Solidity for Beginners: A Guide to Getting Started
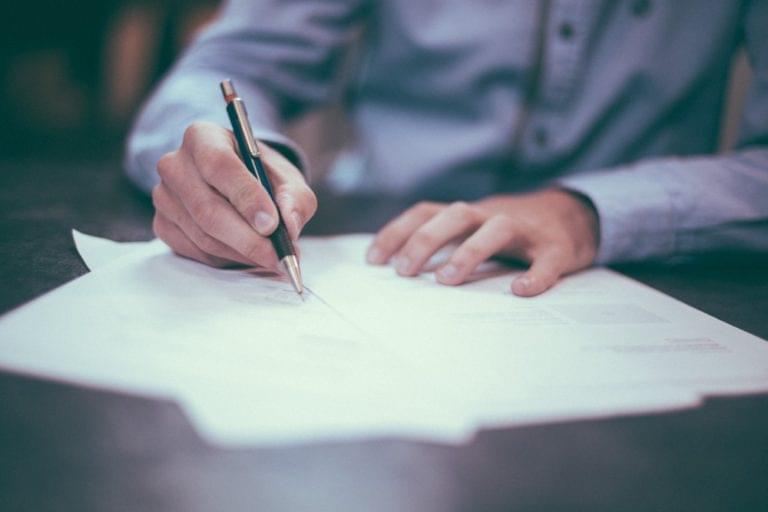
Solidity, a smart contracts programming language, has been a buzzword for quite some time now. This tutorial is meant for beginners ready to explore Solidity and code smart contracts. Before we begin, we’ll get the basics clear. We will begin by exploring smart contracts and their effectiveness, gradually moving on to Solidity itself. Let’s get started!
Key Takeaways
- Solidity is a programming language designed specifically for writing smart contracts on Ethereum, a blockchain-based platform. It’s a simple language with functionalities similar to C and JavaScript, and it supports state variables, data types, and programming functions.
- Smart contracts, or crypto-contracts, are self-executing computer programs that enable digital transactions, business, currency exchanges, asset transfers, and more according to a predefined set of conditions called a smart contract protocol. They function over a given blockchain network and ensure that both parties are following the rules and conditions of the contract.
- While smart contracts theoretically can solve complex issues of trust, transparency, and execution between contract parties, their practical applications are currently limited. This is due to their inherent lack of intelligence and the complexity involved in coding them.
- To test a smart contract on a local system, one can install a test environment like Ethereum TestRPC. This creates a ‘fake’ node at the user site and compiles the Solidity code, enabling the user to test their code without actually having to spend Ether coins.
What You’ll Learn
By the end of this tutorial, you’ll learn;
- The basics of smart contracts
- The basics of Solidity
- Basic coding in Solidity
- The Applications of Solidity
What Are Smart Contracts?
The term smart contracts has created a lot of hype, and it still remains in the spotlight right next to terms like artificial intelligence, blockchain, Machine Learning, and so on. Smart contracts, or crypto-contracts, are nothing but self-executing computer programs that enable digital transactions, business, currency exchanges, asset transfers, and more according to a predefined set of conditions called a smart contract protocol. Smart contracts not only ensure that both the parties are following the rules and conditions of the contract, but also enforce the contract obligations automatically.
How do smart contracts function?
Smart contracts function over a given blockchain network. A smart contract takes the information as an input, assigns a value to that input according to the coded contract rules and conditions, then executes those actions according to the clauses. For example, during an ICO, a smart contract may decide whether the cryptocoin will go to the buyer or the token generator.
All smart contracts run atop a blockchain layer, where all the data is stored with Proof of Work or Proof of Stake algorithms.
Smart contract applications
Smart contracts are handy tools that allow asset transfer and conditional fulfillment digitally. They solve the issue of mutual trust, transparency, global communication and economics. Here are some revolutionary real-world applications of smart contracts:
- The execution of transactions including legal processes.
- The execution of transactions relating to the insurance industry.
- The execution of crowdfunding agreements and ICO campaigns.
- The execution of transactions related to trading, financial derivatives, and simple asset exchange and transfers.
Smart contracts hold potential to disrupt industries which call for expertise and repetitive processes — for example, legal procedures. They also possess a potential for real-time auditing operations (which take many working hours if done manually!) and risk assessment, thereby enforcing compliance with the set norms.
Can smart contracts do anything?
The proposition offered by this unique blockchain tool is tempting. Theoretically, smart contracts can be used to solve complex issues of trust, transparency, and execution between the contract parties while ensuring compliance and risk management. However, the majority of smart contract applications are just theoretical. There are projects currently under development that are exploring uses for smart contracts, but the truth is we have found limited current practical applications.
Smart contracts may not be able to do everything they promise theoretically, at least currently. Here are the primary reasons;
Smart contracts are not actually ‘smart’. Smart coded contracts are not inherently smart or intelligent. They are called ‘smart’ because of the automation and condition enforcement that is coded into them, and because there is no need for a third-party intervention. But in reality, they cannot judge circumstances and play by the ‘code is law’ principle. While this can be useful, it can be harmful as well. For example, conditions cannot be changed or tampered with in the contract, even if both the parties mutually agree to a condition outside the agreement. Smart contracts just execute mutually agreed-upon conditions and follow the code in any state.
Creating smart contracts is highly complicated and tedious. Another reason why practical applications for smart contracts are scarce is the sophisticated engineering involved in coding them. Smart contracts are supposed to be Turing complete, i.e. the smart contract can simulate any Turing machine. For that, it has to be highly complicated, which in turns makes them difficult to analyze. The catch is security. If a smart contract has bugs, it can be breached and there is no point in using it. Analyzing a Turing-complete smart contract becomes a herculean task. Even ordinary contracts take years of expertise and consistent builds and fixes to meet user requirements.
What is Solidity?
Solidity is a rather simple language deliberately created for a simplistic approach to tackle real world solutions. Gavin Wood initially proposed it in the August of 2014. Several developers of the Ethereum chain such as Christian Reitwiessner, Alex Beregszaszi, Liana Husikyan, Yoichi Hirai and many more contributed to creating the language. The Solidity language can be executed on the Ethereum platform, that is a primary Virtual Machine implementing the blockchain network to develop decentralized public ledgers to create smart contract systems.
The language has the same functionalities as C and JavaScript. Additionally, the language supports state variables (similar to objects in Object Oriented Programming), data types, and programming functions. However, the language faces consistent upgrades and rapid changes throughout the platform. Hence as a programmer, you need to pay attention to the updates through Ethereum platform forums, blogs, and chat rooms.
Both Solidity and Ethereum are under proactive development.
How do I learn Solidity?
The syntactical symmetry of Solidity with ECMAScript (JavaScript) has considerably enhanced the usability of the system. The Ethereum design documentation brings out the stack and memory model with a 32-byte instruction word size. This Ethereum Virtual Machine creates the program stack that keeps track of program counter and data storage registers. The program counter loops or jumps to maintain a sequential flow to control the program.
The virtual memory also gives temporary memory for data allocation that is somewhat expandable, compared to the permanent storage allocation contributed by the nodes of the blockchain. The language is created to bring preciseness and determinism to smart contracts. To “mine” a block on Ethereum, the node executes smart contracts and programming methods within the corresponding blocks.
This means the code being executed is scheduled according to the flow of block. The new stage upon execution changes the phase of the program to new storage spaces or transactions. These transactions are initially made at the site of the miner. Once executed, the new block is then propagated to other acting nodes of the blockchain.
To verify the authenticity of the smart contract system each node independently verifies the block by comparing the state changes with the available local copy of the block. If the state changes are deterministic, the transactions are approved by the participating nodes of the blockchain. Consequently, in a situation where the nodes couldn’t come to a consensus, the execution of the block halts and the network may halt.
For the continuous execution of smart contracts on a blockchain network, the contract must be deterministic, where the nodes continuously validate the conditions to be met for the smart contract.
Solidity Basics
To get started with the language and learn the basics let’s dive into the coding. We will begin by understanding the syntax and general data types, along with the variable data types. Solidity supports the generic value types, namely:
Booleans: Returns value as either true or false. The logical operators returning Boolean data types are as follows:
!
Logical negation&&
logical conjunction, “and”||
logical disjunction, “or”==
equality!=
inequality
Integers: Solidity supports int
/unit
for both signed and unsigned integers respectively. These storage allocations can be of various sizes. Keywords such as uint8
and uint256
can be used to allocate a storage size of 8 bits to 256 bits respectively. By default, the allocation is 256 bits. That is, uint
and int
can be used in place of uint256
and int256
. The operators compatible with integer data types are:
- Comparisons:
<=
,<
,==
,!=
,>=
,>
. These are used to evaluate tobool
. - Bit operators:
&
,|
,^
bitwise exclusive ‘or’,~
bitwise negation, “not”. - Arithmetic operators:
+
,-
, unary-
, unary+
,*
,/
,%
remainder,**
exponentiation,<<
left shift,>>
right shift.
The EVM returns a Runtime Exception when the modulus operator is applied to the zero of a “divide by zero” operation.
Address: An address can hold a 20 byte value that is equivalent to the size of an Ethereum address. These address types are backed up with members that serve as the contract base.
String Literals: String literals can be represented using either single or double quotes (for example, "foo"
or 'bar'
). Unlike in the C language, string literals in Solidity do imply trailing value zeroes. For instance, “bar” will represent a three byte element instead of four. Similarly, in the case of integer literals, the literals are convertible inherently using the corresponding fit, that is, byte or string.
Modifier: In a smart contract, modifiers are used to ensure the coherence of the conditions defined before executing the code.
Solidity provides basic arrays, enums, operators, and hash values to create a data structure known as “mappings.” These mappings are used to return values associated with a given storage location. An Array is a contiguous memory allocation of a size defined by the programmer where if the size is initialized as K, and the type of element is instantiated as T, the array can be written as T[k]
.
Arrays can also be dynamically instantiated using the notation uint[][6]
. Here the notation initializes a dynamic array with six contiguous memory allocations. Similarly, a two dimensional array can be initialized as arr[2][4]
, where the two indices point towards the dimensions of the matrix.
We will begin our programming venture with a simple structure of a contract. Consider the following code:
pragma solidity^0.4.0;
contract StorageBasic {
uint storedValue;
function set(uint var) {
storedValue= var;
}
function get() constant returns (uint) {
return storedValue;
}
}
In the above program, the first line of the code declares the source code to be written in Solidity’s version 0.4.0. The code will therefore be compatible with the corresponding EVM or any other superior version.
Such a declaration is essential to ensure that the program executes as expected with all compatible versions of the compiler. The word “Pragma” refers to the instructions given to a compiler to sequentially execute the source code.
Solidity is statically typed language. Therefore, each variable type irrespective of their scope can be instantiated at compile time. These elementary types can be further combined to create complex data types. These complex data types then synchronize with each other according to their respective preferences.
Compiling and testing our smart contract
To test your smart contract on a local system, you need to install a test environment that is easy to use and accept simple Truffle commands. You can use the Ethereum TestRPC for that. It is basically a slim code that creates a ‘fake’ node at the user site and compiles your Solidity code using an 8545 port. The compiler converts the source code to the EVM code, thereby enabling you to test your code without actually having to spend your Ether coins. Here are a few quick install commands:
npm i -g ethereum-testrpc
testrpc -m "This is sample code check check"
To initiate the compile command in Truffle, we use the following command:
truffle compile
Once the source code is successfully compiled the console returns a “Saving artifacts” message. Otherwise, it returns the compilation errors for your code.
Once you are familiar with the idea of smart contracts, go ahead and learn DApp designs over the Ethereum network.
Smart contracts have created a new world of opportunities for online businesses and blockchain innovations. The technology gave birth to Blockchain 2.0 by creating a decentralized ledger to verify and facilitate digital negotiations and unfold a contract. Solidity is the language that will build up the contract systems to their true potential and beyond.
Being a beginner, this article gives you an overview of the programming rules involved in the language and how you can use these basics to create your own smart contract systems.
Frequently Asked Questions about Solidity for Beginners
What is the best way to start learning Solidity?
The best way to start learning Solidity is by understanding the basics of blockchain technology and Ethereum. Once you have a good grasp of these concepts, you can start learning Solidity by reading tutorials, watching video lessons, and practicing coding. It’s also beneficial to join online communities and forums where you can interact with other learners and experienced developers. Remember, practice is key when learning a new programming language.
Can I learn Solidity without any prior programming experience?
While it’s possible to learn Solidity without any prior programming experience, it can be quite challenging. Solidity is a statically-typed programming language used for developing smart contracts on Ethereum. Having a basic understanding of programming concepts like variables, functions, and control structures can make the learning process easier. If you’re completely new to programming, you might want to start with a more beginner-friendly language like Python or JavaScript.
What are the key features of Solidity?
Solidity is a contract-oriented, high-level language whose syntax is similar to that of JavaScript. It is statically typed, supports inheritance, libraries, and complex user-defined types. It is designed to target the Ethereum Virtual Machine (EVM), making it a popular choice for developing decentralized applications (dApps) and smart contracts on the Ethereum blockchain.
How long does it take to learn Solidity?
The time it takes to learn Solidity can vary greatly depending on your prior programming experience and how much time you can dedicate to learning. If you’re already familiar with programming concepts and have experience with languages like JavaScript, you might be able to learn the basics of Solidity in a few weeks. However, mastering Solidity and becoming proficient in developing smart contracts can take several months or more.
What are some common mistakes beginners make when learning Solidity?
Some common mistakes beginners make when learning Solidity include not fully understanding the Ethereum blockchain before starting with Solidity, not testing their smart contracts thoroughly, and not keeping up with the latest updates and changes in the Solidity language and the Ethereum ecosystem. It’s important to have a solid foundation in blockchain technology, to test your code rigorously, and to stay updated with the latest developments.
Are there any good resources for learning Solidity?
There are many great resources available for learning Solidity. The official Solidity documentation is a great place to start. It provides a comprehensive overview of the language and its features. Other resources include online tutorials, video courses, and books. Websites like Stack Overflow and GitHub can also be useful for finding solutions to specific problems and examples of real-world smart contracts.
How can I practice my Solidity skills?
The best way to practice your Solidity skills is by writing and testing your own smart contracts. You can start by creating simple contracts and gradually move on to more complex ones. There are also many online platforms that provide coding challenges and projects that can help you practice and improve your skills.
What are the job prospects for a Solidity developer?
As the popularity of Ethereum and blockchain technology continues to grow, so does the demand for Solidity developers. Solidity developers are needed to create and maintain smart contracts and decentralized applications on the Ethereum blockchain. They can find job opportunities in a wide range of industries, including finance, healthcare, supply chain, and more.
What are smart contracts and how do they work in Solidity?
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They automatically execute transactions when predefined conditions are met. In Solidity, smart contracts are like classes in object-oriented languages. They contain data in the form of state variables and functions that can modify these variables.
What tools do I need to start coding in Solidity?
To start coding in Solidity, you’ll need a text editor or an integrated development environment (IDE) like Remix, which is a web-based IDE with built-in static analysis, test blockchain, and debugging tools. You’ll also need a Solidity compiler to convert your Solidity code into a format that the Ethereum Virtual Machine (EVM) can understand.
Anthony Bergs is a Digital Marketing Specialist and a Project Manager at Writers Per Hour (https://www.writersperhour.com). He always keeps an eye on the marketing sector to implement the best innovations into the strategies that he builds. He’s always open for new connections and partnerships.