jQuery code snippet find the highest z-index of absolute divs. Useful for making a new element appear absolutely at the top of all html elements.
//include jQuery.js -- visit: http://jquery.com/
$(function(){
var maxZ = Math.max.apply(null,$.map($('body > *'), function(e,n){
if($(e).css('position')=='absolute')
return parseInt($(e).css('z-index'))||1 ;
})
);
alert(maxZ);
});
Source
Frequently Asked Questions (FAQs) about jQuery and Z-Index
How can I find the highest Z-Index on a page using jQuery?
To find the highest Z-Index on a page using jQuery, you can use the .css()
method to get the Z-Index of each element and then compare them to find the highest one. Here is a simple code snippet that does this:var highestZIndex = 0;
$("*").each(function() {
var currentZIndex = parseInt($(this).css("z-index"), 10);
if(currentZIndex > highestZIndex) {
highestZIndex = currentZIndex;
}
});
console.log(highestZIndex);
This code will log the highest Z-Index value to the console.
What is the purpose of Z-Index in jQuery?
The Z-Index in jQuery is used to specify the stack order of elements. An element with a higher Z-Index is always in front of an element with a lower Z-Index. This is particularly useful when you have overlapping elements and you want to control which element should be on top.
How can I set the Z-Index of an element using jQuery?
You can set the Z-Index of an element using the .css()
method in jQuery. Here is an example:$("#myElement").css("z-index", 9999);
This will set the Z-Index of the element with the ID “myElement” to 9999.
Can I use negative values for Z-Index in jQuery?
Yes, you can use negative values for Z-Index in jQuery. A negative Z-Index means that the element will be placed behind other elements that have a Z-Index of 0 or greater.
How does Z-Index work with positioned elements in jQuery?
The Z-Index property only works on positioned elements (position:absolute, position:relative, or position:fixed). If the position property is static (the default), the Z-Index property has no effect.
What happens if two elements have the same Z-Index?
If two elements have the same Z-Index, the element that appears later in the HTML will be displayed on top.
How can I find the Z-Index of a specific element using jQuery?
You can find the Z-Index of a specific element using the .css()
method in jQuery. Here is an example:var zIndex = $("#myElement").css("z-index");
console.log(zIndex);
This will log the Z-Index of the element with the ID “myElement” to the console.
Can I use the Z-Index property with non-positioned elements?
No, the Z-Index property only works with positioned elements. If an element is not positioned, the Z-Index property will have no effect.
How can I increment the Z-Index of an element using jQuery?
You can increment the Z-Index of an element using the .css()
method in jQuery. Here is an example:var currentZIndex = parseInt($("#myElement").css("z-index"), 10);
$("#myElement").css("z-index", currentZIndex + 1);
This will increment the Z-Index of the element with the ID “myElement” by 1.
Can I use the Z-Index property with the animate()
method in jQuery?
No, the Z-Index property cannot be animated using the animate()
method in jQuery. The animate()
method can only be used with properties that have numeric values, and the Z-Index property does not have a numeric value.
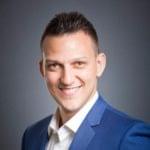
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.