After developing WordPress plugins and themes for a while, you start thinking more and more about your theme performance and what problems could it cause for your customers. One of these problems is enqueuing scripts and styles. In this article we’ll cover the basics of enqueuing scripts and best practices for different use cases. You can check this article for a beginner overview on managing your WordPress assets.
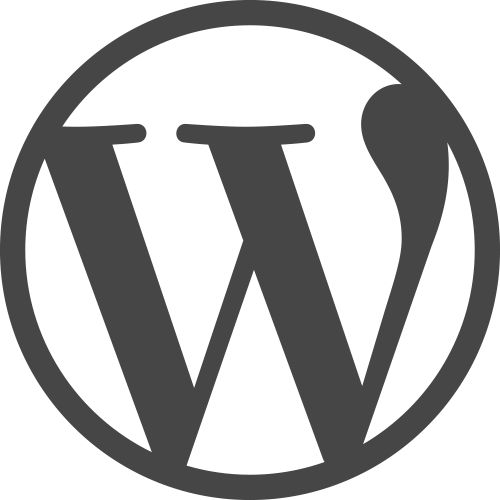
Enqueuing Scripts and Styles
If you need some CSS or JavaScript code to be injected into certain pages of your website, we tend to either add them inside the same markup file or add them using a direct URL to the file.
// ...
<script type="text/javascript">
// some JS code
</script>
<style type="text/css">
// some CSS code
</style>
//...
<script type="text/javascript" src="<?= plugins_url('assets/js/main.js') ?>"></script>
However, this is not the recommended way for adding scripts, and caching plugins won’t optimize and cache your assets to increase website performance. We need to use the admin_enqueue_scripts hook.
add_action( 'admin_enqueue_scripts', function($hook) {
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
} );
The first parameter for the wp_enqueue_script method is the script handler name, we should always prefix the handler to avoid any conflicts and make sure that our script will always be loaded.
The current solution isn’t perfect, and our script is included in every back end page. We can use the $hook
variable passed to the callback function to test for a certain page.
add_action( 'admin_enqueue_scripts', function($hook) {
if ( !in_array($hook, array("options-general.php", "post-new.php")) )
return;
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
} );
Now, our script will only be included inside the settings and new post pages. We can go further to only include our styles when the user is creating a new page.
add_action( 'admin_enqueue_scripts', function($hook) {
if ( $hook == "post-new.php" && isset($_GET['post_type']) && $_GET['post_type'] == "page" )
{
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/main.js'), ['jquery'] );
}
} );
Remove Unnecessary Scripts
Most developers don’t care about enqueuing their scripts just when needed, this is why we’ll always end up getting unexpected errors and weird styling issues. When noticing that a script is throwing an error or causing conflicts, you can remove it before adding your own, this is often useful in debug mode.
add_action( 'admin_enqueue_scripts', function($hook) {
$unautorized_styles = [
'script1',
'another-script'
];
foreach ( $unautorized_styles as $handle )
{
wp_deregister_style( $handle );
}
// enqueue my scripts
} );
Working with Shortcodes
Most plugins and themes provide a set of shortcodes to interact with the website, sometimes they need to inject scripts into the page. Sadly, we cannot test for front end pages as we did previously for the back end.
An easy way to achieve this is to register a callback on the_content
filter and test if it contains your shortcode. If it returns yes, you can enqueue your scripts, otherwise do nothing.
add_filter( 'the_content', function($content) {
if ( has_shortcode($content, "hello-shortcode") )
{
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/hello-shortcode.js') );
}
});
The above code works great, and will only enqueue our scripts for posts containing our shortcode. However, this solution has some serious performance issues on high load websites. We’ll create a more sophisticated solution for this problem.
If you’re not already using Composer to load your classes, I advise you to start doing so. Our plugin composer.json
file would look like the following:
// composer.json
{
"name": "Demo plugin",
"description": "",
"authors": [
{
"name": "Author name",
"email": "author.name@example.com"
}
],
"autoload": {
"psr-4": {
"ESP\\": "src/"
}
}
}
The src/shortcodes/HelloShortcode.php
class holds our shortcode definition.
// src/shortcodes/HelloShortcode.php
namespace ESP\Shortcodes;
class HelloShortcode
{
static $loaded;
public $name;
public function __construct()
{
$this->name = "hello-shortcode";
}
public function run()
{
static::$loaded = true;
return "Hello shortcode!";
}
public function enqueueScripts()
{
if ( static::$loaded == false )
return;
$pluginPrefix = "my-prefix";
wp_enqueue_script( "{$pluginPrefix}main-js", plugins_url('assets/js/hello-shortcode.js') );
}
}
The run
method will return the HTML markup for our shortcode, and will set the loaded
static attribute to true
when the shortcode is used.
The enqueueScripts
method will enqueue our styles when the plugin is loaded. The remaining part will register our shortcode to be recognized inside the post content.
// plugin-file.php
require_once __DIR__."/vendor/autoload.php";
$shortcodes = [
'ESP\\Shortcodes\\HelloShortcode'
];
foreach ($shortcodes as $shortcode) {
$shortcode = new $shortcode;
add_shortcode($shortcode->name, [ $shortcode, 'run' ]);
}
add_action('wp_footer', function() use($shortcodes) {
foreach ($shortcodes as $shortcode) {
(new $shortcode)->enqueueScripts();
}
});
Surely we can avoid putting this code inside the main plugin file, but we’re going to keep things simple and readable.
The $shortcodes
variable holds a list of available shortcodes. The first loop will register our shortcode and make the run
method the handler. Next, we’ll use the wp_footer
hook to enqueue our shortcode scripts before closing the body
tag.
You can now create a new page containing our shortcode and check if our script is loaded properly.
Conclusion
This short article was a summary on how to enqueue your plugin and theme scripts, and the best way to avoid loading them inside every page on your website. If you have any question or comments, please post them below and I’ll do my best to answer them!
Frequently Asked Questions (FAQs) about Enqueuing Scripts and Styles in WordPress
What is the purpose of enqueuing scripts and styles in WordPress?
Enqueuing scripts and styles in WordPress is a best practice that ensures the correct loading order of your scripts and stylesheets. It helps to prevent conflicts between different scripts and styles that your theme or plugins may be using. By enqueuing, you’re telling WordPress when to load the script/style, where to load it, and what other scripts/styles it depends on.
How do I enqueue a script in WordPress?
To enqueue a script in WordPress, you need to use the wp_enqueue_script() function. This function requires at least two parameters: the handle (a unique name for the script) and the src (the URL of the script). You can also specify additional parameters like dependencies, version, and whether to load the script in the footer.
What is the difference between wp_enqueue_script() and wp_register_script()?
Both functions are used to register a script with WordPress, but there’s a key difference. wp_register_script() only registers the script and doesn’t enqueue it. This means the script won’t be printed unless it’s later enqueued with wp_enqueue_script(). On the other hand, wp_enqueue_script() registers and enqueues the script, meaning it will be printed in the HTML.
Can I enqueue styles in WordPress?
Yes, you can enqueue styles in WordPress using the wp_enqueue_style() function. This function works similarly to wp_enqueue_script(), but it’s used for stylesheets instead of scripts.
What is the ‘wp_enqueue_scripts’ action hook?
The ‘wp_enqueue_scripts’ action hook is where you should enqueue your scripts and styles. This hook is called when WordPress is about to print the scripts and styles in the HTML. By hooking into it, you ensure that your scripts and styles are enqueued at the right time.
Why is it important to specify dependencies when enqueuing scripts or styles?
Specifying dependencies ensures that your scripts or styles are loaded in the correct order. If your script depends on another script (like jQuery), WordPress will make sure that jQuery is loaded before your script.
How can I dequeue a script or style in WordPress?
You can dequeue a script or style in WordPress using the wp_dequeue_script() or wp_dequeue_style() function. This is useful if you want to stop a script or style from being loaded.
Can I enqueue scripts and styles in the admin area?
Yes, you can enqueue scripts and styles in the admin area using the ‘admin_enqueue_scripts’ action hook. This works similarly to the ‘wp_enqueue_scripts’ hook, but it’s used for the admin area instead of the front-end.
What is the ‘wp_print_scripts’ action hook?
The ‘wp_print_scripts’ action hook is an older hook that was used to enqueue scripts. However, it’s not recommended to use this hook anymore, as it can lead to issues with script loading order. You should use the ‘wp_enqueue_scripts’ hook instead.
Can I enqueue scripts and styles conditionally?
Yes, you can enqueue scripts and styles conditionally. For example, you can use the is_page() function to only enqueue a script or style if a certain page is being viewed. This can be useful for performance, as it prevents unnecessary scripts and styles from being loaded.

Younes is a freelance web developer, technical writer and a blogger from Morocco. He's worked with JAVA, J2EE, JavaScript, etc., but his language of choice is PHP. You can learn more about him on his website.