This is a useful JavaScript function to create an oAuth popup window which isn’t blocked by web browsers (unless using a popup blocker) and can be monitored with a callback to authenticate through oAuth just like most popular social networks allow.
Demo
jQuery Twitter Widget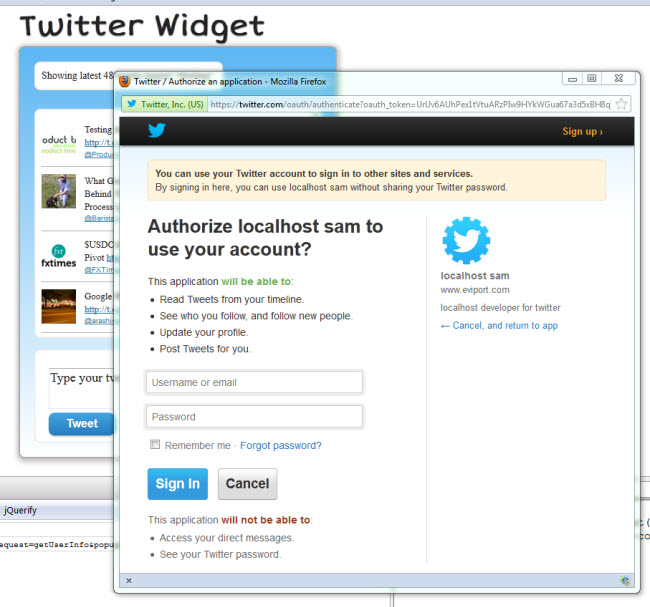
Code
//Authorization popup window code
$.oauthpopup = function(options)
{
options.windowName = options.windowName || 'ConnectWithOAuth'; // should not include space for IE
options.windowOptions = options.windowOptions || 'location=0,status=0,width=800,height=400';
options.callback = options.callback || function(){ window.location.reload(); };
var that = this;
log(options.path);
that._oauthWindow = window.open(options.path, options.windowName, options.windowOptions);
that._oauthInterval = window.setInterval(function(){
if (that._oauthWindow.closed) {
window.clearInterval(that._oauthInterval);
options.callback();
}
}, 1000);
};
Usage
//create new oAuth popup window and monitor it
$.oauthpopup({
path: urltoopen,
callback: function()
{
log('callback');
//do callback stuff
}
});
Frequently Asked Questions (FAQs) about OAuth Popup Window
What is an OAuth popup window and why is it important?
An OAuth popup window is a feature that allows users to authenticate their identity on a website without leaving the page. It’s a part of OAuth (Open Authorization), a protocol that lets users grant third-party access to their web resources without sharing their passwords. This feature is crucial for enhancing user experience, as it simplifies the login process and increases the security of user data.
How does an OAuth popup window work?
An OAuth popup window works by opening a new browser window where users can enter their login credentials. Once the user is authenticated, the popup window closes, and the user is redirected back to the original page. This process is facilitated by the OAuth protocol, which communicates with the server to verify the user’s identity.
How can I create an OAuth popup window?
Creating an OAuth popup window involves several steps. First, you need to register your application with the service provider (like Google or Facebook) to get your client ID and secret. Then, you can use these credentials to request an access token from the service provider. Once you have the access token, you can use it to authenticate the user and open the popup window.
What are the benefits of using an OAuth popup window?
Using an OAuth popup window has several benefits. It enhances user experience by simplifying the login process. It also increases security by allowing users to authenticate their identity without sharing their passwords. Additionally, it can help increase user engagement and conversion rates, as users are more likely to sign up or log in if the process is easy and secure.
Can I use an OAuth popup window with any service provider?
Yes, you can use an OAuth popup window with any service provider that supports the OAuth protocol. This includes popular services like Google, Facebook, Twitter, and LinkedIn. However, the process of setting up an OAuth popup window may vary slightly depending on the service provider.
What are the potential issues with using an OAuth popup window?
While OAuth popup windows offer many benefits, they can also have some potential issues. For example, popup windows can be blocked by browser settings or popup blockers, which can disrupt the user experience. Additionally, if not implemented correctly, OAuth can potentially expose sensitive user data.
How can I ensure the security of my OAuth popup window?
To ensure the security of your OAuth popup window, you should always use a secure connection (HTTPS) and never expose your client secret. You should also regularly update your OAuth credentials and monitor your application for any suspicious activity.
How can I customize my OAuth popup window?
You can customize your OAuth popup window by modifying the HTML and CSS of the popup window. This can include changing the size, position, and appearance of the window. However, keep in mind that any changes should not disrupt the user experience or the functionality of the OAuth process.
Can I use an OAuth popup window on mobile devices?
Yes, you can use an OAuth popup window on mobile devices. However, the process may be slightly different due to the smaller screen size and different browser settings. It’s important to ensure that your popup window is responsive and works well on all devices.
What are some best practices for using an OAuth popup window?
Some best practices for using an OAuth popup window include using a secure connection, regularly updating your OAuth credentials, and ensuring that your popup window is user-friendly and responsive. You should also monitor your application for any suspicious activity to protect user data.
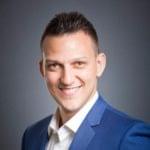
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.