Debugging WordPress: 11 Powerful Tips and Techniques
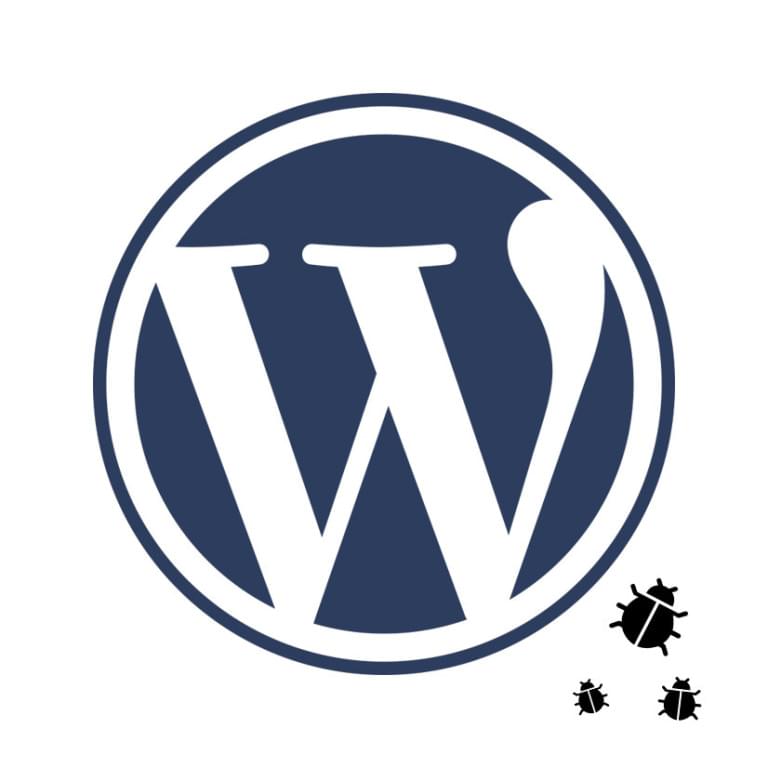
Key Takeaways
- WordPress debugging is a crucial skill for developers, with various tools and techniques available, such as the WP_DEBUG constant, is_wp_error() method, and the Debug Bar plugin, all of which assist in identifying and rectifying errors.
- Other useful debugging methods include creating a test website separate from the live site, using the Simply Show Hooks plugin to identify running hooks, and utilizing PHP Error Logging and a PHP Syntax Checker to spot and fix issues.
- More advanced debugging techniques include using a PHP IDE, such as PhpStorm, for detailed code analysis, and disabling browser cache when working on front-end elements of a website to ensure updates and errors are correctly displayed.
Debugging is an essential skill for any developer. This tutorial will show you 11 powerful ways to debug WordPress and PHP errors.
The first item in the list is the famous WP_Debug, then we’ll jump into some more advanced methods.
First, let’s list the types of common PHP errors:
A – Notice: This is the least important error message you will see with PHP. It does not necessarily mean something is wrong, but there is possible improvement suggested.
Example: null element passed to a function that expect a string.
B – Warning: It’s a more severe error, but does not cause script termination.
Example: giving include() a file that does not exist.
C – Fatal error: This is a dangerous indicator of something going really wrong, and the script terminated.
Example: calling a non-existing function.
1 – WP_DEBUG
WordPress has a global constant to specify the needed level of debugging: WP_DEBUG. Along with this constant, comes two another important ones: WP_DEUBG_DISPLAY, and WP_DEBUG_LOG.
WP_DEBUG is used to set the debugging mode on or off. WP_DEUBG_DISPLAY shows errors or hides them. Finally, WP_DEBUG_LOG saves your error messages in wp-content/debug.log.
The three global constants can be set to true or false in wp-config.php, like this:
define("WP_DEBUG", true);
define("WP_DEBUG_DISPLAY", true);
define("WP_DEBUG_LOG", true);
2 – is_wp_error()
Another WordPress built-in tool for debugging is is_wp_error();. It is a method to check if a certain result is of type WP_Error. WP_Error is actually the returned object you should receive if a WordPress method fails.
Example:
$post = array(
'post_title' => 'Test post',
'post_content' => 'This is my post.',
'post_status' => 'publish',
'post_author' => 1
);
$result = wp_insert_post( $my_post );
if(is_wp_error($result)){
echo $return->get_error_message();
}
The above code will try adding a new post using wp_insert_post(). If this method fails, it will return WP_Error object. You can then catch and get the error message.
3 – Debug Bar
Another useful tool for debugging WordPress errors is the Debug Bar. It is a very handy tool for getting useful information about every page in your website.
After installing it, you will find a new Debug button. When you click on it, an analysis of the queries, templates, PHP installation, and many other useful information.
4 – Test Website
It is of the utmost importance to separate the live website from your tests and development. I usually have two installs of WordPress on my websites. This is important because you don’t want your scripts terminating when you turn on error reporting.
5 – Simply Show Hooks
Simply Show Hooks is a nice plugin for showing every hook that is running on any page. If you encounter a situation where all error reporting ideas are not working, and you will, then comes the time for fetching out every and all running hooks.
This plugin will tell you what action or filter hooks that run on any page. You can then start analyzing and debugging each hook code. You can also see the attached methods to every hook. And even find out the priority of each.
6 – WPDB Error Reporting
If you use the wpdb class for dealing with your database, then you will always need error reporting. Whether it is for making sure that your queries run correctly, or for showing up error messages for debugging.
Example:
global $wpdb;
// Before running your query:
$wpdb->show_errors = TRUE;
$result = $wpdb->get_results("SELECT field_value FROM table_name");
if(! $result){
$wpdb->print_error();
// Or you can choose to show the last tried query.
echo $wpdb->last_query;
}
7 – Server Error Logs
At some points, neither WordPress or PHP will be able to catch some coding errors. For example, if your script exceeded the maximum allowed run time, you won’t get a PHP error message. Rather, Apache (or your server installed system) will pop up something like ‘Internal Server Error.’
This is the time when you should go to your error log, and see what if your PHP code, or a certain part of your WordPress installation did something wrong.
You can consult your web hosting provided on where the logging is stored. Usually it is something under logs folder.
8 – PHP Error Logging
PHP has its own level of error reporting to store the issues resolving beyond WordPress. This is extremely useful, especially if something outside of WordPress running and causing some problem.
You can start by configuring your php.ini file to turn on error reporting, and then choosing where to store such messages.
error_reporting = E_ALL | E_STRICT
error_log = /var/log/php_error.log
Setting the above two lines will turn on error reporting, and set the errors to be saved at the specified path.
Also, you can run phpinfo(); and check the error_log option.
9 – PHP Syntax Checker
If your hosting provider limits access to php.ini file, or you can’t access error logs, things can be a bit harder. But, there are many tools to overcome a situation when you get only a blank page with no error message. One quick tool is PHP Code Checker.
PHP Code Checker is a syntax errors checking tool. It is really useful if you missed a semi-colon or a curly brace, and can’t find where did you miss it.
10 – PHP IDE
If PHP Code Checker didn’t find a syntax error, then you will need a more powerful tool. A powerful IDE like PhpStorm will be the answer for more advanced debugging, and breaking down your code into parts.
In a situation like, when you store a string in a variable, and try printing it, but nothing happens. Using an IDE can find out you are doing something wrong like, overriding this variable later in your code. This is why it is highly recommended to rely on a powerful IDE like PhpStorm, Eclipse, or any tool you prefer.
11 – Disabling Browser Cache
If you are working on the front-end side of your website, then you need to disable browser cache. This is necessary because if you are working on your JavaScript code, and it’s not updating, or even worse, updating but not showing new errors, then you need to disable cache.
Disabling the cache means you ask your browser to stop working on old stored files of your website. This includes CSS and JavaScript files.
To disable Google Chrome browser cache, open the DevTools by right-clicking of any web element. Then, click on Network tab in toolbar. Finally, check the Disable Cache checkbox at the top.
Conclusion
The above 11 tips can be your guide for the debugging process. Although debugging can be tedious at many times, but the above tricks can make the whole process much easier.
Let me know if you have any WordPress debugging tips that you’d like to share, please do so in the comments below!
Frequently Asked Questions about Debugging in WordPress
What is debugging in WordPress and why is it important?
Debugging in WordPress is a way to identify and fix issues that may be causing problems on your website. It involves turning on the WordPress debug mode, which allows you to see any errors or warnings that are occurring on your site. This is important because it helps you to maintain the functionality and performance of your website, ensuring a smooth user experience.
How do I enable debugging in WordPress?
To enable debugging in WordPress, you need to access your website’s wp-config.php file. This can be done through an FTP client or your hosting control panel. Once you’ve accessed the file, look for the line that says “define(‘WP_DEBUG’, false);” and change the ‘false’ to ‘true’. This will turn on the debug mode.
What is the WP_DEBUG_LOG and how do I use it?
The WP_DEBUG_LOG is a tool that allows you to save all error messages to a debug.log file in the wp-content directory. To use it, add the line “define(‘WP_DEBUG_LOG’, true);” to your wp-config.php file. This will enable the logging of errors, which you can then review at your convenience.
What is the WP_DEBUG_DISPLAY and how does it work?
The WP_DEBUG_DISPLAY is a feature that controls whether debug messages are shown inside the HTML of pages or not. By default, it is set to ‘true’, which means that errors will be displayed. However, for live sites, it’s recommended to set it to ‘false’ to prevent the display of errors to visitors.
How can I control the PHP reporting level in WordPress?
You can control the PHP reporting level in WordPress by using the WP_DEBUG and WP_DEBUG_LOG features. By setting WP_DEBUG to ‘true’, you enable the display of PHP errors and warnings. With WP_DEBUG_LOG, you can save these messages to a log file for review.
What should I do if I can’t access my wp-config.php file?
If you can’t access your wp-config.php file, you should contact your hosting provider for assistance. They can help you access the file or make the necessary changes for you.
How can I debug a specific plugin or theme?
To debug a specific plugin or theme, you can use the Plugin Inspector or Theme Check plugins. These tools can help you identify any issues with your plugins or themes that may be causing problems on your site.
What is the SCRIPT_DEBUG and how do I use it?
The SCRIPT_DEBUG is a feature that allows you to use the development versions of core CSS and JavaScript files instead of the minified versions that are normally used. To use it, add the line “define(‘SCRIPT_DEBUG’, true);” to your wp-config.php file.
What should I do if I’m still having trouble after debugging?
If you’re still having trouble after debugging, it may be a good idea to seek professional help. You can contact a WordPress developer or your hosting provider for further assistance.
Is there a risk of damaging my site while debugging?
While debugging can help you identify and fix issues, there is a risk of causing further problems if you’re not careful. Always make sure to back up your site before making any changes, and if you’re unsure about something, seek professional help.
Loai is a web developer with more than seven years of professional experience. He studied computer science and took web development as specialization. Loai currently works at Yellow Media, where he develops yellow pages directory for all business in Egypt. In addition, he works with WordPress for developing themes, plugins, and custom websites. You can contact him on loainagati [at] gmail [dot] com.
Published in
·Debugging·Development Environment·Meta·Patterns & Practices·PHP·Programming·July 1, 2014
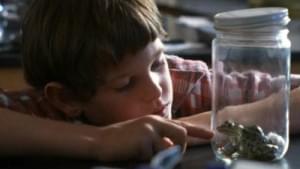
Published in
·Animation·CSS·Design·Design & UX·HTML·HTML & CSS·Illustration·Prototypes & Mockups·UI Design·February 8, 2017