jQuery function to Set any DOM Element to Top View (bring to front) using CSS Z-Index property.
jQuery.fn.mb_bringToFront= function(zIndexContext){
var zi=1;
var els= zIndexContext && zIndexContext!="auto" ? $(zIndexContext): $("*");
els.not(".alwaysOnTop").each(function() {
if($(this).css("position")!="static"){
var cur = parseInt($(this).css('zIndex'));
zi = cur > zi ? parseInt($(this).css('zIndex')) : zi;
}
});
$(this).not(".alwaysOnTop").css('zIndex',zi+=1);
return zi;
};
Frequently Asked Questions about jQuery and Z-Index
How can I set the z-index of a DOM element using jQuery?
To set the z-index of a DOM element using jQuery, you can use the .css() method. This method allows you to get or set the style properties of selected elements. Here’s an example of how you can use it to set the z-index:$("#element").css("z-index", "999");
In this example, “#element” is the id of the DOM element you want to change, and “999” is the new z-index value.
Why isn’t my z-index change taking effect?
There could be several reasons why your z-index change isn’t taking effect. One common reason is that the element you’re trying to change isn’t positioned. The z-index property only works on positioned elements (i.e., elements with position: relative, position: absolute, or position: fixed). If your element isn’t positioned, you can set its position using the .css() method, like this:$("#element").css("position", "relative");
How can I get the current z-index of a DOM element?
You can get the current z-index of a DOM element using the .css() method, like this:var zIndex = $("#element").css("z-index");
In this example, zIndex will hold the current z-index of the element with id “element”.
Can I set the z-index of multiple elements at once?
Yes, you can set the z-index of multiple elements at once using the .css() method. Here’s an example:$(".elements").css("z-index", "999");
In this example, all elements with the class “elements” will have their z-index set to “999”.
How can I dynamically change the z-index of an element?
You can dynamically change the z-index of an element using jQuery’s .css() method in combination with event handlers. For example, you could increase the z-index of an element when it’s clicked, like this:$("#element").click(function() {
var zIndex = parseInt($(this).css("z-index"));
$(this).css("z-index", zIndex + 1);
});
In this example, each time the element with id “element” is clicked, its z-index will increase by 1.
What is the maximum value I can set for z-index?
The maximum value you can set for z-index depends on the browser. Most browsers support z-index values up to 2147483647.
Can I use negative values for z-index?
Yes, you can use negative values for z-index. A negative z-index means that the element will be displayed behind other elements that have a z-index of 0 or greater.
Does z-index work with all HTML elements?
The z-index property works with all HTML elements, but it only affects elements that are positioned (i.e., elements with position: relative, position: absolute, or position: fixed).
Can I use z-index to create a stacking context?
Yes, you can use z-index to create a stacking context. A stacking context is a three-dimensional conceptualization of HTML elements along an imaginary z-axis relative to the user, who is assumed to be facing the viewport or the webpage.
How does z-index work with parent and child elements?
If a parent element has a higher z-index than its child, the child will still appear on top of the parent. This is because child elements are stacked in front of their parent by default. However, if the parent and child elements are in different stacking contexts, the one with the higher z-index will appear on top.
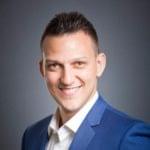
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.