworks, and knowing Django can give you valuable insights and ideas to work better with Rails even if you never use Django in production. I’d love to hear your take on the similarities and differences between Rails and Django in the comments section. Thanks for reading!Frequently Asked Questions (FAQs) about Django for Rails Developers
How does Django’s “batteries included” philosophy compare to Rails’ approach?
Django’s “batteries included” philosophy means that it comes with a lot of built-in features that developers might need, such as an admin panel, authentication, and more. This can be a significant advantage for developers who want to get started quickly without having to search for and integrate third-party libraries. On the other hand, Rails follows the principle of convention over configuration, which means it provides a set of sensible defaults that allow developers to get started quickly without having to make a lot of decisions about the infrastructure. Both approaches have their advantages and can be more or less suitable depending on the specific needs of the project.
How does the performance of Django compare to Rails?
Both Django and Rails are high-level web frameworks that prioritize developer productivity and ease of use over raw performance. However, in general, Django is often cited as being slightly faster than Rails. This is largely due to the fact that Python, the language Django is written in, is generally faster than Ruby, the language Rails is written in. However, the performance difference is usually negligible for most web applications, and other factors such as the quality of the code and the efficiency of the database queries can have a much larger impact on performance.
How does the community support for Django compare to Rails?
Both Django and Rails have large and active communities, which means that developers can usually find help and resources easily. However, Rails has been around for a bit longer than Django and has a slightly larger community. This means that there might be more tutorials, libraries, and tools available for Rails. However, Django’s community is also very active and there are plenty of resources available.
How does the learning curve for Django compare to Rails?
Both Django and Rails have a reputation for being relatively easy to learn, especially compared to lower-level frameworks. However, some developers find Django’s “batteries included” philosophy and explicitness to be easier to grasp for beginners, while others prefer Rails’ convention over configuration approach. Ultimately, the learning curve can depend on the individual’s background and personal preferences.
How does Django handle scalability compared to Rails?
Both Django and Rails are capable of powering large-scale, high-traffic websites. However, Django is often cited as being slightly more scalable than Rails, largely due to Python’s superior performance and Django’s more flexible architecture. However, scalability is often more dependent on the infrastructure and database design than the web framework itself, and both Django and Rails can scale well if properly optimized.
How does Django’s ORM compare to Rails’ ActiveRecord?
Django’s ORM and Rails’ ActiveRecord both provide a high-level, abstracted way to interact with the database. However, they have different philosophies. Django’s ORM is more explicit and gives developers more control, while ActiveRecord follows Rails’ convention over configuration philosophy and automates more tasks. Some developers prefer Django’s approach because it gives them more control, while others prefer ActiveRecord’s approach because it simplifies the code.
How does Django’s admin interface compare to Rails’ ActiveAdmin?
Django’s built-in admin interface is one of its most praised features. It provides a ready-to-use, customizable interface for managing the data in your application. Rails, on the other hand, does not come with a built-in admin interface, but there are several third-party libraries available, such as ActiveAdmin. While ActiveAdmin is highly customizable and powerful, some developers prefer Django’s built-in solution because it is simpler to set up and use.
How does Django’s template language compare to Rails’ ERB?
Django’s template language and Rails’ ERB are both powerful and flexible, allowing developers to generate dynamic HTML. However, Django’s template language is a bit more restrictive and enforces a clear separation between logic and presentation, which some developers prefer. ERB, on the other hand, allows Ruby code to be embedded directly into the templates, which can be more flexible but also potentially messier.
How does Django’s routing system compare to Rails’?
Django’s routing system is explicit and requires developers to define all routes in a central location. This can make the routing easier to understand and manage, especially for larger applications. Rails’ routing system, on the other hand, is more implicit and relies on conventions to automatically generate routes. This can make the routing simpler and more intuitive, but also potentially more confusing for complex applications.
How does Django’s support for asynchronous programming compare to Rails’?
Django has built-in support for asynchronous programming, which can be a significant advantage for applications that need to handle long-running tasks or high levels of concurrency. Rails also supports asynchronous programming, but it is not as integral to the framework and requires more manual setup. However, both frameworks can handle asynchronous tasks effectively if properly configured.
This is a post by Shabda Raaj of Agiliq. Their blog talks about many technical topics including Django.