Destructuring Objects and Arrays in JavaScript
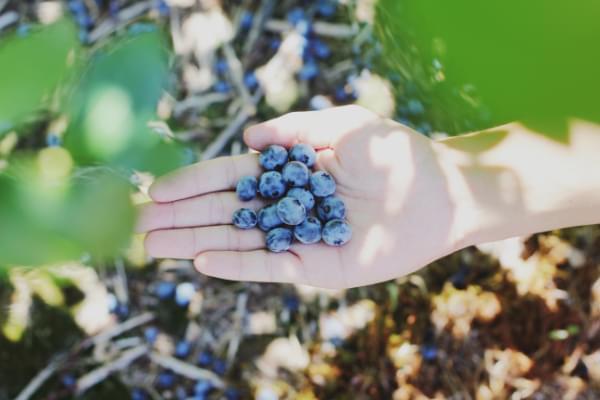
Key Takeaways
- Destructuring assignment in JavaScript allows you to extract individual items from arrays or objects and place them into variables using a shorthand syntax, simplifying code and making it cleaner and easier to read.
- Destructuring can be used in a variety of scenarios including working with APIs responses, functional programming, and in React and other frameworks and libraries. It can also be used with nested objects and arrays, default function parameters, variable value swapping, returning multiple values from a function, for-of iteration, and regular expression handling.
- When using destructuring, it’s important to note that you can’t start a statement with a curly brace as it looks like a code block. To avoid errors, either declare the variables or use parentheses if variables are already declared. Be wary of mixing declared and undeclared variables.
In JavaScript, the destructuring assignment allows you to extract individual items from arrays or objects and place them into variables using a shorthand syntax.
When working with complex data, destructuring can simplify your code by allowing you to easily extract only the values that you need, assign default values, ignore values, and use the rest property to handle the leftover elements or properties.
It is often used in scenarios such as working with APIs responses, functional programming, and in React and other frameworks and libraries.
By simple example, destructuring can make your code look cleaner and easier to read:
// From this:
const author = article.author;
const title = article.title;
const topic = article.topic;
// To this:
const { author, title, topic } = article;
How to use the Destructuring Assignment
Destructuring Arrays
Presume we have an array:
const myArray = ['a', 'b', 'c'];
Destructuring permits a simpler and less error-prone alternative to extracting each element:
const [one, two, three] = myArray;
// one = 'a', two = 'b', three = 'c'
You can ignore certain values by leaving omitting a value name when assigning, e.g.
const [one, , three] = myArray;
// one = 'a', three = 'c'
or use the rest operator (...
) to extract remaining elements:
const [one, ...two] = myArray;
// one = 'a', two = ['b, 'c']
Destructuring Objects
Destructuring also works on objects:
const myObject = {
one: 'a',
two: 'b',
three: 'c'
};
// ES6 destructuring example
const {one, two, three} = myObject;
// one = 'a', two = 'b', three = 'c'
In this example, the variable names one
, two
and three
matched the object property names. We can also assign properties to variables with any name, e.g.
const myObject = {
one: 'a',
two: 'b',
three: 'c'
};
// ES6 destructuring example
const {one: first, two: second, three: third} = myObject;
// first = 'a', second = 'b', third = 'c'
Destructuring Nested Objects
More complex nested objects can also be referenced, e.g.
const meta = {
title: 'Destructuring Assignment',
authors: [
{
firstname: 'Craig',
lastname: 'Buckler'
}
],
publisher: {
name: 'SitePoint',
url: 'https://www.sitepoint.com/'
}
};
const {
title: doc,
authors: [{ firstname: name }],
publisher: { url: web }
} = meta;
/*
doc = 'Destructuring Assignment'
name = 'Craig'
web = 'https://www.sitepoint.com/'
*/
This appears a little complicated but remember that in all destructuring assignments:
- the left-hand side of the assignment is the destructuring target — the pattern which defines the variables being assigned
- the right-hand side of the assignment is the destructuring source — the array or object which holds the data being extracted.
Caveats
There are a number of other caveats. First, you can’t start a statement with a curly brace, because it looks like a code block, e.g.
// THIS FAILS
{ a, b, c } = myObject;
You must either declare the variables, e.g.
// THIS WORKS
const { a, b, c } = myObject;
or use parentheses if variables are already declared, e.g.
// THIS WORKS
({ a, b, c } = myObject);
You should also be wary of mixing declared and undeclared variables, e.g.
// THIS FAILS
let a;
let { a, b, c } = myObject;
// THIS WORKS
let a, b, c;
({ a, b, c } = myObject);
That’s the basics of destructuring. So when would it be useful? I’m glad you asked
Destructuring Use Cases
Easier Declaration
Variables can be declared without explicitly defining each value, e.g.
// ES5
var a = 'one', b = 'two', c = 'three';
// ES6
const [a, b, c] = ['one', 'two', 'three'];
Admittedly, the destructured version is longer. It’s a little easier to read, although that may not be the case with more items.
Variable Value Swapping
Swapping values requires a temporary third variable, but it’s far simpler with destructuring:
var a = 1, b = 2;
// swap
let temp = a;
a = b;
b = temp;
// a = 2, b = 1
// Swap with destructuring assignment
[a, b] = [b, a];
// a = 1, b = 2
You’re not limited to two variables; any number of items can be rearranged, e.g.
// rotate left
[b, c, d, e, a] = [a, b, c, d, e];
Default Function Parameters
Presume we had a function prettyPrint()
to output our meta
object:
var meta = {
title: 'Destructuring Assignment',
authors: [
{
firstname: 'Craig',
lastname: 'Buckler'
}
],
publisher: {
name: 'SitePoint',
url: 'https://www.sitepoint.com/'
}
};
prettyPrint(meta);
Without destructuring, it’s necessary to parse this object to ensure appropriate defaults are available, e.g.
// Default values
function prettyPrint(param) {
param = param || {};
const
pubTitle = param.title || 'No title',
pubName = (param.publisher && param.publisher.name) || 'No publisher';
return pubTitle + ', ' + pubName;
}
Now, we can assign a default value to any parameter, e.g.
// ES6 default value
function prettyPrint(param = {}) {
but we can then use destructuring to extract values and assign defaults where necessary:
// ES6 destructured default value
function prettyPrint(
{
title: pubTitle = 'No title',
publisher: { name: pubName = 'No publisher' }
} = {}
) {
return pubTitle + ', ' + pubName;
}
I’m not convinced this is easier to read, but it’s significantly shorter.
Returning Multiple Values from a Function
Functions can only return one value, but that can be a complex object or multi-dimensional array. Destructuring assignment makes this more practical, e.g.
function f() {
return [1, 2, 3];
}
const [a, b, c] = f();
// a = 1, b = 2, c = 3
For-of Iteration
Consider an array of book information:
const books = [
{
title: 'Full Stack JavaScript',
author: 'Colin Ihrig and Adam Bretz',
url: 'https://www.sitepoint.com/store/full-stack-javascript-development-mean/'
},
{
title: 'JavaScript: Novice to Ninja',
author: 'Darren Jones',
url: 'https://www.sitepoint.com/store/leaern-javascript-novice-to-ninja/'
},
{
title: 'Jump Start CSS',
author: 'Louis Lazaris',
url: 'https://www.sitepoint.com/store/jump-start-css/'
},
];
The ES6 for-of is similar to for-in
, except that it extracts each value rather than the index/key, e.g.
for (const b of books) {
console.log(b.title + ' by ' + b.author + ': ' + b.url);
}
Destructuring assignment provides further enhancements, e.g.
for (const {title, author, url} of books) {
console.log(title + ' by ' + author + ': ' + url);
}
Regular Expression Handling
Regular expressions functions such as match return an array of matched items, which can form the source of a destructuring assignment:
const [a, b, c, d] = 'one two three'.match(/\w+/g);
// a = 'one', b = 'two', c = 'three', d = undefined
Further Reading
- Destructuring Assignment – MDN
- Is there a performance hit for using JavaScript Destructuring – Reddit
- the
for...of
Statement – MDN
Frequently Asked Questions (FAQs) about ES6 Destructuring Assignment
What is the basic syntax of ES6 destructuring assignment?
The basic syntax of ES6 destructuring assignment involves declaring a variable and assigning it a value from an object or array. For instance, if you have an object person
with properties name
and age
, you can extract these values into variables using the following syntax: let {name, age} = person;
. This will create two new variables name
and age
with the values from the corresponding properties in the person
object.
Can I use ES6 destructuring assignment with arrays?
Yes, ES6 destructuring assignment can be used with arrays. The syntax is similar to object destructuring, but uses square brackets instead of curly braces. For example, if you have an array let arr = [1, 2, 3];
, you can extract these values into variables using the following syntax: let [a, b, c] = arr;
. This will create three new variables a
, b
, and c
with the values from the corresponding indices in the array.
How can I use default values with ES6 destructuring assignment?
ES6 destructuring assignment allows you to specify default values for variables that are not found in the object or array. This is done by appending = defaultValue
after the variable name. For example, let {name = 'John', age = 30} = person;
will assign the default values ‘John’ and 30 to name
and age
respectively if these properties do not exist in the person
object.
Can I use ES6 destructuring assignment to swap variables?
Yes, one of the powerful features of ES6 destructuring assignment is the ability to swap variables without the need for a temporary variable. For example, if you have two variables a
and b
, you can swap their values using the following syntax: [a, b] = [b, a];
.
How can I use ES6 destructuring assignment with function parameters?
ES6 destructuring assignment can be used with function parameters to extract values from objects or arrays passed as arguments. For example, if you have a function that takes an object as a parameter, you can extract the object properties into variables using the following syntax: function greet({name, age}) { console.log(
Hello, my name is ${name} and I am ${age} years old.); }
.
Can I use ES6 destructuring assignment with nested objects or arrays?
Yes, ES6 destructuring assignment can be used with nested objects or arrays. The syntax involves specifying the path to the nested property or index. For example, if you have a nested object let person = {name: 'John', address: {city: 'New York', country: 'USA'}};
, you can extract the nested properties into variables using the following syntax: let {name, address: {city, country}} = person;
.
What is the purpose of using ES6 destructuring assignment?
ES6 destructuring assignment is a convenient way of extracting multiple properties from objects or elements from arrays into distinct variables. This can make your code cleaner and more readable, especially when dealing with complex data structures.
Can I use ES6 destructuring assignment with rest parameters?
Yes, ES6 destructuring assignment can be used with rest parameters to collect the remaining elements of an array into a new array. The syntax involves appending ...
before the variable name. For example, let [a, b, ...rest] = [1, 2, 3, 4, 5];
will assign the first two elements to a
and b
, and the remaining elements to the rest
array.
Can I use ES6 destructuring assignment to extract properties from objects into new variables with different names?
Yes, ES6 destructuring assignment allows you to extract properties from objects into new variables with different names. This is done by specifying the new variable name after a colon. For example, let {name: firstName, age: years} = person;
will create two new variables firstName
and years
with the values from the name
and age
properties respectively.
What happens if I try to destructure a property or element that does not exist?
If you try to destructure a property from an object or an element from an array that does not exist, the variable will be assigned the value undefined
. However, you can specify a default value to be used in such cases, as explained in Question 3.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.