Here are some code snippets and examples of how to use jQuery & HTML5 to set cursor Input Focus and Cursor Positions which are common actions with login forms and such. Comments, improvements and suggestions welcomed.
jQuery Input Focus
Simply call the focus() function to set focus to an input.//set focus on input
$('input[name=firstName]').focus();
jsfiddle.net/z9Ldt/
HTML5 Input Focus
Awesome feature provided by HTML5… Couldn’t find this on http://html5please.com/ but I’ve tested it’s working in Chrome & Firefox but not in IE9 or below.//AUTO INPUT FOCUS
<input type="text" name="myInput" autofocus />
jsfiddle.net/89PHL/
jQuery Set Cursor Position
jQuery function to set the cursor position to a specfic character position within an input field.//SET CURSOR POSITION
$.fn.setCursorPosition = function(pos) {
this.each(function(index, elem) {
if (elem.setSelectionRange) {
elem.setSelectionRange(pos, pos);
} else if (elem.createTextRange) {
var range = elem.createTextRange();
range.collapse(true);
range.moveEnd('character', pos);
range.moveStart('character', pos);
range.select();
}
});
return this;
};
Example Usage
Sets cursor position after first character.$('#username').setCursorPosition(1);
jsfiddle.net/tAZSs/
jQuery Set Cursor Position
jQuery function to auto select text (specific number of characters) within an input field.//SELECT TEXT RANGE
$.fn.selectRange = function(start, end) {
return this.each(function() {
if (this.setSelectionRange) {
this.focus();
this.setSelectionRange(start, end);
} else if (this.createTextRange) {
var range = this.createTextRange();
range.collapse(true);
range.moveEnd('character', end);
range.moveStart('character', start);
range.select();
}
});
};
Example Usage
Selects the first 5 characters in the input.$('#username').selectRange(0,5);
jsfiddle.net/yMUx5/
Frequently Asked Questions (FAQs) about jQuery/HTML5 Input Focus and Cursor Positions
How can I set the cursor position at the end of the input field using jQuery?
To set the cursor position at the end of an input field, you can use the focus
and setSelectionRange
methods in jQuery. First, you need to trigger the focus event on the input field. Then, use the setSelectionRange
method to set the cursor position. The setSelectionRange
method takes two parameters: the start and end positions of the selection. To place the cursor at the end, both parameters should be the length of the input value.var input = $('#inputField');
var len = input.val().length;
input.focus();
input[0].setSelectionRange(len, len);
How can I use jQuery to focus on an input field when a link is clicked?
You can use the click
event handler in jQuery to focus on an input field when a link is clicked. Inside the click
event handler, use the focus
method to set the focus on the desired input field. Here’s an example:$('#linkID').click(function() {
$('#inputField').focus();
});
What is the difference between the focus and focusin events in jQuery?
The focus
event is triggered when an element receives focus, either by the user selecting it or by using the keyboard navigation. It does not bubble up the DOM hierarchy. On the other hand, the focusin
event is similar to the focus
event, but it does bubble up the DOM hierarchy. This means that the focusin
event can be handled at the document level, or at any level above the element that gained focus.
How can I trigger the focus event manually in jQuery?
You can manually trigger the focus event using the focus
or trigger
methods in jQuery. Here’s how you can do it:$('#inputField').focus();
// or
$('#inputField').trigger('focus');
How can I detect when an input field loses focus in jQuery?
You can detect when an input field loses focus using the blur
event in jQuery. The blur
event is triggered when the element loses focus. Here’s an example:$('#inputField').blur(function() {
console.log('Input field has lost focus');
});
How can I prevent an input field from losing focus in jQuery?
You can prevent an input field from losing focus by using the focusout
event in conjunction with the preventDefault
method in jQuery. Here’s an example:$('#inputField').focusout(function(e) {
e.preventDefault();
});
How can I set the focus on the next input field when the enter key is pressed in jQuery?
You can set the focus on the next input field when the enter key is pressed by using the keypress
event in conjunction with the focus
method in jQuery. Here’s an example:$('input').keypress(function(e) {
if (e.which == 13) {
$(this).next('input').focus();
e.preventDefault();
}
});
How can I set the cursor position at a specific index in an input field using jQuery?
You can set the cursor position at a specific index in an input field by using the setSelectionRange
method in jQuery. Here’s an example:var input = $('#inputField');
var index = 5; // desired cursor position
input[0].setSelectionRange(index, index);
How can I get the current cursor position in an input field using jQuery?
You can get the current cursor position in an input field by using the selectionStart
property in jQuery. Here’s an example:var input = $('#inputField');
var cursorPosition = input[0].selectionStart;
How can I move the cursor to the start of an input field using jQuery?
You can move the cursor to the start of an input field by using the setSelectionRange
method in jQuery. Here’s an example:var input = $('#inputField');
input[0].setSelectionRange(0, 0);
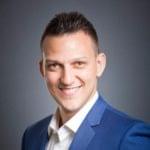
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.