Albacore: Building .NET Applications with Rake
Key Takeaways
- Albacore is a set of extensions to Rake that specifically deals with building .NET-based projects, offering tasks to build, rebuild, and clean projects, run unit tests, copy files and expand templates, and generate assembly info.
- Albacore simplifies the process of building .NET applications, providing a more streamlined build process compared to MSBuild, making it a great choice for developers seeking a simpler, more efficient method.
- The Albacore build system can be easily installed via Ruby gems and integrated into an existing .NET project, allowing developers to compile code, run unit tests, manage AssemblyInfo.cs, and prepare the project for deployment directly from the command line.
What is Albacore?
Albacore is a set of extensions to Rake that deal specifically with building .NET-based projects. It has tasks to build, rebuild, and clean projects, run nunit, xunit, or mstest unit tests, copy files and expand templates, and generate assembly info. .NET does come with it’s own build system called MSBuild. If you’re perfectly happy using this to build your projects, then that’s fine. You probably won’t get much from the rest of this article. If you like the sound of Albacore though and think it might be for you, then read on.Background
First though, a bit of background in case you’re not familiar with Rake (if you do know what Rake is, feel free to skip this section). Rake is Ruby Make, a Ruby build program similar to Make. It revolves around tasks, which live in a Rakefile. A simple example Rakefile looks something like this: [gist id=”2878894″] Here we have 3 tasks:foo
, bar
, and default
. default
has two dependencies: the foo
and bar
tasks. To run Rake, you type rake
at the command-line. It then looks for a Rakefile in the current directory, and runs the default task by default. To run another task, you’d pass the task name as an argument to Rake e.g. rake foo
. Using the example Rakefile above, you can see how the output differs:
[gist id=”2878949″]
Installing Albacore
As Albacore requires Rake (and, thus, Ruby), you’ll need to make sure you have Rake installed first. You probably have, but if you don’t, installing it is easy enough:gem install rake
With Rake installed, all that remains is to install Albacore:
gem install albacore
Once you have Albacore installed, the only other proviso is to make sure you add require 'albacore'
to your Rakefile, otherwise you won’t have access to the Albacore Rake tasks.
Converting an existing project to Albacore
To give you an idea of how you can use Albacore, we’ll create a new project in Visual Studio, add a Rakefile, then add some tasks to handle compiling the project, running unit tests, managing AssemblyInfo.cs, and preparing the project for deployment. We’ll start by creating a default ASP.NET MVC application in Visual Studio. I went File -> New Project, and created a new ASP.NET MVC 4 Web Application (if you don’t have ASP.NET MVC 4 installed, any other ASP.NET web application should work just as well):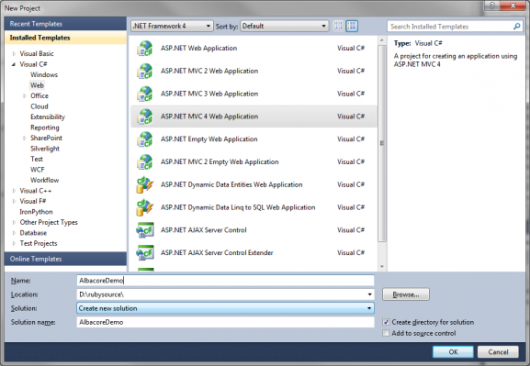
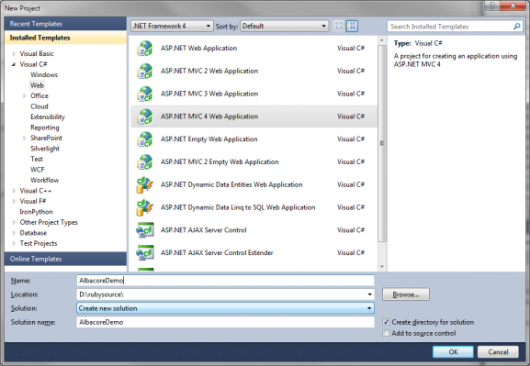
- Create a text file called
Rakefile
in the root folder of your project (you can optionally give it an.rb
file extension if you wish). - Add the following lines:
default
task and set the msbuild
task as a dependency. Then we define the msbuild
task, which will compile our code for us, using the solution and project files already created by Visual Studio. We set the build properties
to use the debug configuration, set which targets we want to call, and tell the msbuild
task where to find the solution file. Note that the path to the sln
file is relative to the location of the Rakefile.
Verify this works by opening the command prompt, changing into your project’s root folder, and typing the following at the command prompt:
$> rake
This calls the default
task, which in turn will call the msbuild
task and compile our project.
- Next, create an mstest task to handle running our unit tests:
msbuild
task, as we won’t be able to run the tests if the project hasn’t been compiled. To check this works, switch back to the command prompt and type the following:
$> rake mstest
You should see the test output, and everything should pass.
- Let’s add a task to populate our AssemblyInfo.cs class with the correct information. First, you’ll need to install the
version_bumper
gem:
$> gem install version_bumper
version_bumper
requires a text file to hold the current version number. Create a text file called VERSION
in the root folder of your project, and add the version number for your project in the format #.#.#.#. Mine looks like this:
[gist id=”2878920″]
Load the version_bumper
using require
, then add the assemblyinfo
task:
[gist id=2878908]
To check this works type the following at the command prompt:
$> rake assemblyinfo
then open up the AlbacoreDemo/Properties/AssemblyInfo.cs file and you should find the relevant properties have been set accordingly:
[gist id=2878899]
We’ll probably want the assemblyinfo
task to run each time we compile our project, so add it as a dependency for the msbuild
task:
[gist id=2878911]
- Finally, let’s add an
output
task that will prepare all the files we’ll need to deploy the site:
out.file
, and the directories we want to copy with out.dir
. Note the line out.file 'Web.Debug.config', :as => 'Web.config'
.
This is similar to web.config transforms, but AFAIK doesn’t actually run the transforms; it just copies the file. Depending on how reliant you are on web.config transforms, YMMV with this, and you might get better results adding the config transforms as another target in your msbuild
task.
- Now we’ve added the tasks we need, we can tidy them up a bit by adding
a
configure
block:
msbuild
. The corresponding lines in the msbuild
and mstest
tasks can be removed.
The full Rakefile now looks like this. Note that we’ve changed the default
task so that it builds, tests, and gets our site ready for deployment:
[gist id=”2878918″]
Albacore is available on GitHub. The wiki has extensive documentation on all supported tasks, so is a good place to find out more info. It also contains a link to some projects using Albacore in real life, so you can see how it’s being used in production.
Hopefully this has given you a taster for what you can do with Albacore, and how you could use it to start building existing .NET projects.
Frequently Asked Questions (FAQs) about Albacore: Building .NET Applications with Rake
What is Albacore and how does it help in building .NET applications?
Albacore is a suite of Rake tasks that simplifies the process of building .NET applications. It provides a set of pre-defined tasks that automate the build process, making it easier and more efficient. These tasks include compiling code, running unit tests, creating assemblies, and deploying applications. Albacore is designed to work with the .NET framework and supports multiple .NET languages, including C#, VB.NET, and F#.
How does Albacore compare to other build tools like MSBuild?
While MSBuild is a powerful tool, it can be complex and difficult to use, especially for large projects. Albacore, on the other hand, is designed to be simple and easy to use. It uses a Ruby-based DSL (Domain Specific Language) for defining tasks, which is more readable and easier to understand than the XML-based syntax used by MSBuild. This makes Albacore a great choice for developers who want a simpler, more streamlined build process.
Can I use Albacore with other Ruby tools?
Yes, Albacore is designed to work seamlessly with other Ruby tools. For example, you can use it with Bundler to manage your project’s dependencies, or with RSpec for testing. This makes Albacore a versatile tool that can fit into a variety of development workflows.
How do I install Albacore?
Albacore is distributed as a Ruby gem, so you can install it using the gem install
command. Once installed, you can require it in your Rakefile to start using its tasks.
How do I define tasks with Albacore?
Defining tasks with Albacore is straightforward. You simply use the task
method, followed by the name of the task and a block of code that defines what the task should do. For example, to define a task that compiles a C# project, you might write:task :compile do
csc = CSC.new
csc.compile
end
Can I use Albacore with non-.NET languages?
While Albacore is designed for .NET, it’s flexible enough to be used with other languages. However, you may need to write custom tasks or use additional tools to fully support non-.NET languages.
How do I run tasks with Albacore?
To run a task with Albacore, you simply use the rake
command followed by the name of the task. For example, to run the compile
task defined above, you would type rake compile
at the command line.
Can I use Albacore on non-Windows platforms?
Yes, Albacore is platform-agnostic and can be used on any platform that supports Ruby. This includes Linux, macOS, and Windows.
How do I handle errors with Albacore?
Albacore provides several ways to handle errors. For example, you can use the fail
method to stop a task if an error occurs, or the rescue
method to catch and handle exceptions.
Can I extend Albacore with my own tasks?
Yes, Albacore is designed to be extensible. You can define your own tasks using the task
method, or create custom task classes that inherit from the Albacore::Task
class. This allows you to add your own functionality to Albacore and tailor it to your specific needs.
Ian Oxley has been building stuff on the Web professionally since 2004. He lives and works in Newcastle-upon-Tyne, England, and often attends local user groups and meetups. He's been known to speak at them on occasion too. When he's not in front of a computer Ian can be found playing guitar, and taking photos. But not usually at the same time.