Time to promote some open source projects again! This time we’ve got an alternative to Laravel, but one that requires PHP7 (awesome!), some packages that really care about request validation and query param filtering, HPKP (security upgrades for everyone!), a package that makes your objects stricter and finally, a treat from the people who made Symfony!
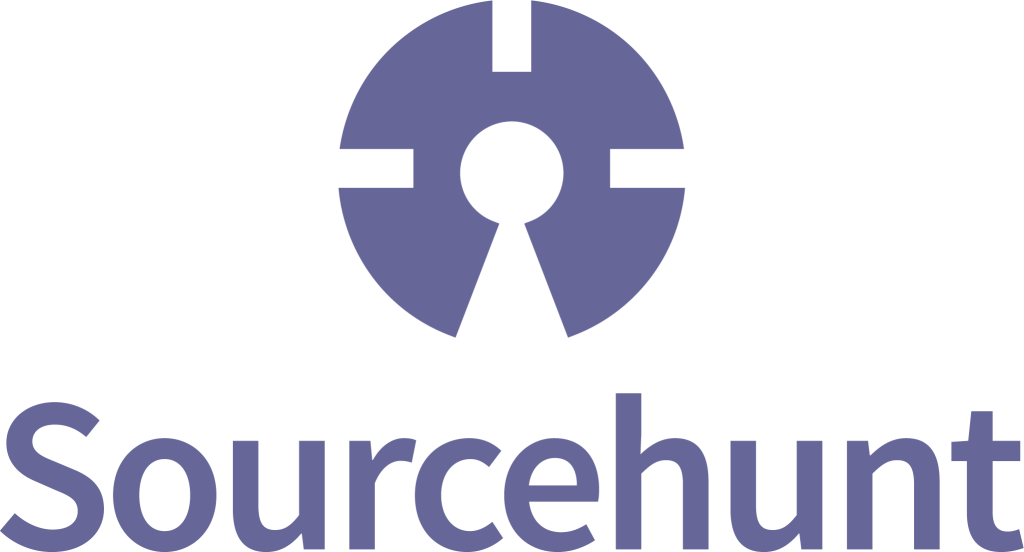
paragonie/hpkp-builder [15 ★]
This library aims to make it easy to build HTTP Public-Key-Pinning headers in your PHP projects, and requires at least PHP 7. HTTP Public Key Pinning, or HPKP, is a security policy delivered via a HTTP response header much like HSTS and CSP. It allows a host to provide information to a user agent about which cryptographic identities it should accept from the host in the future. This can protect a host website from a security compromise at a Certificate Authority where rogue certificates may be issued for your hostname. Read more about HPKP here.Rican7/incoming [137 ★]
Incoming is a PHP library designed to simplify and abstract the transformation of loose, complex input data into consistent, strongly-typed data structures.// Create our incoming processor
$incoming = new Incoming\Processor();
// Process our raw form/request input into a User model
$user = $incoming->process(
$_POST, // Our HTTP form-data array
new User(), // Our model to hydrate
new UserHydrator() // The hydrator above
);
Explaining it to any great detail is outside the scope of this short post, but in essence it allows us to precisely define what kind of input information goes through and hydrates our model, rejecting, filtering, or transforming everything else.
It’s like Fractal, backwards. (Fractal makes sure the output matches a set structure, rather than input)
The library currently has one outstanding issue – and it’s a discussion around a feature – but could definitely use some users and feedback! Maybe even a SitePoint post about it?
LinioIT/input [18 ★]
Yet another input filtering mechanism, this component of the Linio framework (?!) aims to abstract HTTP request input handling, allowing a seamless integration with your domain model. The component is responsible for:- Parsing request body contents
- Validating input data
- Hydrating input data into objects
class RegistrationHandler extends InputHandler
{
public function define()
{
$this->add('referrer', 'string');
$this->add('registration_date', 'datetime');
$user = $this->add('user', 'Linio\Model\User');
$user->add('name', 'string');
$user->add('email', 'string');
$user->add('age', 'integer');
}
}
and then reference that in a controller:
class RegistrationController
{
public function registerAction(Request $request): Response
{
$input = new RegistrationHandler();
$input->bind($request->request->all());
if (!$input->isValid()) {
return new Response($input->getErrorsAsString());
}
$data = $input->getData();
$data['referrer']; // string
$data['registration_date']; // \DateTime
$data['user']; // Linio\Model\User
return new Response(['message' => 'Valid!']);
}
}
The library also supports types, constraints, transformers, and more – all about which you can read in the docs.
mpscholten/request-parser [18 ★]
On a similar note, request-parser does something more lightweight.public function index()
{
$page = $this->queryParameter('page')->int()->required();
$order = $this->queryParameter('order')->oneOf(['asc', 'desc'])->required();
$createdAt = $this->queryParameter('createdAt')->dateTime()->defaultsTo(null);
}
Simple, right? Define required and optional parameters, with types, directly on your current request. There’s more to it, of course, but this is the gist of it.
With a mere 18 stars, this library could definitely use some attention – both in terms of users, and in terms of contributors / testers.
opulencephp/Opulence [322 ★]
Opulence could be the competition Laravel has been waiting for. It’s a full stack framework with a minimum requirement of PHP 7.0. As the docs say:Opulence is a PHP web application framework that simplifies the difficult parts of creating and maintaining a secure, scalable website. With Opulence, things like database management, caching, ORM, page templates, and routing are a cinch. It was written with customization, performance, and best-practices in mind. Thanks to test-driven development (TDD), the framework is reliable and thoroughly tested. Opulence is split into components, which can be installed separately or bundled together.Like Laravel, it comes with its own components all around and its author is very determined to keep it cutting edge. Opulence blew up on Reddit the other day, collecting generally favorable reviews, so the star count isn’t as low as on other projects we’re mentioning in this post, but it could still use contributors if we want to give it the inertia needed to bite at Laravel’s heels.
zeeshanu/yell [7 ★]
Yell is a PHP package to make your objects strict and throw exceptions when you try to access or set some undefined property in them.use Zeeshanu\Yell\Scream;
class Person
{
use Scream;
public $name;
public $age;
}
$person = new Person();
$person->name = 'John Doe';
$person->age = 23;
// An exception will be thrown when showing message "Trying to set undefined property $profession in class Person"
$person->profession = 'Teacher';
sensiolabs-de/deptrac [355 ★]
Deptrac is a new tool from Sensiolabs (Symfony), and is a static code analysis tool that helps to enforce rules for dependencies between software layers. What does this mean specifically? For example, you can define a rule like “controllers may not depend on models”. To ensure this, deptrac analyzes your code to find any usages of models in your controllers and will show you where this rule was violated. As an introduction, here’s a handy video they prepared: Admittedly, this package is a little less indie than the others, but seems interesting enough to warrant promotion – we’d definitely like to see some use cases for it in tutorials. Care to write some?That’s it for June – as always, please throw your links at us with the hashtags #sourcehunt and #php – here’s the link to the combination. Naturally, if you’d like to sourcehunt a project written in another language, alter accordingly. Happy coding!
Frequently Asked Questions about PHP7 and Laravel Alternatives
What are some of the best alternatives to Laravel for PHP7?
There are several alternatives to Laravel for PHP7. Some of the most popular ones include Symfony, CodeIgniter, CakePHP, Yii, and Zend Framework. Each of these frameworks has its own strengths and weaknesses, and the best choice depends on your specific needs and preferences. For example, Symfony is known for its flexibility and scalability, while CodeIgniter is praised for its simplicity and performance. CakePHP offers a robust set of tools for building web applications, while Yii excels in terms of speed and efficiency. Zend Framework, on the other hand, is a powerful enterprise-level framework that offers a wide range of features and capabilities.
How does Laravel compare to other PHP frameworks in terms of performance?
Laravel is known for its elegant syntax and rich set of features, but it’s not necessarily the fastest PHP framework in terms of performance. Other frameworks like Phalcon and Yii are often cited as being faster. However, Laravel’s performance is more than adequate for most web applications, and it offers other advantages such as ease of use, a vibrant community, and a wide range of packages that can extend its functionality.
Can I use Laravel with PHP 7.0?
Yes, Laravel is compatible with PHP 7.0. However, it’s recommended to use the latest version of PHP for the best performance and security. Laravel 5.5 and later versions require PHP 7.0 or higher.
What are the main differences between Laravel and Symfony?
Laravel and Symfony are both popular PHP frameworks, but they have some key differences. Laravel is known for its simplicity and ease of use, making it a great choice for beginners. It also has a more active community and a larger number of packages available. Symfony, on the other hand, is more flexible and modular, making it a good choice for complex, enterprise-level applications. It also follows the PHP-FIG standards more closely than Laravel.
What are the benefits of using PHP7 over older versions of PHP?
PHP7 offers several improvements over older versions of PHP. It’s significantly faster, which can lead to better performance for your web applications. It also introduces new features like scalar type declarations, return type declarations, null coalescing operator, spaceship operator, and more. Additionally, PHP7 has improved error handling and has removed several deprecated functions and features, making your code cleaner and more efficient.
How can I migrate my Laravel application to PHP7?
Migrating a Laravel application to PHP7 usually involves updating your server to run PHP7, updating your composer.json file to require PHP7, and then updating your code to take advantage of the new features and improvements in PHP7. It’s also important to thoroughly test your application after the migration to ensure everything works as expected.
What is HPKP and how does it relate to Laravel and PHP7?
HPKP, or HTTP Public Key Pinning, is a security feature that can prevent man-in-the-middle attacks by associating a specific cryptographic public key with a certain web server. Laravel and PHP7 don’t directly support HPKP, but you can implement it in your web server or use a package to add HPKP support to your Laravel application.
What are the main features of Laravel?
Laravel offers a wide range of features that make it a powerful and flexible framework for web development. Some of its main features include an ORM (Object-Relational Mapping) for database operations, a routing system, a templating engine called Blade, authentication and authorization systems, a task scheduler, and more. It also has a package system called Composer for managing dependencies, and it integrates with many popular tools and services.
How does Laravel handle database migrations?
Laravel provides a powerful migration system that allows you to version control your database schema. This makes it easy to modify your database structure and apply those changes across multiple development environments. Laravel’s migrations are paired with a schema builder to provide a database-agnostic way of building and modifying tables.
What is the learning curve like for Laravel compared to other PHP frameworks?
Laravel is often praised for its ease of use and simplicity, which makes it a good choice for beginners. It has a clear and concise syntax, and it provides a lot of functionality out of the box. However, like any framework, it does require some time to learn, especially if you’re new to PHP or MVC frameworks. Other frameworks like CodeIgniter or CakePHP might have a slightly lower learning curve, but they may not offer the same level of functionality or flexibility as Laravel.
Bruno is a blockchain developer and technical educator at the Web3 Foundation, the foundation that's building the next generation of the free people's internet. He runs two newsletters you should subscribe to if you're interested in Web3.0: Dot Leap covers ecosystem and tech development of Web3, and NFT Review covers the evolution of the non-fungible token (digital collectibles) ecosystem inside this emerging new web. His current passion project is RMRK.app, the most advanced NFT system in the world, which allows NFTs to own other NFTs, NFTs to react to emotion, NFTs to be governed democratically, and NFTs to be multiple things at once.