There are heaps of form validation plugins out there at the moment. So today we are sharing you what we think are 5 really Good jQuery Validate Form Demos. jQuery powered ofcourse! Enjoy =) Also see: BASIC JQUERY FORM VALIDATION EXAMPLE (2MINS)
1. jQuery Validation Plugin
This jQuery plugin makes simple clientside form validation easy, whilst still offering plenty of customization options.
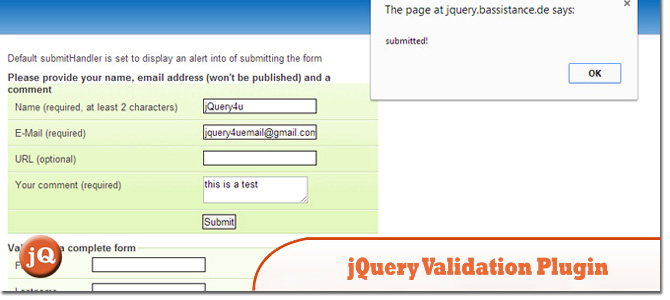
2. jQuery Validation Plugin – ThemeRollered demo
ThemeRollered demo.
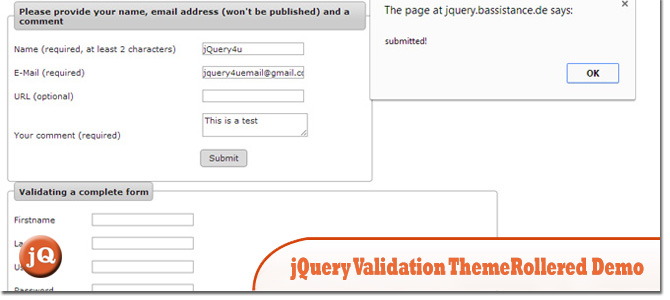
3. jQuery Validate Demo
With styles from Twitter Bootstrap
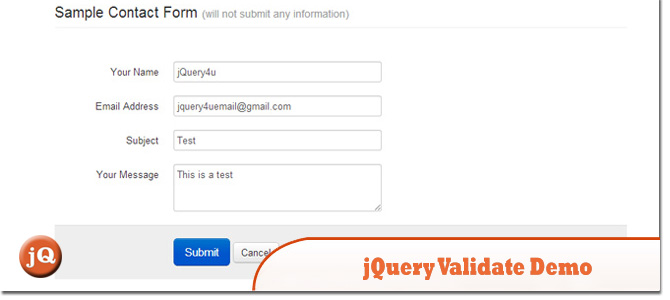
4. Basic jQuery Validation Form Demo
This demo shows you how easy validation your forms can be using a little jQuery. It uses the jQuery validation plugin and some basic input validation rules.
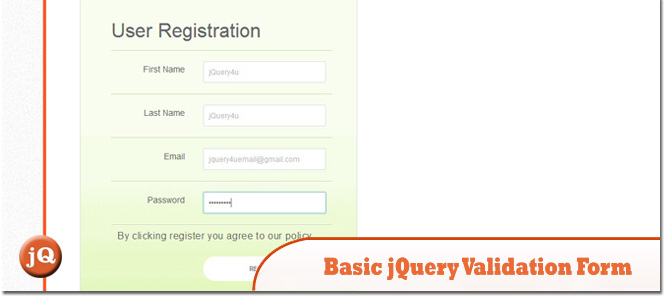
5. Ajax Form Validation Example
This shows two examples of client side validation in a form using JavaScript (with jQuery).
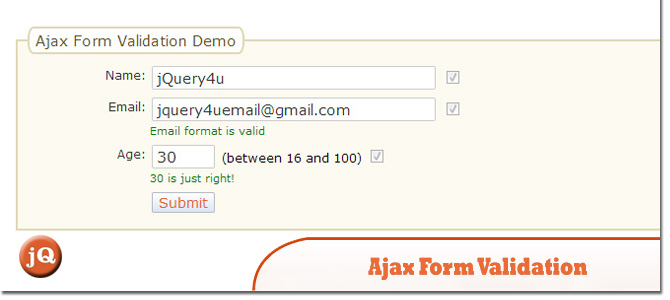
Frequently Asked Questions (FAQs) about jQuery Form Validation
How can I customize the error messages in jQuery form validation?
Customizing error messages in jQuery form validation is quite simple. You can use the messages
option in the validate
method to set your own error messages. For each form field, you can specify the error message for each validation rule. For example:$("#myForm").validate({
rules: {
name: {
required: true,
minlength: 2
}
},
messages: {
name: {
required: "Please enter your name",
minlength: "Your name must consist of at least 2 characters"
}
}
});
In this example, if the ‘name’ field is empty, the message “Please enter your name” will be displayed. If the ‘name’ field has less than 2 characters, the message “Your name must consist of at least 2 characters” will be displayed.
Can I validate an email address using jQuery form validation?
Yes, jQuery form validation provides a built-in method for email validation. You can use the email
rule to validate an email address. Here’s an example:$("#myForm").validate({
rules: {
email: {
required: true,
email: true
}
},
messages: {
email: {
required: "Please enter your email address",
email: "Please enter a valid email address"
}
}
});
In this example, if the ’email’ field is empty, the message “Please enter your email address” will be displayed. If the ’email’ field does not contain a valid email address, the message “Please enter a valid email address” will be displayed.
How can I validate a form on submit using jQuery form validation?
jQuery form validation automatically validates the form when the submit event is triggered. You just need to call the validate
method on the form when the page is loaded. Here’s an example:$(document).ready(function() {
$("#myForm").validate({
rules: {
name: {
required: true,
minlength: 2
},
email: {
required: true,
email: true
}
},
messages: {
name: {
required: "Please enter your name",
minlength: "Your name must consist of at least 2 characters"
},
email: {
required: "Please enter your email address",
email: "Please enter a valid email address"
}
}
});
});
In this example, the form with the id ‘myForm’ will be validated when the submit button is clicked. If the form fields do not meet the validation rules, the corresponding error messages will be displayed and the form will not be submitted.
Can I use jQuery form validation with AJAX?
Yes, you can use jQuery form validation with AJAX. You can use the submitHandler
option in the validate
method to handle the form submission using AJAX. Here’s an example:$("#myForm").validate({
rules: {
name: {
required: true,
minlength: 2
},
email: {
required: true,
email: true
}
},
messages: {
name: {
required: "Please enter your name",
minlength: "Your name must consist of at least 2 characters"
},
email: {
required: "Please enter your email address",
email: "Please enter a valid email address"
}
},
submitHandler: function(form) {
$.ajax({
type: "POST",
url: "/submit",
data: $(form).serialize(),
success: function(response) {
// handle the response
}
});
return false; // prevent the form from submitting normally
}
});
In this example, when the form is valid and the submit button is clicked, the form data will be sent to the server using AJAX.
How can I validate a checkbox using jQuery form validation?
You can use the required
rule to validate a checkbox. If the checkbox is not checked, an error message will be displayed. Here’s an example:$("#myForm").validate({
rules: {
agree: {
required: true
}
},
messages: {
agree: {
required: "You must agree to the terms"
}
}
});
In this example, if the ‘agree’ checkbox is not checked, the message “You must agree to the terms” will be displayed.
How can I validate a select field using jQuery form validation?
You can use the required
rule to validate a select field. If no option is selected, an error message will be displayed. Here’s an example:$("#myForm").validate({
rules: {
country: {
required: true
}
},
messages: {
country: {
required: "Please select your country"
}
}
});
In this example, if no option is selected in the ‘country’ select field, the message “Please select your country” will be displayed.
How can I validate multiple fields with the same name using jQuery form validation?
You can use the required
rule with a function to validate multiple fields with the same name. The function should return true if at least one field is filled in. Here’s an example:$("#myForm").validate({
rules: {
"options[]": {
required: function() {
return $("input[name='options[]']:checked").length == 0;
}
}
},
messages: {
"options[]": {
required: "Please select at least one option"
}
}
});
In this example, if no ‘options’ checkboxes are checked, the message “Please select at least one option” will be displayed.
How can I validate a file input using jQuery form validation?
You can use the required
rule to validate a file input. If no file is selected, an error message will be displayed. Here’s an example:$("#myForm").validate({
rules: {
file: {
required: true
}
},
messages: {
file: {
required: "Please select a file"
}
}
});
In this example, if no file is selected in the ‘file’ input, the message “Please select a file” will be displayed.
How can I validate a number using jQuery form validation?
You can use the number
rule to validate a number. If the field does not contain a valid number, an error message will be displayed. Here’s an example:$("#myForm").validate({
rules: {
age: {
required: true,
number: true
}
},
messages: {
age: {
required: "Please enter your age",
number: "Please enter a valid number"
}
}
});
In this example, if the ‘age’ field is empty, the message “Please enter your age” will be displayed. If the ‘age’ field does not contain a valid number, the message “Please enter a valid number” will be displayed.
How can I validate a date using jQuery form validation?
You can use the date
rule to validate a date. If the field does not contain a valid date, an error message will be displayed. Here’s an example:$("#myForm").validate({
rules: {
birthdate: {
required: true,
date: true
}
},
messages: {
birthdate: {
required: "Please enter your birthdate",
date: "Please enter a valid date"
}
}
});
In this example, if the ‘birthdate’ field is empty, the message “Please enter your birthdate” will be displayed. If the ‘birthdate’ field does not contain a valid date, the message “Please enter a valid date” will be displayed.
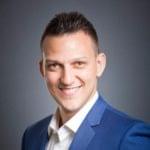
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.