ES6 in Action: New String Methods — String.prototype.*
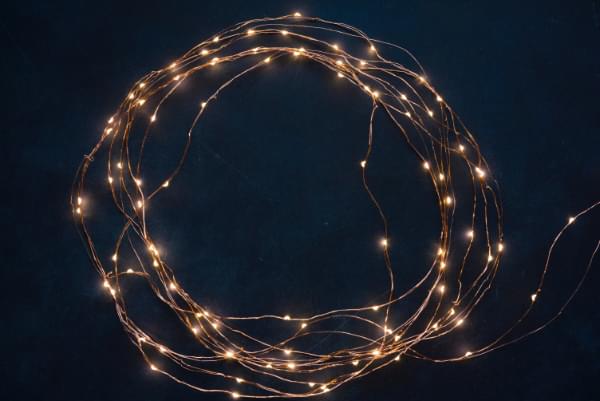
In my previous article on ES6 array methods, I introduced the new methods available in ECMAScript 6 that work with the Array
type. In this tutorial, you’ll learn about new ES6 methods that work with strings: String.prototype.*
We’ll develop several examples, and mention the polyfills available for them. Remember that if you want to polyfill them all using a single library, you can employ es6-shim by Paul Miller.
Key Takeaways
- ES6 introduces several new methods for string manipulation, including String.prototype.startsWith(), String.prototype.endsWith(), String.prototype.includes(), String.prototype.repeat(), and String.raw(). These methods provide easier and more efficient ways to check if a string starts or ends with a given substring, if a string contains a certain substring, to repeat a string a specified number of times, and to process template strings.
- The String.prototype.startsWith() and String.prototype.endsWith() methods in ES6 allow you to verify if a string starts or ends with a given substring. They both accept a substring and a position as parameters, and return true or false depending on whether the string starts or ends with the provided substring.
- The String.prototype.includes() method in ES6 returns true if a string is contained within another string, no matter the position. It accepts the same parameters as the startsWith() and endsWith() methods.
- The String.prototype.repeat() method in ES6 returns a new string containing the original string repeated a specified number of times. The String.raw() function is a tag function of template strings that compiles a string and replaces placeholders with provided values.
String.prototype.startsWith()
One of the most-used functions in every modern programming language is the one to verify if a string starts with a given substring. Before ES6, JavaScript had no such function, meaning you had to write it yourself. The following code shows how developers usually polyfilled it:
if (typeof String.prototype.startsWith !== 'function') {
String.prototype.startsWith = function (str){
return this.indexOf(str) === 0;
};
}
Or, alternatively:
if (typeof String.prototype.startsWith !== 'function') {
String.prototype.startsWith = function (str){
return this.substring(0, str.length) === str;
};
}
These snippets are still valid, but they don’t reproduce exactly what the newly available String.prototype.startsWith()
method does. The new method has the following syntax:
String.prototype.startsWith(searchString[, position]);
You can see that, in addition to a substring, it accepts a second argument. The searchString
parameter specifies the substring you want to verify is the start of the string. position
indicates the position at which to start the search. The default value of position
is 0. The methods returns true
if the string starts with the provided substring, and false
otherwise. Remember that the method is case sensitive, so “Hello” is different from “hello”.
An example use of this method is shown below:
const str = 'hello!';
let result = str.startsWith('he');
// prints "true"
console.log(result);
// verify starting from the third character
result = str.startsWith('ll', 2);
// prints "true"
console.log(result);
A live demo of the previous code is shown below and also available at JSBin.
The method is supported in Node and all modern browsers, with the exception of Internet Explorer. If you need to support older browsers, a polyfill for this method can be found in the method’s page on MDN. Another polyfill has also been developed by Mathias Bynens.
String.prototype.endsWith()
In addition to String.prototype.startsWith()
, ECMAScript 6 introduces the String.prototype.endsWith()
method. It verifies that a string terminates with a given substring. The syntax of this method, shown below, is very similar to String.prototype.startsWith()
:
String.prototype.endsWith(searchString[, position]);
As you can see, this method accepts the same parameters as String.prototype.startsWith()
, and also returns the same type of values.
A difference is that the position
parameter lets you search within the string as if the string were only this long. In other words, if we have the string house
and we call the method with 'house'.endsWith('us', 4)
, we obtain true
, because it’s like we actually had the string hous
(note the missing “e”).
An example use of this method is shown below:
const str = 'hello!';
const result = str.endsWith('lo!');
// prints "true"
console.log(result);
// verify as if the string was "hell"
result = str.endsWith('lo!', 5);
// prints "false"
console.log(result);
A live demo of the previous snippet is shown below and is also available at JSBin.
The method is supported in Node and all modern browsers, with the exception of Internet Explorer. If you need to support older browsers, a polyfill for this method can be found in the method’s page on MDN. Another polyfill has been developed by Mathias Bynens.
String.prototype.includes()
While we’re talking about verifying if one string is contained in another, let me introduce you to the String.prototype.includes()
method. It returns true
if a string is contained in another, no matter where, and false
otherwise.
Its syntax is shown below:
String.prototype.includes(searchString[, position]);
The meaning of the parameters is the same as for String.prototype.startsWith()
, so I won’t repeat them. An example use of this method is shown below:
const str = 'Hello everybody, my name is Aurelio De Rosa.';
let result = str.includes('Aurelio');
// prints "true"
console.log(result);
result = str.includes('Hello', 10);
// prints "false"
console.log(result);
You can find a live demo below and also as at JSBin.
String.prototype.includes()
is supported in Node and all modern browsers, with the exception of Internet Explorer. If you need to support older browsers, as with the other methods discussed in this tutorial, you can find a polyfill provided by Mathias Bynens (this guy knows how to do his job!) and another on the Mozilla Developer Network.
Note: until version 48, Firefox uses the non-standard name contains
.
String.prototype.repeat()
Let’s now move on to another type of method. String.prototype.repeat()
is a method that returns a new string containing the same string it was called upon but repeated a specified number of times. The syntax of this method is the following:
String.prototype.repeat(times);
The times
parameter indicates the number of times the string must be repeated. If you pass zero you’ll obtain an empty string, while if you pass a negative number or infinity you’ll obtain a RangeError
.
An example use of this method is shown below:
const str = 'hello';
let result = str.repeat(3);
// prints "hellohellohello"
console.log(result);
result = str.repeat(0);
// prints ""
console.log(result);
A live demo of the previous code is shown below and is also available at JSBin.
The method is supported in Node and all modern browsers, with the exception of Internet Explorer. If you need to support older browsers, two polyfills are available for this method: the one developed by Mathias Bynens and another on the Mozilla Developer Network.
String.raw()
The last method I want to cover in this tutorial is String.raw()
. It’s defined as a tag function of template strings
. It’s interesting, because it’s kind of a replacement for templating libraries, although I’m not 100% sure it can scale enough to actually replace those libraries. However, the idea is basically the same as we’ll see shortly. What it does is to compile a string and replace every placeholder with a provided value.
Its syntax is the following (note the backticks):
String.raw`templateString`
The templateString
parameter represents the string containing the template to process.
To better understand this concept, let’s see a concrete example:
const name = 'Aurelio De Rosa';
const result = String.raw`Hello, my name is ${name}`;
// prints "Hello, my name is Aurelio De Rosa" because ${name}
// has been replaced with the value of the name variable
console.log(result);
A live demo of the previous code is shown below and is also available at JSBin.
The method is supported in Node and all modern browsers, with the exception of Opera and Internet Explorer. If you need to support older browsers, you can employ a polyfill, such as this one available on npm.
Conclusion
In this tutorial, you’ve learned about several new methods introduced in ECMAScript 6 that work with strings. Other methods that we haven’t covered are String.fromCodePoint(), String.prototype.codePointAt(), and String.prototype.normalize(). I hope you enjoyed the article and that you’ll continue to follow our channel to learn more about ECMAScript 6.
Frequently Asked Questions about ES6 String Methods
What are the new string methods introduced in ES6?
ES6, also known as ECMAScript 2015, introduced several new string methods to make string manipulation easier and more efficient. These include methods like startsWith()
, endsWith()
, includes()
, repeat()
, and template literals
. The startsWith()
method checks if a string starts with a specified string, while endsWith()
checks if a string ends with a specified string. The includes()
method checks if a string contains a specified string. The repeat()
method repeats a string a specified number of times. Template literals allow you to embed expressions within string literals, using ${}
syntax.
How does the startsWith()
method work in ES6?
The startsWith()
method in ES6 is used to determine whether a string begins with the characters of a specified string. It returns true if the string starts with the specified string and false otherwise. The syntax is str.startsWith(searchString[, position])
. The searchString
is the characters to be searched for at the start of str
. The position
, which is optional, is the position in the string where the search should start. If omitted, the search starts at the beginning of the string.
What is the endsWith()
method in ES6?
The endsWith()
method in ES6 is used to determine whether a string ends with the characters of a specified string. It returns true if the string ends with the specified string and false otherwise. The syntax is str.endsWith(searchString[, length])
. The searchString
is the characters to be searched for at the end of str
. The length
, which is optional, is the length of the string to be searched. If omitted, the length of the string is used.
How does the includes()
method work in ES6?
The includes()
method in ES6 is used to determine whether one string may be found within another string. It returns true if the string contains the specified string and false otherwise. The syntax is str.includes(searchString[, position])
. The searchString
is the string to search for. The position
, which is optional, is the position within the string at which to begin searching. If omitted, the search starts at the beginning of the string.
What is the repeat()
method in ES6?
The repeat()
method in ES6 constructs and returns a new string which contains the specified number of copies of the string on which it was called, concatenated together. The syntax is str.repeat(count)
, where count
is the number of times to repeat the string. The count
must be between 0 and less than infinity and not be a negative number.
How do template literals work in ES6?
Template literals in ES6 are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them. They are enclosed by the backtick (
) character instead of double or single quotes. Template literals can contain placeholders, indicated by the dollar sign and curly braces (${expression}
). The expressions in the placeholders and the text between them get passed to a function.
What is the difference between includes()
and indexOf()
in ES6?
Both includes()
and indexOf()
methods are used to check if a string contains a specified string. However, there is a key difference between them. The includes()
method returns a boolean value – true if the string contains the specified string, and false otherwise. On the other hand, the indexOf()
method returns the index of the first occurrence of the specified string, or -1 if the string is not found.
Can I use ES6 string methods in all browsers?
Most modern browsers support ES6 string methods. However, for older browsers that do not support ES6, you may need to use a transpiler like Babel to convert ES6 code into ES5, which is more widely supported.
How can I use ES6 string methods with arrays?
Some ES6 string methods can be used with arrays. For example, the includes()
method can be used to determine whether an array contains a certain element. However, not all string methods are applicable to arrays. It’s important to understand the specific use and limitations of each method.
What are tagged template literals in ES6?
Tagged template literals in ES6 are more advanced form of template literals. With tagged templates, you can parse template literals with a function. The first argument of a tag function contains an array of string values. The remaining arguments are related to the expressions. The tag function can then perform operations on these arguments and return manipulated string.
I'm a (full-stack) web and app developer with more than 5 years' experience programming for the web using HTML, CSS, Sass, JavaScript, and PHP. I'm an expert of JavaScript and HTML5 APIs but my interests include web security, accessibility, performance, and SEO. I'm also a regular writer for several networks, speaker, and author of the books jQuery in Action, third edition and Instant jQuery Selectors.
Published in
·CMS & Frameworks·Development Environment·Miscellaneous·Patterns & Practices·PHP·September 29, 2014
Published in
·automation·Debugging & Deployment·Development Environment·Meta·Patterns & Practices·PHP·November 29, 2014