jQuery code snippet to autoScroll to a div or any page element with an id. Just change the jQuery selector “mydiv” with whatever element id you wish.
function scroll_to(div){
$('html, body').animate({
scrollTop: $("mydiv").offset().top
},1000);
}
Frequently Asked Questions (FAQs) about jQuery Autoscroll
How can I implement jQuery autoscroll to a specific element on my webpage?
Implementing jQuery autoscroll to a specific element on your webpage involves a few steps. First, you need to include the jQuery library in your HTML file. You can do this by adding the following script tag in your HTML file: <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
.
Next, you need to identify the specific element you want to scroll to. This can be done by using the element’s ID or class. For instance, if you have a div with an ID of “target”, you can select it using $('#target')
.
Finally, you can use the animate
function in jQuery to smoothly scroll to the selected element. Here’s a sample code snippet:$('html, body').animate({
scrollTop: $('#target').offset().top
}, 2000);
In this code, 2000
is the duration of the scroll animation in milliseconds. You can adjust this value according to your needs.
Can I use jQuery autoscroll for elements that are dynamically loaded?
Yes, you can use jQuery autoscroll for elements that are dynamically loaded. However, you need to ensure that the autoscroll code is executed after the dynamic content has been loaded. This can be done by placing the autoscroll code inside a callback function that is executed after the dynamic content is loaded.
For instance, if you’re using the $.ajax
function to load dynamic content, you can place the autoscroll code inside the success
callback function. Here’s a sample code snippet:$.ajax({
url: 'your-url',
success: function(data) {
// Load dynamic content
$('#container').html(data);
// Autoscroll to specific element
$('html, body').animate({
scrollTop: $('#target').offset().top
}, 2000);
}
});
In this code, #container
is the ID of the element where the dynamic content is loaded, and #target
is the ID of the element you want to scroll to.
How can I stop the jQuery autoscroll animation?
You can stop the jQuery autoscroll animation by using the stop
function. This function stops the currently running animation on the selected elements. Here’s a sample code snippet:$('html, body').stop();
This code will immediately stop the autoscroll animation. If you want to stop the animation and immediately scroll to the target element, you can use the scrollTop
function after the stop
function:$('html, body').stop().scrollTop($('#target').offset().top);
In this code, #target
is the ID of the element you want to scroll to.
Can I use jQuery autoscroll on mobile devices?
Yes, you can use jQuery autoscroll on mobile devices. However, the scrolling behavior might be different depending on the mobile browser. Some mobile browsers might not support smooth scrolling, or they might have a different scrolling speed.
To ensure a consistent scrolling behavior across different mobile browsers, you can use a jQuery plugin like Smooth Scroll. This plugin provides a smooth scrolling experience on all browsers and devices.
How can I adjust the speed of the jQuery autoscroll animation?
You can adjust the speed of the jQuery autoscroll animation by changing the duration parameter in the animate
function. The duration is specified in milliseconds. For instance, if you want the scroll animation to last for 3 seconds, you can set the duration to 3000:$('html, body').animate({
scrollTop: $('#target').offset().top
}, 3000);
In this code, #target
is the ID of the element you want to scroll to, and 3000
is the duration of the scroll animation in milliseconds.
Can I use jQuery autoscroll with horizontal scrolling?
Yes, you can use jQuery autoscroll with horizontal scrolling. Instead of using the scrollTop
function, you can use the scrollLeft
function. Here’s a sample code snippet:$('html, body').animate({
scrollLeft: $('#target').offset().left
}, 2000);
In this code, #target
is the ID of the element you want to scroll to, and 2000
is the duration of the scroll animation in milliseconds.
How can I use jQuery autoscroll with a fixed header or navbar?
If you have a fixed header or navbar, you might notice that the autoscroll animation scrolls to the target element, but the element is hidden behind the header or navbar. To fix this, you can subtract the height of the header or navbar from the scroll position. Here’s a sample code snippet:$('html, body').animate({
scrollTop: $('#target').offset().top - $('#header').outerHeight()
}, 2000);
In this code, #target
is the ID of the element you want to scroll to, #header
is the ID of the header or navbar, and 2000
is the duration of the scroll animation in milliseconds.
Can I use jQuery autoscroll with multiple target elements?
Yes, you can use jQuery autoscroll with multiple target elements. You can do this by using a loop to iterate over the target elements and apply the autoscroll animation to each one. Here’s a sample code snippet:$('.target').each(function() {
$('html, body').animate({
scrollTop: $(this).offset().top
}, 2000);
});
In this code, .target
is the class of the target elements, and 2000
is the duration of the scroll animation in milliseconds.
How can I trigger the jQuery autoscroll animation on a button click?
You can trigger the jQuery autoscroll animation on a button click by using the click
event. Here’s a sample code snippet:$('#button').click(function() {
$('html, body').animate({
scrollTop: $('#target').offset().top
}, 2000);
});
In this code, #button
is the ID of the button, #target
is the ID of the element you want to scroll to, and 2000
is the duration of the scroll animation in milliseconds.
Can I use jQuery autoscroll with a scrollspy?
Yes, you can use jQuery autoscroll with a scrollspy. A scrollspy is a navigation mechanism that automatically highlights the navigation links based on the scroll position.
To use jQuery autoscroll with a scrollspy, you need to modify the click event of the navigation links to include the autoscroll animation. Here’s a sample code snippet:$('#navbar a').click(function(event) {
event.preventDefault();
var target = $(this).attr('href');
$('html, body').animate({
scrollTop: $(target).offset().top
}, 2000);
});
In this code, #navbar a
is the selector for the navigation links, and 2000
is the duration of the scroll animation in milliseconds.
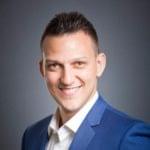
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.