Quick Tip: Convenience Hacks for Passing Data to Views

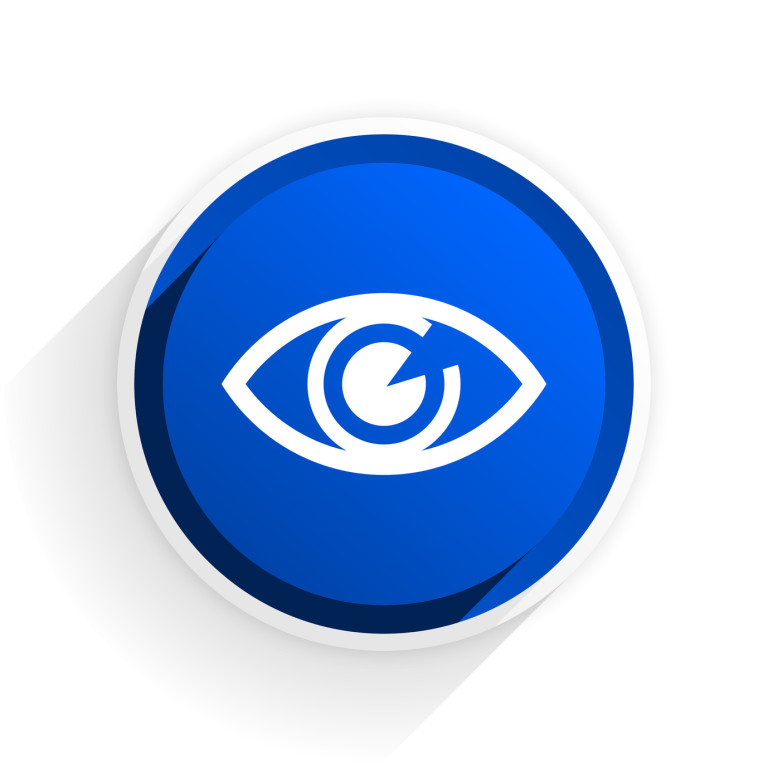
In MVC based architectures, working with template engines is an inevitable part of the development routine. It usually goes like this: we prepare and pass the data to the view. In the view, we print them based on our layout design.
Here is a basic example of how it works using Twig.
<?php
// Twig initialization
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$twig->render('author.page', [
'user' => $user,
'posts' => $posts,
'comments' => $comments,
]);
// ...
There are times, however, when the number of variables might be much higher than this: ten or more. In that case, we’ll have a tall list of variables (as an associative array), being passed to the respective template. It gets messy and unreadable quickly. If only there was a way to just list what we need by name, and have PHP take care of the rest for us. Well… there is!
In this post, we’re going to talk about a trick for passing the defined variables to the view in a somewhat more convenient way: we can use the PHP native compact() function to handpick the data we need, by referring to the variables by name:
<?php
// Twig initialization
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$twig->render('author.page', compact('user', 'posts'));
// ...
compact()
accepts a list of names, looks for variables with those names (within the current scope) and returns them all as one associative array.
Sometimes it’s even more convenient to specify the variables we don’t need instead of those we do. Since there’s no built-in solution in PHP to compact all the variables (within the current scope) with a few of them excluded, we have to do this as a three-step process.
First we need to get all the variables within the controller’s scope with get_defined_vars()
:
<?php
// ...
$variables = get_defined_vars();
// ...
Then, we filter out the unwanted variable names from wanted ones, by using PHP’s array_diff function.
array_diff
compares an array against one or more arrays, and returns the entries in the first array, which are not present in any of the other arrays.
<?php
// ...
$variables = get_defined_vars();
$including = array_diff(array_keys($variables), ['user', 'posts', 'comments']);
// ...
Finally, we can extract our desired variables (listed in $including
) out of $variables
by using array_intersect_key()
.
array_intersect_key()
accepts a number of arrays and returns the entries of the first array the keys of which exist in all the other arrays.
Please note since array_intersect_key()
compares keys to get the intersection of the arrays, we need to switch the keys with their associated values in $including
by using array_flip():
<?php
// ...
$variables = get_defined_vars();
$including = array_diff(array_keys($variables), ['user', 'posts', 'comments']);
$vars = array_intersect_key($variables, array_flip($including));
// ...
Now, to make the procedure reusable, we can abstract the complexity into a helper function:
<?php
//Helpers.php
// ...
if (!function_exists('only_compact')) {
function only_compact($values, $keys) {
$keys = array_diff(array_keys($values), $keys);
return array_intersect_key($values, array_flip($keys));
}
And this is how it will make things easier:
<?php
// Twig initialization...
// Preparing data
$user = 'user data';
$posts = 'posts';
$comments = 'comments';
$anotherOne = 'some value';
$yetAnotherOne = 0;
$andAnotherOne = 0;
$counter = 0;
$test_1 = 1;
$test_2 = 2;
$test_3 = 3;
$test_4 = 4;
$test_5 = 5;
$test_6 = 6;
// Even more variables ...
$twig->render('author.page', only_compact(get_defined_vars(), ['counter', 'twig']));
);
// ...
As a result, we get an array of variables with all the unwanted variables excluded.
To use the helper file, we can add it under files
(under autoload
) in composer.json
:
// ...
"autoload":{
"files": [
"helpers.php"
]
}
// ...
Since we’re using PHP’s built-in functions, the performance impact isn’t noticeable. Below is the result of a quick profile test done with Blackfire, before and after using the above techniques:
Without using the functions:
With using the functions:
As you can see, the results of both profile runs are almost the same.
Hopefully, this trick will save you some typing!
Have any other tips to share in regards to templating and view efficiency? Let us know!
Frequently Asked Questions (FAQs) on Passing Data to Views
How can I pass data to views in a more efficient way?
There are several ways to pass data to views more efficiently. One of the most common methods is to use the ‘compact’ function in PHP. This function allows you to pass multiple variables to a view without having to specify each one individually. For example, instead of writing return view('page', ['name' => $name, 'age' => $age]);
, you can simply write return view('page', compact('name', 'age'));
. This can save a lot of time and make your code cleaner and easier to read.
What is the difference between associative arrays and objects in Twig?
In Twig, associative arrays and objects are both used to store data, but they work in slightly different ways. An associative array is a type of array that uses keys instead of numerical indexes. This can make your code easier to understand, as you can use meaningful names for your keys. On the other hand, an object is an instance of a class, which can contain properties and methods. You can access the properties of an object using the dot notation, like this: object.property
.
How can I loop through an associative array in Twig?
Looping through an associative array in Twig is quite straightforward. You can use the ‘for’ loop, like this: {% for key, value in array %} {{ key }}: {{ value }} {% endfor %}
. This will iterate over each key-value pair in the array, allowing you to access both the key and the value within the loop.
What are some useful Twig functions I should know about?
Twig comes with a number of built-in functions that can make your life easier. For example, the ‘merge’ function allows you to combine two or more arrays into one. The ‘length’ function can be used to get the number of items in an array or the length of a string. The ‘dump’ function is useful for debugging, as it outputs the contents of a variable in a readable format.
How can I use the ‘merge’ filter in Twig?
The ‘merge’ filter in Twig allows you to combine two or more arrays into one. You can use it like this: {% set array = array1|merge(array2) %}
. This will create a new array that contains all the elements from both ‘array1’ and ‘array2’. If both arrays have a key with the same name, the value from ‘array2’ will overwrite the value from ‘array1’.
How can I display associative array data in Twig?
To display associative array data in Twig, you can use the ‘for’ loop. Here’s an example: {% for key, value in array %} {{ key }}: {{ value }} {% endfor %}
. This will iterate over each key-value pair in the array, and print out the key and value.
How can I access object properties in Twig?
In Twig, you can access object properties using the dot notation. For example, if you have an object called ‘user’ with a property called ‘name’, you can access the name like this: {{ user.name }}
. If the property doesn’t exist, Twig will return null and won’t throw an error.
How can I use the ‘length’ function in Twig?
The ‘length’ function in Twig can be used to get the number of items in an array or the length of a string. You can use it like this: {{ array|length }}
or {{ string|length }}
. This will return the number of items in the array or the number of characters in the string.
How can I use the ‘dump’ function in Twig for debugging?
The ‘dump’ function in Twig is a useful tool for debugging. It outputs the contents of a variable in a readable format. You can use it like this: {% dump variable %}
. This will print out the contents of ‘variable’, which can be very helpful when you’re trying to figure out what’s going on in your code.
How can I pass multiple variables to a view in Laravel?
In Laravel, you can pass multiple variables to a view using the ‘with’ method or the ‘compact’ function. The ‘with’ method allows you to chain multiple variables together, like this: return view('page')->with('name', $name)->with('age', $age);
. The ‘compact’ function allows you to pass multiple variables in a more concise way, like this: return view('page', compact('name', 'age'));
. Both methods achieve the same result, so you can choose the one that you find most readable and convenient.
A web developer with a solid background in front-end and back-end development, which is what he's been doing for over ten years. He follows two major principles in his everyday work: beauty and simplicity. He believes everyone should learn something new every day.
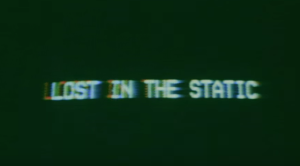
Published in
·automation·Debugging & Deployment·Development Environment·Libraries·Performance·PHP·Standards·Testing·September 7, 2016
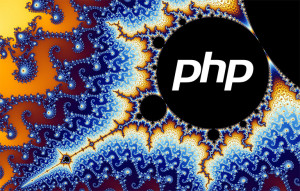
Published in
·APIs·automation·CMS & Frameworks·Frameworks·Laravel·Libraries·Patterns & Practices·PHP·Standards·February 25, 2017
Published in
·Databases·Debugging & Deployment·Development Environment·Extensions·PHP·Programming·June 12, 2014