A Guide to Optimizing JavaScript Files
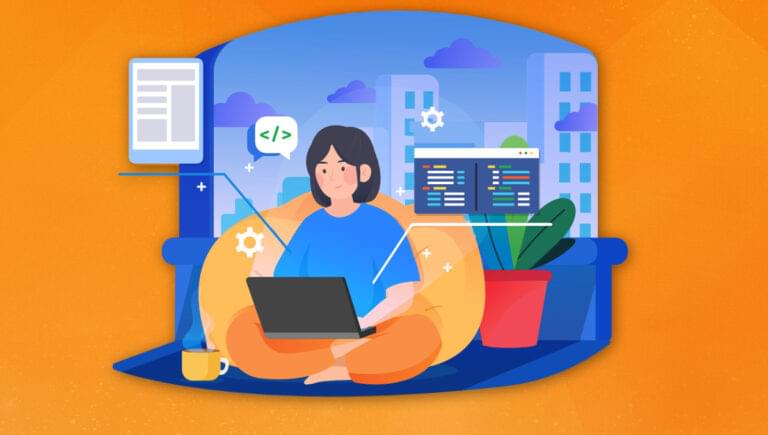
This article will explore practical JavaScript file optimization techniques, how to deal with performance issues related to JavaScript files, and tools to help the optimization process. You’ll gain the knowledge to boost your web application speed and provide a seamless experience to your users.
JavaScript files are vital aspects of the web application process, but website speed and user experience are critical to a website’s success. So it’s crucial to optimize JavaScript files to ensure seamless performance. Optimizing JavaScript files resolves the issue of render blocking, page load time, large file size, and so on.
Key Takeaways
Understanding JavaScript Optimization
JavaScript optimization is the process of improving the performance of JavaScript. To understand the benefits of JavaScript optimization, we first have to understand the issues associated with JavaScript. Some include:
- Script execution. JavaScript files containing blocking code delay page rendering. Script execution prevents other content from loading, resulting in a poor user experience.
- Large file size. Large JavaScript files take longer to download, impacting page load times.
- Code complexity and inefficiencies. Poorly optimized JavaScript code — such as excessive loops, redundant calculations, or inefficient algorithms — leads to performance blocks.
The benefits of optimizing JavaScript files are numerous. JavaScript optimization helps improve the responsiveness and interactivity of web applications, delivering a more satisfying user experience and better performance. It includes faster form submissions, dynamic content updates, and smooth animations.
By helping to reduce the size of JavaScript files and optimize their delivery, page load times are faster. Slow-loading pages result in higher bounce rates and negatively impact user experience, while reduced friction increases the likelihood of conversions.
Search engines consider page load times as a ranking factor. Optimizing JavaScript files improves website performance, thus improving search engine rankings.
Methods for JavaScript Optimization
Let’s look at practical steps to optimize our JavaScript files.
Minification
Minifying JavaScript files involves removing unnecessary characters, whitespace, and comments to reduce the file size. It helps to improve load times by reducing the amount of data that needs transferring from the server to the client browser.
Compression
Compressing JavaScript files — using techniques like gzip compression — reduces file sizes. The compressed files are sent from the server to the browser and decompressed for execution, resulting in faster downloads and improved website performance.
For more information on JavaScript compression, check out these resources:
- JSCompress
- Easy JavaScript Compression with the Closure Compiler REST API
- Compress CSS and JavaScript Using PNGs and Canvas
Asynchronous and deferred loading
JavaScript files load synchronously by default, meaning they block the rendering of the web page until the script fully loads and executes. Asynchronous and deferred loading techniques allow JavaScript files to load independently of the page rendering process, minimizing the impact on load times. Asynchronous loading ensures that the script is loaded and executed as soon as it’s available, while deferred loading delays the execution until the HTML parsing is complete.
For more information on asynchronous and deferred loading, check out these resources:
- Load Non-blocking JavaScript with HTML5 Async and Defer
- Five Ways to Lazy Load Images for Better Website Performance
- JavaScript Goes Asynchronous (and It’s Awesome)
Improving Load Performance
We’ll now consider some ways to improve page load performance.
Conditional and lazy loading
Lazy loading is a technique where JavaScript files load only when needed, like when a specific action or event occurs on the web page. It reduces the initial page load time by deferring the loading of non-critical scripts until required, enhancing the total user experience.
Conditional loading allows you to load JavaScript files selectively based on specific conditions. For example, you can load different scripts based on the user device type, browser capabilities, or user interactions. Loading only the necessary scripts reduces the payload and improves performance.
Dependency management and script concatenation
Managing dependencies between JavaScript files is crucial for efficient loading. Script concatenation involves combining multiple JavaScript files to a single file, reducing the number of HTTP requests needed to load the scripts. This merge minimizes network latency and increases load times.
Tree shaking
Tree shaking is commonly used with module bundlers like Webpack. It eliminates unused code from JavaScript modules during the build process, reducing the file size and enhancing performance. Tree shaking helps optimize the delivery of only the necessary code to the web browser.
Caching and content delivery networks (CDNs)
Leveraging browser caching and utilizing CDNs can improve JavaScript file loading times. Caching allows the browser to store and reuse previously loaded JavaScript files, reducing the need for repeated downloads. CDNs store JavaScript files in multiple locations worldwide, enabling faster delivery to users by serving the files from a server closer to their geographical location.
Code organization and modularization
For better functionality, split your JavaScript code into modular components or modules. Use bundlers to combine and optimize the code into a single bundle. Apply a modular design pattern (ES modules) to ensure better code organization and maintainability.
Performance monitoring and testing
Engage performance monitoring tools (such as Lighthouse and WebPageTest) to analyze JavaScript performance and identify areas for improvement. Regularly test your website load times and responsiveness under various device types and network conditions.
Regular updates and optimization reviews
Stay updated with the latest best practices and advancements in JavaScript optimization procedures. Review and optimize your JavaScript codebase to remove redundancies, improve performance, and ensure compatibility with new browser features and standards.
Leveraging (Plain) JavaScript for Optimization
Leveraging plain JavaScript can lead to efficient optimization without relying on external tools or libraries like React, Vue, and Angular. Here are some practical ways to optimize your JavaScript code.
Efficient loops and iterations
Avoid unnecessary work within loops and use methods like map
, filter
, and reduce
for array manipulations.
Suppose you have an array of numbers and want to square each number:
// Original loop-based approach:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = [];
for (let i = 0; i < numbers.length; i++) {
squaredNumbers.push(numbers[i] * numbers[i]);
}
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Now, let’s optimize this loop using the map
method:
// Optimized approach using map:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(number => number * number);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
In this example, the map
method creates a new array called squaredNumbers
. The map
method iterates over each element in the numbers array, applies the provided callback function (in this case, squaring the number), and returns a new array with the transformed values.
The optimized approach with map
is more concise and easier to read and maintain. It benefits from performance optimizations using built-in array methods like maps.
Debouncing and throttling
When handling events that trigger frequent JavaScript executions (such as window resizing or scrolling), implement debouncing or throttling to control the rate of function calls and reduce unnecessary processing.
Here’s a debouncing example:
function debounce(func, delay) {
let timeout;
return (...args) => {
clearTimeout(timeout);
timeout = setTimeout(() => func(...args), delay);
};
}
const handleResize = () => {
// Perform resizing-related tasks
};
window.addEventListener('resize', debounce(handleResize, 300));
Use efficient data structures
Choose appropriate data structures for your application. For example, use Map
or Set
when fast data retrieval or uniqueness is required.
Here’s an example of using Set
:
const uniqueValues = new Set();
uniqueValues.add(1);
uniqueValues.add(2);
uniqueValues.add(1); // Won't be added again
console.log([...uniqueValues]); // [1, 2]
Use textContent instead of innerHTML
When updating the content of an element, use the textContent
property over innerHTML to avoid potential security risks and improve performance.
Here’s an example of using textContent
:
// Avoid using innerHTML:
const element = document.getElementById('myElement');
element.innerHTML = '<strong>Updated content</strong>';
// With textContent:
const element = document.getElementById('myElement');
element.textContent = 'Updated content';
Efficient error handling
Proper error handling is crucial for maintaining application stability. However, avoid overusing try–catch blocks, as they can impact performance. Use them only when necessary, with potentially failing code.
Let’s look at an example of efficient error handling. Suppose you have a function that parses JSON data. You want to handle errors that might occur during the JSON parsing process:
function parseJson(jsonString) {
try {
const parsedData = JSON.parse(jsonString);
return parsedData;
} catch (error) {
console.error('Error parsing JSON:', error.message);
return null;
}
}
const validJson = '{"name": "John", "age": 30}';
const invalidJson = 'invalid-json';
const validResult = parseJson(validJson);
console.log(validResult); // Output: { name: 'John', age: 30 }
const invalidResult = parseJson(invalidJson);
console.log(invalidResult); // Output: null
In this example, the parseJson()
attempts to parse a JSON string using JSON.parse()
. If the parsing is successful, it returns the parsed data. However, if an error occurs (for example, due to invalid JSON syntax), the catch block catches the error and logs an appropriate error message. The function then returns null
.
By using the try–catch block in this way, you handle potential errors without negatively impacting performance. This approach ensures you properly catch and manage errors while only applying the error handling logic when necessary.
Efficient event handling
Use event delegation to minimize the number of event listeners attached to individual elements. This is useful when dealing with multiple elements of the same type.
Here’s an example of event delegation:
// Instead of attaching individual event listeners:
const buttons = document.querySelectorAll('.button');
buttons.forEach(button => {
button.addEventListener('click', handleClick);
});
// Use event delegation on a parent element:
document.addEventListener('click', event => {
if (event.target.classList.contains('button')) {
handleClick(event);
}
});
Reduce/avoid global variables
Minimize the use of global variables to prevent namespace pollution and potential conflicts. Instead, use module patterns or closures to encapsulate functionality.
Here’s an example of using a closure:
const counter = (function () {
let count = 0;
return {
increment: function () {
count++;
},
getCount: function () {
return count;
},
};
})();
counter.increment();
console.log(counter.getCount()); // Output: 1
DOM fragment for batch updates
When making multiple changes to the DOM, create a DocumentFragment
to batch those changes before appending to the actual DOM. It reduces reflows and enhances performance.
Here’s an example of using a DocumentFragment
:
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const element = document.createElement('div');
element.textContent = `Item ${i}`;
fragment.appendChild(element);
}
document.getElementById('container').appendChild(fragment);
Efficient string concatenation
Use template literals for efficient string concatenation, as they provide better readability and performance, unlike traditional string concatenation methods.
Here’s an example of using template literals:
const name = 'John';
const age = 30;
const message = `My name is ${name} and I am ${age} years old.`;
Caching expensive calculations
Cache the results of expensive calculations or function calls to avoid redundant processing.
Here’s an example of caching calculations:
const cache = {};
function expensiveCalculation(input) {
if (cache[input]) {
return cache[input];
}
const result = performExpensiveCalculation(input);
cache[input] = result;
return result;
}
function performExpensiveCalculation(input) {
//an expensive calculation (factorial)
let result = 1;
for (let i = 1; i <= input; i++) {
result *= i;
}
return result;
}
// Test the expensive calculation with caching
console.log(expensiveCalculation(5)); // Output: 120 (5 factorial)
console.log(expensiveCalculation(7)); // Output: 5040 (7 factorial)
console.log(expensiveCalculation(5)); // Output: 120 (Cached result)
In this example, the expensiveCalculation()
checks whether the result for a given input is already present in the cache object. If found, it returns directly. Otherwise, the expensive calculation loads using the performExpensiveCalculation()
, and the result gets stored in the cache before being returned.
Using Tools for JavaScript File Optimization
These tools offer various features and functionalities to streamline the optimization process, and improve website performance.
Webpack
Webpack is a powerful module bundler that helps with dependency management and offers optimization features. With Webpack, you can bundle and concatenate JavaScript files, optimize file size, and apply advanced optimizations like tree shaking and code splitting. It also supports integrating other optimization tools and plugins into the build process.
CodeSee
CodeSee is a very useful tool for JavaScript file optimization. It provides insights into codebases, facilitates code exploration, and aids the identification of optimization opportunities. You can do things like visualize code dependencies, analyze code complexity, navigate your codebase, carry out time travel debugging, perform collaborative code review, maintain code, and generate documentation for your code.
UglifyJS
UglifyJS is a JavaScript minification tool. It removes unnecessary characters, renames variables, and performs other optimizations to reduce file size. It supports ECMAScript 5 and advanced versions, making it compatible with modern JavaScript code.
Babel
Babel is a versatile JavaScript compiler that allows developers to write code using the latest JavaScript features and syntax while ensuring compatibility with older browsers. Babel transpiles modern JavaScript code into backward-compatible versions, optimizing the code for broader browser support.
Grunt
Grunt is a task runner that automates repetitive tasks in JavaScript projects, including JavaScript optimization. It provides many plugins and configurations for minifying, concatenating, and compressing JavaScript files. Grunt simplifies the optimization workflow and can be customized to suit specific project requirements.
Gulp
Gulp is another accepted task runner that streamlines the build process, including JavaScript optimization. It uses a code-over-configuration approach and offers a vast ecosystem of plugins. Gulp allows developers to define custom tasks for minification, concatenation, and other optimization techniques.
Rollup
Rollup is a module bundler designed for modern JavaScript projects. It focuses on creating optimized bundles by leveraging tree shaking and code splitting. Rollup helps eliminate dead code and produce smaller, more efficient JavaScript files.
The Closure Compiler
The Closure Compiler is a JavaScript optimization tool developed by Google. It analyzes and minifies JavaScript code, performs advanced optimizations, and provides static analysis to optimize runtime performance. The Closure Compiler is handy for large-scale projects and applications.
WP Rocket
WP Rocket is a popular WordPress caching plugin that offers built-in optimization features for JavaScript files. It can minify and concatenate JavaScript files, integrate with CDNs, and provide advanced caching options to improve website performance.
ESLint
ESLint, while not an optimization tool, is a powerful linter for JavaScript that helps enforce code quality and identify potential performance issues. It can detect and flag problematic patterns or inefficient code practices that could impact the performance of JavaScript files.
Lighthouse
Lighthouse is an open-source tool from Google that audits web pages for performance, accessibility, and best practices. It provides suggestions and recommendations for optimizing JavaScript code, including reducing file sizes, eliminating render-blocking scripts, and leveraging caching.
Wrapping Up
JavaScript file optimization is necessary for improving performance, providing a more responsive and interactive user experience, improving search engine rankings, reducing page load times, and increasing conversion rates of web applications.
Addressing issues like script execution delays, large file sizes, render-blocking scripts, and code complexity helps the JavaScript optimization process. You may use various techniques for JavaScript optimization, including minification, compression, asynchronous/deferred loading, conditional/lazy loading, dependency management, script concatenation, tree shaking, caching, and CDNs.
Using plain JavaScript techniques empowers you to optimize your codebase without relying on external libraries. You achieve better performance and a smoother user experience in your web applications.
Several tools such as Webpack, CodeSee, UglifyJS, Babel, Grunt, Gulp, Rollup, Closure Compiler, WP Rocket, ESLint, and Lighthouse effectively streamline the JavaScript optimization process, automate tasks, and improve website performance.
To ensure continuous improvement, stay updated with the latest best practices, regularly review and optimize JavaScript codebases, and utilize performance monitoring tools to identify areas for improvement. By prioritizing JavaScript file optimization, you deliver faster, more efficient web applications providing a seamless user experience.
Frequently Asked Questions (FAQs) about Optimizing JavaScript Files
What are the benefits of optimizing JavaScript files?
Optimizing JavaScript files can significantly improve the performance of your website or application. It reduces the file size, making it quicker to download and load. This can lead to a better user experience, as pages load faster and are more responsive. Additionally, optimized JavaScript files consume less bandwidth, which can be beneficial for users with limited internet connections. Lastly, it can also improve your website’s SEO ranking, as search engines favor sites that load quickly.
How can I minify my JavaScript files?
Minification is a process that removes unnecessary characters (like spaces, line breaks, comments) from the code without changing its functionality. You can use various online tools and npm packages like UglifyJS, Terser, and others for this purpose. These tools parse your JavaScript files and produce a minified version that you can use in your production environment.
What is the difference between JavaScript minification and obfuscation?
While both minification and obfuscation aim to reduce the size of the JavaScript file, they serve different purposes. Minification removes unnecessary characters to reduce file size and improve load times. On the other hand, obfuscation alters the code to make it harder to understand and reverse-engineer, providing a layer of security for the code.
How does gzip compression work in optimizing JavaScript files?
Gzip is a file format and a software application used for file compression and decompression. When applied to JavaScript files, it can significantly reduce the file size, thus improving load times. When a user visits your website, the server sends the gzipped file, which is then decompressed by the user’s browser.
What is the role of Content Delivery Networks (CDNs) in JavaScript file optimization?
CDNs can significantly improve the delivery of JavaScript files to your users. They work by distributing your files across a network of servers around the world. When a user requests your site, the CDN delivers the JavaScript files from the server closest to the user, reducing the time it takes for the data to travel, thus improving load times.
How can I use async and defer attributes to optimize JavaScript loading?
The async and defer attributes allow you to control how JavaScript files are loaded in relation to the rest of your webpage. The async attribute loads the script while the rest of the page continues to parse. The defer attribute, on the other hand, tells the browser to hold off on executing the script until the HTML parsing is complete. Using these attributes can help prevent JavaScript files from blocking the rendering of your webpage, thus improving load times.
What is tree shaking in JavaScript?
Tree shaking is a term commonly used in the JavaScript context for dead-code elimination. It involves removing unused code from the final bundle during the build process, thus reducing the size of the resulting JavaScript file and improving load times.
How can I optimize JavaScript execution?
Optimizing JavaScript execution can be achieved by following best practices such as avoiding global variables, reducing DOM manipulation, using requestAnimationFrame for animations, debouncing and throttling events, and using Web Workers for complex calculations.
What is the impact of JavaScript optimization on SEO?
JavaScript optimization can significantly improve your website’s SEO. Search engines favor websites that load quickly and provide a smooth user experience. By reducing the size of your JavaScript files and optimizing their delivery and execution, you can improve your site’s load times, which can lead to higher rankings in search engine results.
How can I measure the performance of my JavaScript code?
You can use various tools to measure the performance of your JavaScript code. The Performance API in modern browsers provides data on various aspects of your site’s performance. Tools like Google Lighthouse and WebPageTest can provide comprehensive reports on your site’s performance, including JavaScript execution. Additionally, the Chrome DevTools Performance panel allows you to record and analyze runtime performance, helping you identify areas of your code that could be optimized.
Blessing is a proficient technical writer with 3+ years of expertise in software documentation and user guides, actively mentoring aspiring writers and contributing to open-source projects to foster growth and understanding in the tech sector.