Simple jQuery code snippet to loop select box options (drop down boxes) in a form to get the values and text from each option. Useful for manipulating values in form select boxes.
$('#select > option').each(function() {
alert($(this).text() + ' ' + $(this).val());
});
$('#select > option:selected').each(function() {
alert($(this).text() + ' ' + $(this).val());
});
This function will return an array of text/value pairs for the selects matching the given class.
function getSelects(klass) {
var selected = [];
$('select.' + klass).children('option:selected').each( function() {
var $this = $(this);
selected.push( { text: $this.text(), value: $this.val() );
});
return selected;
}
Frequently Asked Questions (FAQs) about jQuery Loop Select Options
How can I use jQuery to loop through select options in a dropdown list?
jQuery provides a simple and efficient way to loop through select options in a dropdown list. You can use the .each()
function, which is a built-in jQuery method that iterates over the DOM elements that are part of the jQuery object. Here’s a basic example:$('select option').each(function() {
var optionValue = $(this).val();
var optionText = $(this).text();
// Perform your operations here
});
In this code, $('select option')
selects all the options in the dropdown list. The .each()
function then loops through each option, and for each option, it gets the value and the text.
How can I select a specific option in a dropdown list using jQuery?
jQuery provides several ways to select a specific option in a dropdown list. One common method is to use the :selected
selector. This selector selects the option that is currently selected in the dropdown list. Here’s an example:var selectedOption = $('select option:selected').val();
In this code, $('select option:selected')
selects the currently selected option in the dropdown list, and .val()
gets its value.
How can I change the selected option in a dropdown list using jQuery?
You can change the selected option in a dropdown list using the .val()
method in jQuery. This method sets or returns the value of the selected elements. Here’s an example:$('select').val('Option2');
In this code, $('select').val('Option2')
changes the selected option to the option with the value ‘Option2’.
How can I add an option to a dropdown list using jQuery?
You can add an option to a dropdown list using the .append()
method in jQuery. This method inserts specified content at the end of the selected elements. Here’s an example:$('select').append('<option value="newOption">New Option</option>');
In this code, $('select').append('<option value="newOption">New Option</option>')
adds a new option with the value ‘newOption’ and the text ‘New Option’ to the dropdown list.
How can I remove an option from a dropdown list using jQuery?
You can remove an option from a dropdown list using the .remove()
method in jQuery. This method removes the selected elements, including all text and child nodes. Here’s an example:$('select option[value="Option2"]').remove();
In this code, $('select option[value="Option2"]').remove()
removes the option with the value ‘Option2’ from the dropdown list.
How can I disable an option in a dropdown list using jQuery?
You can disable an option in a dropdown list using the .attr()
method in jQuery. This method sets or returns attributes and values of the selected elements. Here’s an example:$('select option[value="Option2"]').attr('disabled', 'disabled');
In this code, $('select option[value="Option2"]').attr('disabled', 'disabled')
disables the option with the value ‘Option2’ in the dropdown list.
How can I enable a disabled option in a dropdown list using jQuery?
You can enable a disabled option in a dropdown list using the .removeAttr()
method in jQuery. This method removes one or more attributes from the selected elements. Here’s an example:$('select option[value="Option2"]').removeAttr('disabled');
In this code, $('select option[value="Option2"]').removeAttr('disabled')
enables the option with the value ‘Option2’ in the dropdown list.
How can I get the number of options in a dropdown list using jQuery?
You can get the number of options in a dropdown list using the .length
property in jQuery. This property returns the number of elements in the jQuery object. Here’s an example:var numberOfOptions = $('select option').length;
In this code, $('select option').length
gets the number of options in the dropdown list.
How can I clear all options in a dropdown list using jQuery?
You can clear all options in a dropdown list using the .empty()
method in jQuery. This method removes all child nodes and content from the selected elements. Here’s an example:$('select').empty();
In this code, $('select').empty()
removes all options from the dropdown list.
How can I check if a dropdown list is empty using jQuery?
You can check if a dropdown list is empty using the .children()
method and the .length
property in jQuery. The .children()
method gets the children of each element in the set of matched elements, optionally filtered by a selector. Here’s an example:if ($('select').children().length == 0) {
// The dropdown list is empty
}
In this code, $('select').children().length == 0
checks if the dropdown list is empty. If it is, it executes the code inside the if statement.
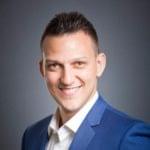
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.