Cloud Storage: Choosing Between Drobpox, Drive, S3 and Others
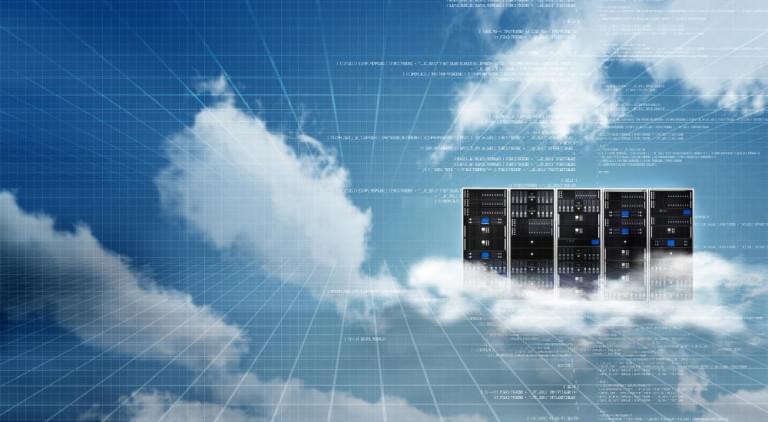
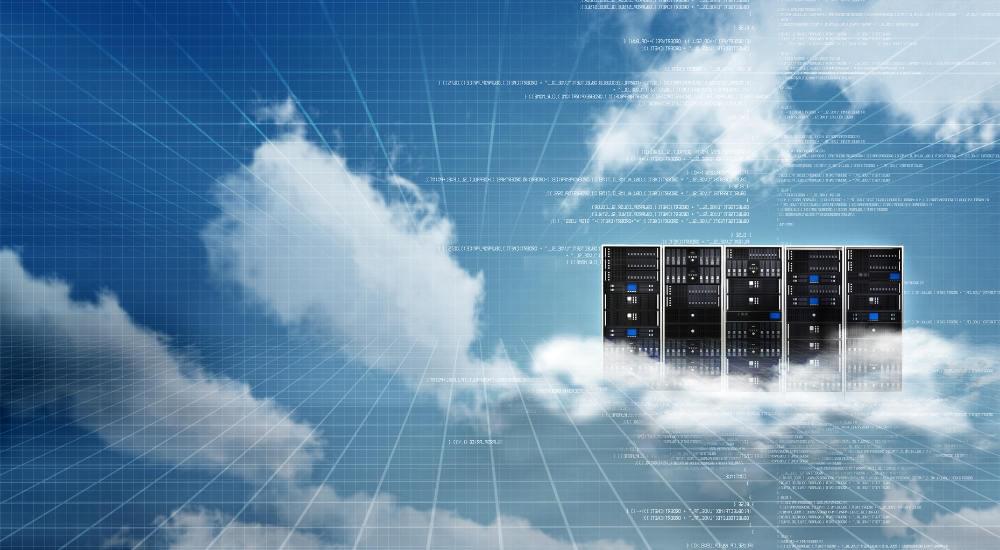
Whether it’s high throughput storage for your very successful app, archiving for your business and project files, or even easily integrated desktop services for sharing data, there’s a service targeted at you to handle 100GB, 100TB, or 100PB just as well.
In our previous installment about cloud services, A Side-by-Side Comparison of AWS, Google Cloud and Azure, we did an overview of what is it that you can do in the cloud, covering topics such as computing, analytics and networking. In this article, we’ll dig deeper into cloud storage, starting from small and very practical requirements for domestic users (desktop services), and scaling up to the needs of businesses, using the same technologies.
Key Takeaways
- Diverse Needs, Diverse Solutions: Whether you need storage for a high-traffic app, business archives, or integrated desktop sharing, cloud services like Dropbox, Google Drive, and Amazon S3 offer scalable solutions for storage needs ranging from 100GB to 100PB.
- Evolving Technology: The shift from traditional single-server storage to multi-server, multi-location cloud storage offers increased redundancy, security, and accessibility, minimizing data loss and latency issues globally.
- Integrated Services Enhance Productivity: Platforms like Google Drive and Dropbox provide not only storage but also tools for document management and collaboration, making them ideal for personal use and enhancing workplace productivity.
- Specialized Storage Options: Specific services cater to different types of data – for instance, Google Photos and iCloud Photo Library offer robust tools for photo storage and management, while services like Amazon Music and Google Play Music address audio storage and streaming needs.
- Business-Focused Features: For enterprise users, cloud storage services include features like easy online collaboration, administrative control panels, and scalable plans, which are crucial for managing company data efficiently and securely.
What Is “the Cloud”?
The “old” way
Not more than just a decade ago, when you needed to upload something to the internet, you would hire a hosting company to rent a certain amount of online space. If you were fancy, you would make an additional backup of files, just in case something happened to that server. And of course, things did happen to servers: hardware failures (it even seemed that hard disk drives (HDD) were just meant to break), crackers (if you ever had an account on a shared hosting, you just knew that every couple of months some foreign flag would appear on your site), and the always unintentional but clumsy overwriting or deletion of files (come on, we all did it).
Now, if you were really fancy, you would deploy your files to different locations at once to ensure redundancy and availability, and to minimize the latency worldwide (that is, the amount of time between you request a file, and when you finally access it). However, doing things the right way was not only costly and time consuming, but it required a fair amount of technical savvy (and we didn’t even get to discuss doing load balancing so that your files would still be available during high traffic situations).
The new way
Today, when you upload a file to a service such as Google Drive, iCloud, Amazon S3 or a hosting grid, this file is automatically replicated in multiple servers and, very often, to multiple locations — so that when you try to access your documents while in Japan or the East Coast of the United States, your request is automatically routed to the closest pool of data that’s available. That is the cloud.
Under this new architecture, if a server or a data center gets disrupted, chances are you won’t ever find out, because the traffic is going to be transparently redirected to the next available pool of data. And even if a server gets cracked, the attackers are not going to come across regular files as in a traditional hosting service, because in many cases the data is obscured in some way and even encrypted, and it’s your request that actually builds the file.
Mind you, this technology — which is pretty much ubiquitous today — made its breakthrough just a decade ago:
- Amazon S3, launched in 2006
- Dropbox, founded in 2007
- iCloud, launched in 2011
- Google Drive, launched in 2012
- … et cetera.
You are already using it
Some people (OK, my ex-girlfriend) are hesitant about using the cloud. “The cloud?” they say. “No thanks!” They don’t like this idea of having their files “everywhere”, and it does sound awkward when you put it that way. But as we mentioned, it’s not quite “your files” that are everywhere, but rather the bits of data that can build your files upon your requests. And if you use services such as Gmail or Yahoo Mail (which is another way of saying “if you’re using the internet”), you are already using the cloud, and you realize how convenient that is.
To explain further: some of you won’t even remember the days when email was downloaded with a client program to a single machine and everything was lost if something happened to that computer, should there be no backup of those files somewhere else. Gmail or Yahoo Mail are just the cloud version of email — a ubiquitous, secure and reliable service that’s replicated around the world for instant access … Yes, the cloud!
Desktop Services
There are tons of services, but for this survey, we’ll be listing some that have been running for a couple of years and that offer full-featured free plans, not just limited trial periods. Some of these services have specific tools, or come integrated with services for certain kinds of storage, such as music files, pictures and documents, which we will cover in detail later in the article. (The w is a link the Wikipedia entry.)
service | docs | pics | music | free | plans | |
---|---|---|---|---|---|---|
Amazon Drive | w | no | yes | yes | 5GB | unlimited/$59.99/yr |
Apple iCloud | w | no | yes | yes | 5GB | 50GB/$0.99/mo; 1TB/$9.99/mo; and more |
iDrive | w | no | limited | no | 5GB | 1TB/$52.12/yr |
Google Drive | w | yes | yes | yes | 15GB | 100GB/$1.99/mo; 1TB/$9.99/mo; and more |
Dropbox | w | yes | limited | no | 2GB | 1TB/$9.99/mo |
OneDrive | w | yes | limited | yes | 5GB | 50GB/$1.99/mo; 1TB/$6.99/mo |
Pay attention to how you count space! All of the space you’re using on the same provider will count as a single resource. For example, if you’re using 5GB on Gmail and 2GB on files stored on Google Drive, that means you’re using a total of 7GB, so your available space in case you’re using the free tier would be:
15GB (total space)
- 5GB (from Gmail)
- 2GB (from Google Drive)
= 8GB available
iCloud is more of a backup service for the iPhone and the Mac rather than a full-featured cloud service, and as such is not available for non-Apple products. In fact, Apple has earned a poor reputation when in comes to interoperability and, while it’s convenient in Apple environments, you’ll come across a number of problems if you ever need to change to Android or temporarily switch to a PC.
On the other hand OneDrive (formerly SkyDrive) is not exclusively a Windows tool, even though it’s developed by Microsoft. You can freely use it on a Mac, your iPhone, and on Linux environments such as Android phones.
Documents
The field for productivity is certainly that of office documents. You can store these files in any storage service. After all, they’re just files. However, we’re listing here services that, besides storage, also give you the possibility to manage documents right there, allowing users to create, edit, and share documents.
Google Drive is the home for Google Docs, Sheets, Slides and Forms. All of them are mature tools that you can use without limitations and for free through your browser. And Google has really managed to make collaborative editing in real-time a true highlight of its office suite.
Late but determined, Microsoft joined the online club of productivity tools. You can store your Office documents in OneDrive and have a limited editing and free visualization via Office Online, the free version of Office 365. The licensing scheme for the full-featured products is way to complex to reproduce here, but have a look at it if what you need is full support for Microsoft Office products.
Paper is the all-in-one work solution by Dropbox. It’s a fairly limited tool, specially when compared against Google’s tools or the full-fledged Office 365. But its simplicity might also be its main advantage, given that it’s a very straightforward tool to use that requires little training. And it does support collaboration, rich formatting and, of course, Dropbox attachments.
There are some workarounds and plans to integrate iCloud with iWork, Apple’s office suite, which would bring document editing capabilities to the iCloud service. For now, though, we count it as a missing feature.
Pictures
If there is a backup service you should start using now, this is it. It’s sad to know that still today many families keep their treasured memories on a desktop or laptop computer hard drive. If I wasn’t clear before, I’ll try to make it clear now: the hard drive of a computer (especially the traditional HDD) is a bomb about to explode. And even if you have a more reliable solid-state drive (SSD), still, your laptop could get stolen, get a virus, or disappear into the blue (why not?).
When it comes to storing and sharing pictures, for some of you Facebook may do just fine, but it does fall short on features: you can’t order prints, you can’t search by date, or edit. And what if you decided one day to get rid of Facebook altogether?
As a solution, all of the services we’ll cover here will automatically upload for you every new pic or video that you take on your phone or that you sync from your camera to your computer, thus enabling you to clean up space very quickly and allowing you to select later what you keep or not.
service | free space | plans | |
---|---|---|---|
Amazon Prime Photos | w | 5GB | unlimited/$59.99/yr |
Flickr | w | 1TB | 2TB/$5.99/mo |
Google Photos | w | unlimited | 100GB/$1.99/mo; 1TB/$9.99/mo; and more |
iCloud Photo Library | w | 5GB | 50GB/$0.99/mo; 1TB/$9.99/mo; and more |
Dropbox used to bundle a decent photo service called Carousel that they unfortunately decided to shut down in early 2016. They still have support for automatically uploading of photos from your phone and visualizing them with an app or the browser, but features come nowhere close to those of these other services. Microsoft also hasn’t integrated OneDrive with a dedicated photo service so far.
Flickr is arguably the place for sharing photos, with the biggest community of pros and amateur photo enthusiasts, a website that’s been running and adding features since 2004, and great organization tools and privacy settings.
iCloud comes with a tool called iCloud Photo Library, and Google Drive comes integrated with Google Photos (formerly Picasa Web Albums). Both are full-featured photo services for tagging people, sharing and creating albums, and even editing pictures, along with many more features.
A huge plus is that Google Photos gives you unlimited storage for pictures for free (that is, without subtracting space from your Google Drive account) as long as you don’t need to store the originals for pictures in resolutions higher than 16 megapixels. Should you choose this option, it will shrink your hi-res pics to 2048×2048 (in other words, OK for most domestic uses).
A fairly limited product with few organizational tools and no search, Amazon Prime Photos (paid) is the most generous when it comes to storage — unlimited for all images, including hi-res.
Music
You can also use the cloud for storing your ripped CDs and MP3 files. You’ll have the benefit of being able to stream your music from everywhere for free with your phone or computer, and forget altogether about the idea of bringing your files on USB drives and other media whenever you want to take your music somewhere else.
Aside from streaming, all of these services allow you with certain limitations to upload your own music collection:
service | free upload | w/subscription | plans | |
---|---|---|---|---|
Amazon Music | w | 250 | 250,000 | $25/yr (storing only) |
Groove Music | w | up to 5 GB | unlimited | $7/mo (streaming and storing) |
Google Play Music | w | 50,000 | 50,000 | $9.99/mo (streaming and storing) |
iTunes Match | w | no | 100,000 | $25/yr (storing only) |
Some of these services also include family packages so that different users can simultaneously access the service for a reduced fee. This is the case with the Google Play Music family plan, allowing unlimited access up to six family members for $14.99/mo.
Notice that, when you pay a streaming subscription, you also have access to millions of songs in high definition audio. But as we covered here, this is not a requirement for uploading your own music files to the cloud.
And talking about music subscription services, we won’t discuss services such as Apple Music , Spotify, Deezer or Tidal, as these are intended strictly for streaming (that is, you pay a fee to stream music on demand) with no option for uploading and storing your own music library.
Google allows for uploading local music to the cloud with the Music Manager tool. The subscription to Google Play Music also includes YouTube Red, which is the ad-free version of YouTube, along with access to YouTube Red Original series and films.
Amazon Music also allows uploading music, but through an app. Although Amazon shut down its Music Importer tool, they didn’t entirely get rid of the option to upload music, which can be done now with the Amazon Music app.
iTunes Match will automatically try to match your local collection to what’s available on iTunes, and it will automatically add to your online library whatever matches without you needing to upload any actual files. However, you need to pay a subscription to be able to use this feature.
Uploading files to Groove Music is a bit more of a hassle, and you need to keep space in mind. First, there’s no way to match your collection as with iTunes, so you’ll need to upload files individually or sync your Music
folder to OneDrive. Second, and unlike the previously mentioned services, here you’ll be using up space from your cloud storage account, in this case OneDrive. However, if you’re already paying for a OneDrive subscription, this may be OK with you; same if you’re paying the $7/mo subscription for Groove Music.
Storage for Business
There are very interesting options for those who like the simplicity and practicality of the desktop services we described, and would like to take just that to the working environment.
These services may be very attractive for companies, because some of the functionalities we already described plus some of these extras:
- easy online collaboration (which is a huge plus for the enterprise)
- no APIs to integrate;
- in general, no additional training for users (for example, if you already use Google Docs, you’re good to go)
- control panels for administrators (to manage users’ access, space quotas, and other features).
service | space | plans, per user |
---|---|---|
Box | 100GB (starter); unlimited (business) | €4/mo (starter); €12/mo (business) |
iDrive Business | 250GB (A); 1.25TB (C) | $74.62/yr (A); $374.62/yr (C) [unlimited users] |
Dropbox Business | unlimited | $15/mo |
Google Drive for Work | 1TB (for less than 5 users) | $10/mo |
OneDrive for Business | 1TB (plan 1); 5TB (plan 2) | $5/mo (plan 1); $10/mo (plan 2) |
Be careful when evaluating the total cost of these services, as you’ll need to take into account both your storage requirements and the amount of employees.
Cloud Storage for Websites, Apps and Archiving
There are, of course, services oriented towards storing objects for websites and applications, as well as online archiving, and even building a content delivery network (CDN). For details about these services, you should see the “Storage” section of “A Side-by-Side Comparison of AWS, Google Cloud and Azure“.
Conclusions
We’ve seen plenty of options for using the cloud to store your digital assets, including free and very rich-featured ones. If you’re a domestic user who wants to keep it simple, you might be better off choosing a desktop service and sticking to it for all of your needs. In addition, or as an alternative, you might pick a specific service for a specific type of storage (say, Flickr for pictures). And in some cases, you’ll be able to smoothly transfer these practices and tools to your company.
In any case, don’t risk losing files and information you value, and keep your music, pictures and documents in a safe, accessible place. The cloud is your friend.
Frequently Asked Questions on Cloud Storage for You and Your Business
What are the key differences between Amazon S3 and Google Cloud Storage?
Amazon S3 and Google Cloud Storage are both popular cloud storage services, but they have some key differences. Amazon S3 is known for its scalability, reliability, and security features. It offers a wide range of storage classes to meet different needs and budgets. On the other hand, Google Cloud Storage is recognized for its high performance and integration with other Google services. It also offers a unified, consistent API for all storage classes, making it easier to manage.
How does the pricing of Amazon S3 and Google Cloud Storage compare?
Both Amazon S3 and Google Cloud Storage have a pay-as-you-go pricing model, but the costs can vary depending on the storage class, data transfer, and additional features used. Amazon S3 pricing is based on the amount of data stored, the number of requests made, and the region. Google Cloud Storage pricing is also based on the amount of data stored, network usage, and operations performed. It’s important to calculate the total cost of ownership considering all these factors.
Which cloud storage service is more secure, Amazon S3 or Google Cloud Storage?
Both Amazon S3 and Google Cloud Storage offer robust security features to protect your data. Amazon S3 provides features like encryption, access control policies, and versioning. Google Cloud Storage also offers encryption, identity and access management, and audit logging. The choice between the two often comes down to your specific security requirements and the ecosystem you are more comfortable with.
How does the performance of Amazon S3 and Google Cloud Storage compare?
Both Amazon S3 and Google Cloud Storage are designed to deliver high performance. However, the performance can vary depending on factors like the size of the data, the number of requests, and the network conditions. Some users have reported faster upload and download speeds with Google Cloud Storage, while others prefer the consistent performance of Amazon S3.
Can I switch from Amazon S3 to Google Cloud Storage or vice versa?
Yes, it’s possible to switch from Amazon S3 to Google Cloud Storage or vice versa. Both services provide tools and documentation to help you migrate your data. However, it’s important to consider the potential costs, time, and complexity involved in the migration process.
Which cloud storage service offers better integration with other services?
Both Amazon S3 and Google Cloud Storage offer excellent integration with their respective ecosystems. Amazon S3 integrates seamlessly with other AWS services like EC2 and Lambda. Google Cloud Storage integrates well with other Google services like Compute Engine and BigQuery. The choice often depends on which ecosystem you are already using or plan to use.
What are the storage classes offered by Amazon S3 and Google Cloud Storage?
Amazon S3 offers several storage classes including Standard, Intelligent-Tiering, One Zone-IA, and Glacier for different use cases and budgets. Google Cloud Storage offers four storage classes: Standard, Nearline, Coldline, and Archive, each designed for different access patterns and storage durations.
How reliable are Amazon S3 and Google Cloud Storage?
Both Amazon S3 and Google Cloud Storage are designed for high durability and availability. Amazon S3 promises 99.999999999% durability and 99.99% availability. Google Cloud Storage also promises 99.999999999% durability and offers 99.95% availability for its Standard storage class.
How easy is it to use Amazon S3 and Google Cloud Storage?
Both Amazon S3 and Google Cloud Storage are designed to be user-friendly. They offer web-based interfaces, command-line tools, and SDKs for various programming languages. However, some users find Google Cloud Storage’s unified API and integration with Google services to be more convenient.
Which cloud storage service is better for my business, Amazon S3 or Google Cloud Storage?
The choice between Amazon S3 and Google Cloud Storage depends on your specific needs and circumstances. Factors to consider include the size and type of your data, your budget, your security requirements, the other services you use, and your personal preference. It’s recommended to try both services and see which one works best for your business.
Lucero is a programmer and entrepreneur with a feel for Python, data science and DevOps. Raised in Buenos Aires, Argentina, he's a musician who loves languages (those you use to talk to people) and dancing.