Features
- Enter your JSON either by Uploading a JSON File or Copy and Paste.
- Expandable tree view.
- Hover nodes to see the path of the variable.
- Click to copy the full path of the node.
- Specify a custom delimiter to the copy node path.
Example Create JSON Tree Calls
If you want to create your own Trees this is how you can create them. JSONTREEVIEWER is the main namespace.$(function () {
//Initialise JQUERY4U JSON Tree Viewer
JSONTREEVIEWER.init();
//Events to load example files
$('#example1').bind('click', function () {
JSONTREEVIEWER.processJSONTree('one-level.json');
});
});
Main Function to Process JSON Tree
This function determines where to get the JSON from: 1) file upload; or 2) copy and paste; 3) example files./*Load the JSON file either by upload or example file and process tree*/
processJSONTree: function (filename) {
$('#loading').show();
var data = '',
branches = '';
if (filename === 'copyandpastejson') {
var copypastejson = $('#copyandpastejson').val(); /*validate JSON*/
if (JSONTREEVIEWER.isValidJSON(copypastejson)) {
data = copypastejson;
} else {
return false;
}
if (data === false) {
return false;
} /*Build JSON Tree*/
JSONTREEVIEWER.buildTree(JSONTREEVIEWER.processNodes(jQuery.parseJSON(data)), filename);
} else {
//get the JSON file from file upload
$.ajax({
type: 'GET',
url: filename,
async: false,
beforeSend: function (x) {
if (x && x.overrideMimeType) {
x.overrideMimeType('application/j-son;charset=UTF-8');
}
},
dataType: 'json',
success: function (data) { /*Build JSON Tree*/
JSONTREEVIEWER.buildTree(JSONTREEVIEWER.processNodes(data), filename);
},
error: function (e) { /*Invalid JSON*/
alert('Invalid JSON: ' + e.responseText);
JSONTREEVIEWER.showErrorMsg();
return false;
}
});
}
},
Build Tree Function
This function constructs the tree from the branches and displays them on the page./*Build JSON Tree*/
buildTree: function (branches, filename) {
//console.log('branches' + branches);
if (typeof branches !== 'undefined' || branches !== '') {
$('#browser').empty().html(branches);
$('#browser').treeview({
control: '#treecontrol',
add: branches
});
$('#selected_filename').html('(' + filename + ')');
$('#loading').hide();
$('#browser-text').hide();
} else {
$('#selected_filename').html('Please select JSON file above...');
$('#loading').hide();
}
},
JSON Branch Recursion Function
This function is fairly complex and took a while to code. It basically takes each JSON object recursively, determines is type and creates the HTML for the branches./*Process each node by its type (branch or leaf)*/
processNodes: function (node) {
var return_str = '';
switch (jQuery.type(node)) {
case 'string':
if ($('#hierarchy_chk').is(':checked')) {
return_str += '' + node + '';
} else {
return_str += '' + node + '';
}
break;
case 'array':
$.each(node, function (item, value) {
return_str += JSONTREEVIEWER.processNodes(this);
});
break;
default:
/*object*/
$.each(node, function (item, value) {
if ($('#hierarchy_chk').is(':checked')) {
return_str += '' + item + '';
return_str += JSONTREEVIEWER.processNodes(this);
return_str += '';
} else {
return_str += JSONTREEVIEWER.processNodes(this);
}
});
} /*Clean up any undefined elements*/
return_str = return_str.replace('undefined', '');
return return_str;
},
Check if JSON is valid
Helper function to check if they JSON is valid and display a message if it’s invalid./*Helper function to check if JSON is valid*/
isValidJSON: function (jsonData) {
try {
jsonData = jQuery.parseJSON(jsonData);
//console.log('valid json');
return true;
} catch (e) {
//console.log('invalid json');
alert(e);
JSONTREEVIEWER.showErrorMsg();
return false;
}
},
Get Path of Node
This function searches the HTML recursively to construct the branch path of a node./*jQuery function to create path function used to get the path of the node in the tree*/
jQuery.fn.extend({
getPath: function (path) { /*The first time this function is called, path won't be defined*/
if (typeof path == 'undefined') path = ''; /*Add the element name*/
var cur = this.get(0).nodeName.toLowerCase();
var id = this.attr('id'); /*Add the #id if there is one*/
if (typeof id != 'undefined') { /*escape goat*/
if (id == 'browser') {
return path;
}
}
var html = this.html();
if (html.search(' ' + path);
} else {
return this.parent().getPath(path);
}
}
});
Events
A few functions to handle events when a user uploads a JSON file or hovers over the tree./*change event when user changes file upload input*/
$('#loadfile').live('change', function () {
JSONTREEVIEWER.processJSONTree($(this).val());
});
/*store nodepath value to clipboard (copy to top of page)*/
$('#browser li').live('click', function () {
var path = $('#pathtonode').html();
var pathdelim = $('#pathdelim_chk').val();
path = path.replace(/ > /g, pathdelim);
JSONTREEVIEWER.addtoppath(path);
});
/*mouse IN hover to show path of node*/
$('#browser li span').live('mouseenter', function () {
$('#pathtonode').html(JSONTREEVIEWER.getNodePath(this));
$('#pathtonode').show();
});
/*mouse OUT hover to show path of node*/
$('#browser li span').live('mouseleave', function () {
$('#pathtonode').empty();
$('#pathtonode').hide();
});
Requirements
I have used the jquery.treeview.async.js plugin to create the expandable tree view.Frequently Asked Questions (FAQs) about Online JSON Tree Viewer
What is an Online JSON Tree Viewer?
An Online JSON Tree Viewer is a tool that allows you to visualize the hierarchical structure of JSON data. It takes JSON data, either through direct input or from a URL, and transforms it into a tree structure. This makes it easier to understand and navigate through complex JSON data. It’s particularly useful for developers and data analysts who work with JSON data regularly.
How do I use the Online JSON Tree Viewer?
Using the Online JSON Tree Viewer is straightforward. You simply paste your JSON data into the input box and click on the ‘Visualize’ button. The tool will then generate a tree structure of your data. You can expand and collapse nodes to view different parts of your data. Some viewers also allow you to search for specific nodes, which can be very helpful when dealing with large data sets.
Can I edit my JSON data in the Tree Viewer?
Yes, some Online JSON Tree Viewers allow you to edit your JSON data directly in the tree structure. You can add, delete, or modify nodes as needed. Once you’re done editing, you can export your modified JSON data. This feature can be very useful for debugging and data manipulation.
Is my data safe when using an Online JSON Tree Viewer?
While most Online JSON Tree Viewers do not store your data, it’s always a good idea to check the privacy policy of the tool you’re using. If you’re dealing with sensitive data, consider using a local JSON Tree Viewer instead.
Can I use the Online JSON Tree Viewer with large JSON files?
Most Online JSON Tree Viewers can handle large JSON files, but performance may vary depending on the size of the file and the capabilities of the tool. If you’re dealing with very large files, consider using a local JSON Tree Viewer or splitting your data into smaller chunks.
Can I search for specific nodes in the JSON Tree Viewer?
Yes, many Online JSON Tree Viewers include a search function that allows you to find specific nodes in your data. This can be very helpful when dealing with large or complex JSON data.
Can I use the Online JSON Tree Viewer offline?
As the name suggests, an Online JSON Tree Viewer requires an internet connection to work. If you need to work with JSON data offline, consider using a local JSON Tree Viewer.
Can I visualize nested JSON data with the Online JSON Tree Viewer?
Yes, the Online JSON Tree Viewer is designed to handle nested JSON data. It will display nested data as child nodes of their parent node, allowing you to easily navigate through the hierarchy of your data.
Can I export my data from the Online JSON Tree Viewer?
Yes, most Online JSON Tree Viewers allow you to export your data, either as raw JSON or in other formats like XML or CSV. This can be very useful for data analysis and manipulation.
Can I use the Online JSON Tree Viewer on any device?
Yes, most Online JSON Tree Viewers are web-based tools that can be used on any device with a web browser, including desktop computers, laptops, tablets, and smartphones. However, performance may vary depending on the device and the size of the JSON data.
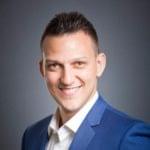
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.